svcore
1.9
|
TransformFactory.cpp
Go to the documentation of this file.
119 cerr << "inserting transform into set: id = " << i->second.identifier << " (" << i->second.name << ")" << endl;
127 cerr << "inserting transform into list: id = " << i->identifier << " (" << i->name << ")" << endl;
436 cerr << "WARNING: TransformFactory::populateTransforms: No plugin static data available for instance " << pluginId << endl;
491 cerr << "Feature extraction plugin transform: " << transformId << " friendly name: " << friendlyName << endl;
527 cerr << "WARNING: TransformFactory::populateTransforms: No real time plugin factory for instance " << pluginId << endl;
535 cerr << "WARNING: TransformFactory::populateTransforms: Failed to query plugin " << pluginId << endl;
542 // cout << "TransformFactory::populateRealTimePlugins: plugin " << pluginId << " has " << descriptor.controlOutputPortCount << " control output ports, " << descriptor.audioOutputPortCount << " audio outputs, " << descriptor.audioInputPortCount << " audio inputs" << endl;
1197 SVDEBUG << "WARNING: TransformFactory::search: Uninstalled transforms are not populated yet" << endl
TransformInstallStatus getTransformInstallStatus(TransformId id)
Definition: TransformFactory.cpp:213
Definition: Thread.h:43
Definition: TransformFactory.h:34
Transform getDefaultTransformFor(TransformId identifier, sv_samplerate_t rate=0)
A single transform ID can lead to many possible Transforms, with different parameters and execution c...
Definition: TransformFactory.cpp:766
std::vector< QString > getTransformCategories(TransformDescription::Type)
Definition: TransformFactory.cpp:263
QString getTransformTypeName(TransformDescription::Type) const
Definition: TransformFactory.cpp:317
std::vector< std::string > controlOutputPortNames
Definition: RealTimePluginFactory.h:47
void setParametersFromPlugin(Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin)
Set the plugin parameters, program and configuration strings on the given Transform object from the g...
Definition: TransformFactory.cpp:955
TransformDescriptionMap m_transforms
Definition: TransformFactory.h:209
TransformList getUninstalledTransformDescriptions()
Definition: TransformFactory.cpp:155
void transformsPopulated()
virtual void setParametersFromXml(QString xml)
Set the parameters and program of a plugin from an XML plugin element as returned by toXml...
Definition: PluginXml.cpp:195
void setPluginParameters(const Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin)
Set the parameters, program and configuration strings on the given plugin from the given Transform ob...
Definition: TransformFactory.cpp:1003
QMutex m_uninstalledTransformsMutex
Definition: TransformFactory.h:224
std::map< TransformId, TransformDescription > TransformDescriptionMap
Definition: TransformFactory.h:207
TransformDescription getTransformDescription(TransformId id)
Definition: TransformFactory.cpp:136
void run() override
Definition: TransformFactory.cpp:102
Vamp::Plugin::InputDomain getTransformInputDomain(TransformId identifier)
Definition: TransformFactory.cpp:887
Definition: RealTimePluginFactory.h:50
Provider getPluginProvider() const
Definition: PluginRDFDescription.cpp:85
virtual std::vector< QString > getPluginIdentifiers(QString &errorMsg)=0
Return all installed plugin identifiers.
virtual RealTimePluginDescriptor getPluginDescriptor(QString identifier) const =0
Get some basic information about a plugin (rapidly).
bool haveTransform(TransformId identifier)
Return true if the given transform is known.
Definition: TransformFactory.cpp:848
bool haveInstalledTransforms()
Definition: TransformFactory.cpp:148
static RealTimePluginFactory * instanceFor(QString identifier)
Definition: RealTimePluginFactory.cpp:65
QString getOutputName(QString outputId) const
Definition: PluginRDFDescription.cpp:102
Definition: PluginRDFDescription.h:28
QStringList getOutputIds() const
Definition: PluginRDFDescription.cpp:91
void uninstalledTransformsPopulated()
UninstalledTransformsPopulateThread * m_thread
Definition: TransformFactory.h:236
std::shared_ptr< Vamp::PluginBase > instantiatePluginFor(const Transform &transform)
Load an appropriate plugin for the given transform and set the parameters, program and configuration ...
Definition: TransformFactory.cpp:787
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Export plugin settings to XML.
Definition: PluginXml.cpp:62
std::map< std::string, std::string > ConfigurationPairMap
Definition: RealTimePluginInstance.h:139
unsigned int audioInputPortCount
Definition: RealTimePluginFactory.h:44
bool m_uninstalledTransformsPopulated
Definition: TransformFactory.h:213
const ConfigurationMap & getConfiguration() const
Definition: Transform.cpp:263
unsigned int parameterCount
Definition: RealTimePluginFactory.h:43
void test(Match &match, QStringList keywords, QString text, QString textType, int score)
Definition: TextMatcher.cpp:27
static TransformId getIdentifierForPluginOutput(QString pluginIdentifier, QString output="")
Definition: Transform.cpp:237
static std::vector< QString > getAllPluginIdentifiers()
Definition: RealTimePluginFactory.cpp:73
unsigned int controlOutputPortCount
Definition: RealTimePluginFactory.h:46
virtual QString getPluginCategory(QString identifier)=0
Get category metadata about a plugin (without instantiating it).
