svcore
1.9
|
CodedAudioFileReader.cpp
Go to the documentation of this file.
84 SVDEBUG << "CodedAudioFileReader::~CodedAudioFileReader: deleting cache file " << m_cacheFileName << endl;
86 SVDEBUG << "WARNING: CodedAudioFileReader::~CodedAudioFileReader: Failed to delete cache file \"" << m_cacheFileName << "\"" << endl;
120 // SVCERR << "CodedAudioFileReader(" << this << ")::endSerialised: id = " << (m_serialiser ? m_serialiser->getId() : "(none)") << endl;
139 SVDEBUG << "CodedAudioFileReader::initialiseDecodeCache: ERROR: File sample rate unknown (bug in subclass implementation?)" << endl;
140 throw FileOperationFailed("(coded file)", "sample rate unknown (bug in subclass implementation?)");
144 SVDEBUG << "CodedAudioFileReader::initialiseDecodeCache: rate (from file) = " << m_fileRate << endl;
220 SVDEBUG << "ERROR: CodedAudioFileReader::initialiseDecodeCache: Failed to construct WAV file reader for temporary file: " << m_cacheFileReader->getError() << endl;
228 SVDEBUG << "CodedAudioFileReader::initialiseDecodeCache: failed to open cache file \"" << m_cacheFileName << "\" (" << m_channelCount << " channels, sample rate " << m_sampleRate << " for writing, falling back to in-memory cache" << endl;
233 SVDEBUG << "CodedAudioFileReader::initialiseDecodeCache: failed to create temporary directory! Falling back to in-memory cache" << endl;
243 SVCERR << "WARNING: CodedAudioFileReader::setSamplesToTrim: Can't handle trimming more frames from end (" << m_trimFromEnd << ") than can be stored in cache-write buffer (" << (m_cacheWriteBufferFrames * m_channelCount) << "), won't trim anything from the end after all";
328 SVDEBUG << "WARNING: CodedAudioFileReader::finishDecodeCache: Cache was never initialised!" << endl;
486 SVCERR << "CodedAudioFileReader: Caught bad_alloc when trying to add " << count << " elements to buffer" << endl;
499 // SVDEBUG << "pushBufferResampling: ratio = " << ratio << ", sz = " << sz << ", final = " << final << endl;
523 SVDEBUG << "CodedAudioFileReader::pushBufferResampling: frameCount = " << m_frameCount << ", equivFileFrames = " << double(m_frameCount) / ratio << ", m_fileFrameCount = " << m_fileFrameCount << ", padFrames = " << padFrames << ", padSamples = " << padSamples << endl;
536 SVDEBUG << "CodedAudioFileReader::pushBufferResampling: resampled padFrames to " << out << " frames" << endl;
541 SVDEBUG << "CodedAudioFileReader::pushBufferResampling: clipping that to " << out << " to avoid producing more samples than desired" << endl;
sv_frame_t pushBuffer(float *interleaved, sv_frame_t sz, bool final)
Definition: CodedAudioFileReader.cpp:414
Definition: Exceptions.h:81
sv_frame_t m_trimFromStart
Definition: CodedAudioFileReader.h:128
sv_frame_t m_cacheWriteBufferIndex
Definition: CodedAudioFileReader.h:116
float * m_cacheWriteBuffer
Definition: CodedAudioFileReader.h:115
int m_resampleBufferFrames
Definition: CodedAudioFileReader.h:121
void pushBufferResampling(float *interleaved, sv_frame_t sz, double ratio, bool final)
Definition: CodedAudioFileReader.cpp:496
SNDFILE * m_cacheFileWritePtr
Definition: CodedAudioFileReader.h:113
float * m_resampleBuffer
Definition: CodedAudioFileReader.h:120
floatvec_t getInterleavedFrames(sv_frame_t start, sv_frame_t count) const override
Return interleaved samples for count frames from index start.
Definition: CodedAudioFileReader.cpp:550
void startSerialised(QString id, const std::atomic< bool > *cancelled)
Definition: CodedAudioFileReader.cpp:108
sv_frame_t m_fileFrameCount
Definition: CodedAudioFileReader.h:122
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
virtual ~CodedAudioFileReader()
Definition: CodedAudioFileReader.cpp:70
static void notifyPlannedAllocation(AllocationArea area, size_t size)
Specify that we are planning to use a given amount of storage (in kilobytes), but haven't allocated i...
Definition: StorageAdviser.cpp:248
Definition: Exceptions.h:69
void addSamplesToDecodeCache(float **samples, sv_frame_t nframes)
Definition: CodedAudioFileReader.cpp:251
QString getError() const override
If isOK() is false, return an error string.
Definition: WavFileReader.h:52
CodedAudioFileReader(CacheMode cacheMode, sv_samplerate_t targetRate, bool normalised)
Definition: CodedAudioFileReader.cpp:34
WavFileReader * m_cacheFileReader
Definition: CodedAudioFileReader.h:114
static void notifyDoneAllocation(AllocationArea area, size_t size)
Specify that we have now allocated, or abandoned the allocation of, the given amount (in kilobytes) o...
Definition: StorageAdviser.cpp:257
void pushBufferNonResampling(float *interleaved, sv_frame_t sz)
Definition: CodedAudioFileReader.cpp:433
bool isOK() const
Return true if the file was opened successfully and no error has subsequently occurred.
Definition: AudioFileReader.h:38
sv_frame_t m_cacheWriteBufferFrames
Definition: CodedAudioFileReader.h:117
void pushCacheWriteBufferMaybe(bool final)
Definition: CodedAudioFileReader.cpp:372
breakfastquay::Resampler * m_resampler
Definition: CodedAudioFileReader.h:119
void finishDecodeCache()
Definition: CodedAudioFileReader.cpp:321
void setFramesToTrim(sv_frame_t fromStart, sv_frame_t fromEnd)
Definition: CodedAudioFileReader.cpp:101
void initialiseDecodeCache()
Definition: CodedAudioFileReader.cpp:127
Definition: Exceptions.h:47
floatvec_t getInterleavedFrames(sv_frame_t start, sv_frame_t count) const override
Must be safe to call from multiple threads with different arguments on the same object at the same ti...
Definition: WavFileReader.cpp:164
Definition: Serialiser.h:25
Generated by
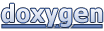