svcore
1.9
|
StorageAdviser.h
Go to the documentation of this file.
Definition: StorageAdviser.h:35
A utility class designed to help decide whether to store cache data (for example FFT outputs) in memo...
Definition: StorageAdviser.h:30
Definition: StorageAdviser.h:36
Definition: StorageAdviser.h:98
Definition: StorageAdviser.h:99
Definition: StorageAdviser.h:45
static Recommendation recommend(Criteria criteria, size_t minimumSize, size_t maximumSize)
Recommend where to store some data, given certain storage and recall criteria.
Definition: StorageAdviser.cpp:67
Definition: StorageAdviser.h:47
Definition: StorageAdviser.h:48
static QString criteriaToString(int)
Definition: StorageAdviser.cpp:26
static Recommendation m_baseRecommendation
Definition: StorageAdviser.h:95
Definition: StorageAdviser.h:100
static void notifyPlannedAllocation(AllocationArea area, size_t size)
Specify that we are planning to use a given amount of storage (in kilobytes), but haven't allocated i...
Definition: StorageAdviser.cpp:248
Definition: StorageAdviser.h:46
static void notifyDoneAllocation(AllocationArea area, size_t size)
Specify that we have now allocated, or abandoned the allocation of, the given amount (in kilobytes) o...
Definition: StorageAdviser.cpp:257
Definition: StorageAdviser.h:49
Definition: StorageAdviser.h:101
static void setFixedRecommendation(Recommendation recommendation)
Force all subsequent recommendations to use the (perhaps partial) specification given here...
Definition: StorageAdviser.cpp:271
static QString recommendationToString(int)
Definition: StorageAdviser.cpp:38
static QString storageStatusToString(StorageStatus)
Definition: StorageAdviser.cpp:52
Generated by
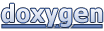