svcore
1.9
|
A utility class designed to help decide whether to store cache data (for example FFT outputs) in memory or on disk in the TempDirectory. More...
#include <StorageAdviser.h>
Public Types | |
enum | Criteria { NoCriteria = 0, SpeedCritical = 1, PrecisionCritical = 2, LongRetentionLikely = 4, FrequentLookupLikely = 8 } |
enum | Recommendation { NoRecommendation = 0, UseMemory = 1, PreferMemory = 2, PreferDisc = 4, UseDisc = 8, ConserveSpace = 16, UseAsMuchAsYouLike = 32 } |
enum | AllocationArea { MemoryAllocation, DiscAllocation } |
Static Public Member Functions | |
static Recommendation | recommend (Criteria criteria, size_t minimumSize, size_t maximumSize) |
Recommend where to store some data, given certain storage and recall criteria. More... | |
static void | notifyPlannedAllocation (AllocationArea area, size_t size) |
Specify that we are planning to use a given amount of storage (in kilobytes), but haven't allocated it yet. More... | |
static void | notifyDoneAllocation (AllocationArea area, size_t size) |
Specify that we have now allocated, or abandoned the allocation of, the given amount (in kilobytes) of a storage area that was previously notified using notifyPlannedAllocation. More... | |
static void | setFixedRecommendation (Recommendation recommendation) |
Force all subsequent recommendations to use the (perhaps partial) specification given here. More... | |
Private Types | |
enum | StorageStatus { Unknown, Insufficient, Marginal, Sufficient } |
Static Private Member Functions | |
static QString | criteriaToString (int) |
static QString | recommendationToString (int) |
static QString | storageStatusToString (StorageStatus) |
Static Private Attributes | |
static size_t | m_discPlanned = 0 |
static size_t | m_memoryPlanned = 0 |
static Recommendation | m_baseRecommendation = StorageAdviser::NoRecommendation |
Detailed Description
A utility class designed to help decide whether to store cache data (for example FFT outputs) in memory or on disk in the TempDirectory.
This is basically a compendium of simple rules of thumb.
Definition at line 30 of file StorageAdviser.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NoCriteria | |
SpeedCritical | |
PrecisionCritical | |
LongRetentionLikely | |
FrequentLookupLikely |
Definition at line 34 of file StorageAdviser.h.
Enumerator | |
---|---|
NoRecommendation | |
UseMemory | |
PreferMemory | |
PreferDisc | |
UseDisc | |
ConserveSpace | |
UseAsMuchAsYouLike |
Definition at line 43 of file StorageAdviser.h.
Enumerator | |
---|---|
MemoryAllocation | |
DiscAllocation |
Definition at line 67 of file StorageAdviser.h.
|
private |
Enumerator | |
---|---|
Unknown | |
Insufficient | |
Marginal | |
Sufficient |
Definition at line 97 of file StorageAdviser.h.
Member Function Documentation
|
static |
Recommend where to store some data, given certain storage and recall criteria.
The minimum size is the approximate amount of data in kilobytes that will be stored if the recommendation is to ConserveSpace; the maximum size is approximately the amount that will be used if UseAsMuchAsYouLike is returned.
May throw InsufficientDiscSpace exception if there appears to be nowhere the minimum amount of data can be stored.
!! We have a potentially serious problem here if multiple
Definition at line 67 of file StorageAdviser.cpp.
References ConserveSpace, criteriaToString(), FrequentLookupLikely, GetDiscSpaceMBAvailable(), TempDirectory::getInstance(), TempDirectory::getPath(), GetRealMemoryMBAvailable(), Insufficient, LongRetentionLikely, m_baseRecommendation, m_discPlanned, m_memoryPlanned, Marginal, NoRecommendation, PrecisionCritical, PreferDisc, PreferMemory, recommendationToString(), SpeedCritical, storageStatusToString(), Sufficient, SVDEBUG, Unknown, UseAsMuchAsYouLike, UseDisc, and UseMemory.
Referenced by AudioFileReaderFactory::createReader().
|
static |
Specify that we are planning to use a given amount of storage (in kilobytes), but haven't allocated it yet.
Definition at line 248 of file StorageAdviser.cpp.
References DiscAllocation, m_discPlanned, m_memoryPlanned, MemoryAllocation, and SVDEBUG.
Referenced by CodedAudioFileReader::finishDecodeCache().
|
static |
Specify that we have now allocated, or abandoned the allocation of, the given amount (in kilobytes) of a storage area that was previously notified using notifyPlannedAllocation.
Definition at line 257 of file StorageAdviser.cpp.
References DiscAllocation, m_discPlanned, m_memoryPlanned, MemoryAllocation, and SVDEBUG.
Referenced by CodedAudioFileReader::~CodedAudioFileReader().
|
static |
Force all subsequent recommendations to use the (perhaps partial) specification given here.
If NoRecommendation given here, this will reset to the default free behaviour.
Definition at line 271 of file StorageAdviser.cpp.
References m_baseRecommendation.
|
staticprivate |
Definition at line 26 of file StorageAdviser.cpp.
References FrequentLookupLikely, LongRetentionLikely, PrecisionCritical, and SpeedCritical.
Referenced by recommend().
|
staticprivate |
Definition at line 38 of file StorageAdviser.cpp.
References ConserveSpace, PreferDisc, PreferMemory, UseAsMuchAsYouLike, UseDisc, and UseMemory.
Referenced by recommend().
|
staticprivate |
Definition at line 52 of file StorageAdviser.cpp.
References Insufficient, m_baseRecommendation, m_discPlanned, m_memoryPlanned, Marginal, NoRecommendation, and Sufficient.
Referenced by recommend().
Member Data Documentation
|
staticprivate |
Definition at line 93 of file StorageAdviser.h.
Referenced by notifyDoneAllocation(), notifyPlannedAllocation(), recommend(), and storageStatusToString().
|
staticprivate |
Definition at line 94 of file StorageAdviser.h.
Referenced by notifyDoneAllocation(), notifyPlannedAllocation(), recommend(), and storageStatusToString().
|
staticprivate |
Definition at line 95 of file StorageAdviser.h.
Referenced by recommend(), setFixedRecommendation(), and storageStatusToString().
The documentation for this class was generated from the following files:
Generated by
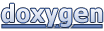