QString getTransformName(TransformId identifier)
Full name of a transform, suitable for putting on a menu.
Definition: TransformFactory.cpp:855
QString getTransformFriendlyName(TransformId identifier)
Brief but friendly name of a transform, suitable for use as the name of the output layer...
Definition: TransformFactory.cpp:863
static TransformFactory * getInstance()
Definition: TransformFactory.cpp:48
bool getTransformChannelRange(TransformId identifier, int &minChannels, int &maxChannels)
If the transform has a prescribed number or range of channel inputs, return true and set minChannels ...
Definition: TransformFactory.cpp:920
TransformList getAllTransformDescriptions()
Definition: TransformFactory.cpp:112
A rather eccentric interface for matching texts in differently-scored fields.
Definition: TextMatcher.h:27
virtual std::shared_ptr< RealTimePluginInstance > instantiatePlugin(QString identifier, int clientId, int position, sv_samplerate_t sampleRate, int blockSize, int channels)=0
Instantiate a plugin.
QString getPluginDescription() const
Definition: PluginRDFDescription.cpp:73
void populateUninstalledTransforms()
Definition: TransformFactory.cpp:646
void populateFeatureExtractionPlugins(TransformDescriptionMap &)
Definition: TransformFactory.cpp:416
QString getPluginConfigurationXml(const Transform &transform)
Retrieve a <plugin ...
Definition: TransformFactory.cpp:1079
std::shared_ptr< Vamp::PluginBase > instantiateDefaultPluginFor(TransformId id, sv_samplerate_t rate)
Definition: TransformFactory.cpp:803
bool isTransformConfigurable(TransformId identifier)
Return true if the transform has any configurable parameters, i.e.
Definition: TransformFactory.cpp:912
QStringList getIndexedPluginIds()
Definition: PluginRDFIndexer.cpp:253
bool havePopulated()
Return true if the transforms have been populated, i.e.
Definition: TransformFactory.cpp:330
Provider getTransformProvider(TransformId identifier)
Definition: TransformFactory.cpp:879
void setConfiguration(const ConfigurationMap &cm)
Definition: Transform.cpp:269
QString getPluginMaker() const
Definition: PluginRDFDescription.cpp:79
Definition: Transform.h:71
std::vector< TransformDescription::Type > getAllTransformTypes()
Definition: TransformFactory.cpp:245
virtual std::shared_ptr< Vamp::Plugin > instantiatePlugin(QString identifier, sv_samplerate_t inputSampleRate)=0
Instantiate (load) and return pointer to the plugin with the given identifier, at the given sample ra...
std::vector< QString > getTransformMakers(TransformDescription::Type)
Definition: TransformFactory.cpp:290
Definition: Transform.h:34
static FeatureExtractionPluginFactory * instance()
Definition: FeatureExtractionPluginFactory.cpp:26
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
void performConsistencyChecks()
Perform various checks for consistency of RDF definitions, printing warnings to stderr/logfile as app...
Definition: PluginRDFIndexer.cpp:189
QString getPluginName() const
Definition: PluginRDFDescription.cpp:67
void setParametersFromPluginConfigurationXml(Transform &transform, QString xml)
Set the plugin parameters, program and configuration strings on the given Transform object from the g...
Definition: TransformFactory.cpp:1103
bool haveUninstalledTransforms(bool waitForCheckToComplete=false)
Definition: TransformFactory.cpp:194
std::map< TransformId, TextMatcher::Match > SearchResults
Definition: TransformFactory.h:78
static bool compareUserStrings(QString s1, QString s2)
Definition: TransformDescription.h:85
QString getTransformUnits(TransformId identifier)
Definition: TransformFactory.cpp:871
Definition: Transform.h:71
virtual ConfigurationPairMap getConfigurePairs()
Definition: RealTimePluginInstance.h:140
Definition: TextMatcher.h:33
virtual QString getPluginCategory(QString identifier)=0
Get category metadata about a plugin (without instantiating it).
void populateRealTimePlugins(TransformDescriptionMap &)
Definition: TransformFactory.cpp:511
bool indexConfiguredURLs()
Index all URLs obtained from index files defined in the current settings.
Definition: PluginRDFIndexer.cpp:141
static PluginRDFIndexer * getInstance()
Definition: PluginRDFIndexer.cpp:57
virtual piper_vamp::PluginStaticData getPluginStaticData(QString ident)=0
Return static data for the given plugin.
Definition: Provider.h:22
void makeContextConsistentWithPlugin(Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin)
If the given Transform object has no processing step and block sizes set, set them to appropriate def...
Definition: TransformFactory.cpp:1041
void startPopulationThread()
TransformFactory has a background thread that can populate uninstalled transforms from network RDF re...
Definition: TransformFactory.cpp:86
SearchResults searchUnadjusted(QStringList keywords)
Definition: TransformFactory.cpp:1162
TransformDescription getUninstalledTransformDescription(TransformId id)
Definition: TransformFactory.cpp:181
Definition: PluginXml.h:26
TransformDescriptionMap m_uninstalledTransforms
Definition: TransformFactory.h:212
unsigned int audioOutputPortCount
Definition: RealTimePluginFactory.h:45
Generated by
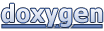