svcore
1.9
|
RealTimeEffectModelTransformer.cpp
Go to the documentation of this file.
50 SVDEBUG << "RealTimeEffectModelTransformer::RealTimeEffectModelTransformer: plugin " << pluginId << ", output " << transform.getOutput() << endl;
82 cerr << "RealTimeEffectModelTransformer: Plugin has fewer than desired " << m_outputNo << " control outputs" << endl;
132 SVDEBUG << "RealTimeEffectModelTransformer::run: Waiting for input model to be ready..." << endl;
void abandon()
Hint to the processing thread that it should give up, for example because the process is going to exi...
Definition: ModelTransformer.h:66
void setPluginParameters(const Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin)
Set the parameters, program and configuration strings on the given plugin from the given Transform ob...
Definition: TransformFactory.cpp:1003
Definition: RealTimePluginFactory.h:50
static RealTime frame2RealTime(sv_frame_t frame, sv_samplerate_t sampleRate)
Convert a sample frame at the given sample rate into a RealTime.
Definition: RealTimeSV.cpp:498
int m_outputNo
Definition: RealTimeEffectModelTransformer.h:38
Definition: ModelTransformer.h:44
static RealTimePluginFactory * instanceFor(QString identifier)
Definition: RealTimePluginFactory.cpp:65
void run() override
Definition: RealTimeEffectModelTransformer.cpp:114
std::shared_ptr< RealTimePluginInstance > m_plugin
Definition: RealTimeEffectModelTransformer.h:37
static TransformFactory * getInstance()
Definition: TransformFactory.cpp:48
virtual std::shared_ptr< RealTimePluginInstance > instantiatePlugin(QString identifier, int clientId, int position, sv_samplerate_t sampleRate, int blockSize, int channels)=0
Instantiate a plugin.
QString m_units
Definition: RealTimeEffectModelTransformer.h:36
virtual ~RealTimeEffectModelTransformer()
Definition: RealTimeEffectModelTransformer.cpp:109
static sv_frame_t realTime2Frame(const RealTime &r, sv_samplerate_t sampleRate)
Convert a RealTime into a sample frame at the given sample rate.
Definition: RealTimeSV.cpp:490
Definition: Transform.h:34
An immutable(-ish) type used for point and event representation in sparse models, as well as for inte...
Definition: Event.h:55
A ModelTransformer turns one data model into another.
Definition: ModelTransformer.h:37
QString getTransformUnits(TransformId identifier)
Definition: TransformFactory.cpp:871
RealTimeEffectModelTransformer(Input input, const Transform &transform)
Definition: RealTimeEffectModelTransformer.cpp:32
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
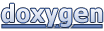