svcore
1.9
|
CodedAudioFileReader.h
Go to the documentation of this file.
49 CacheInMemory
sv_frame_t m_trimFromStart
Definition: CodedAudioFileReader.h:128
bool isQuicklySeekable() const override
Intermediate cache means all CodedAudioFileReaders are quickly seekable.
Definition: CodedAudioFileReader.h:64
sv_frame_t m_cacheWriteBufferIndex
Definition: CodedAudioFileReader.h:116
float * m_cacheWriteBuffer
Definition: CodedAudioFileReader.h:115
int m_resampleBufferFrames
Definition: CodedAudioFileReader.h:121
Definition: AudioFileReader.h:27
Definition: CodedAudioFileReader.h:40
SNDFILE * m_cacheFileWritePtr
Definition: CodedAudioFileReader.h:113
float * m_resampleBuffer
Definition: CodedAudioFileReader.h:120
sv_frame_t m_fileFrameCount
Definition: CodedAudioFileReader.h:122
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
QString getLocalFilename() const override
Return the local file path of the audio data.
Definition: CodedAudioFileReader.h:61
sv_samplerate_t getNativeRate() const override
Return the native samplerate of the file.
Definition: CodedAudioFileReader.h:59
WavFileReader * m_cacheFileReader
Definition: CodedAudioFileReader.h:114
sv_frame_t m_cacheWriteBufferFrames
Definition: CodedAudioFileReader.h:117
breakfastquay::Resampler * m_resampler
Definition: CodedAudioFileReader.h:119
Definition: CodedAudioFileReader.h:36
Definition: Serialiser.h:25
bool isDecodeCacheInitialised() const
Definition: CodedAudioFileReader.h:87
Generated by
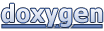