svcore
1.9
|
TransformFactory.h
Go to the documentation of this file.
173 void makeContextConsistentWithPlugin(Transform &transform, std::shared_ptr<Vamp::PluginBase> plugin);
222 std::shared_ptr<Vamp::PluginBase> instantiateDefaultPluginFor(TransformId id, sv_samplerate_t rate);
TransformInstallStatus getTransformInstallStatus(TransformId id)
Definition: TransformFactory.cpp:213
Definition: TransformFactory.h:34
Transform getDefaultTransformFor(TransformId identifier, sv_samplerate_t rate=0)
A single transform ID can lead to many possible Transforms, with different parameters and execution c...
Definition: TransformFactory.cpp:766
std::vector< QString > getTransformCategories(TransformDescription::Type)
Definition: TransformFactory.cpp:263
QString getTransformTypeName(TransformDescription::Type) const
Definition: TransformFactory.cpp:317
void setParametersFromPlugin(Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin)
Set the plugin parameters, program and configuration strings on the given Transform object from the g...
Definition: TransformFactory.cpp:955
TransformDescriptionMap m_transforms
Definition: TransformFactory.h:209
TransformList getUninstalledTransformDescriptions()
Definition: TransformFactory.cpp:155
void transformsPopulated()
void setPluginParameters(const Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin)
Set the parameters, program and configuration strings on the given plugin from the given Transform ob...
Definition: TransformFactory.cpp:1003
QMutex m_uninstalledTransformsMutex
Definition: TransformFactory.h:224
std::map< TransformId, TransformDescription > TransformDescriptionMap
Definition: TransformFactory.h:207
TransformDescription getTransformDescription(TransformId id)
Definition: TransformFactory.cpp:136
void run() override
Definition: TransformFactory.cpp:102
Vamp::Plugin::InputDomain getTransformInputDomain(TransformId identifier)
Definition: TransformFactory.cpp:887
TransformFactory * m_factory
Definition: TransformFactory.h:233
bool haveTransform(TransformId identifier)
Return true if the given transform is known.
Definition: TransformFactory.cpp:848
bool haveInstalledTransforms()
Definition: TransformFactory.cpp:148
void uninstalledTransformsPopulated()
UninstalledTransformsPopulateThread * m_thread
Definition: TransformFactory.h:236
std::shared_ptr< Vamp::PluginBase > instantiatePluginFor(const Transform &transform)
Load an appropriate plugin for the given transform and set the parameters, program and configuration ...
Definition: TransformFactory.cpp:787
bool m_uninstalledTransformsPopulated
Definition: TransformFactory.h:213
UninstalledTransformsPopulateThread(TransformFactory *factory)
Definition: TransformFactory.h:229
QString getTransformName(TransformId identifier)
Full name of a transform, suitable for putting on a menu.
Definition: TransformFactory.cpp:855
QString getTransformFriendlyName(TransformId identifier)
Brief but friendly name of a transform, suitable for use as the name of the output layer...
Definition: TransformFactory.cpp:863
static TransformFactory * getInstance()
Definition: TransformFactory.cpp:48
bool getTransformChannelRange(TransformId identifier, int &minChannels, int &maxChannels)
If the transform has a prescribed number or range of channel inputs, return true and set minChannels ...
Definition: TransformFactory.cpp:920
TransformList getAllTransformDescriptions()
Definition: TransformFactory.cpp:112
void populateUninstalledTransforms()
Definition: TransformFactory.cpp:646
void populateFeatureExtractionPlugins(TransformDescriptionMap &)
Definition: TransformFactory.cpp:416
QString getPluginConfigurationXml(const Transform &transform)
Retrieve a <plugin ...
Definition: TransformFactory.cpp:1079
std::shared_ptr< Vamp::PluginBase > instantiateDefaultPluginFor(TransformId id, sv_samplerate_t rate)
Definition: TransformFactory.cpp:803
bool isTransformConfigurable(TransformId identifier)
Return true if the transform has any configurable parameters, i.e.
Definition: TransformFactory.cpp:912
bool havePopulated()
Return true if the transforms have been populated, i.e.
Definition: TransformFactory.cpp:330
Provider getTransformProvider(TransformId identifier)
Definition: TransformFactory.cpp:879
std::vector< TransformDescription::Type > getAllTransformTypes()
Definition: TransformFactory.cpp:245
std::vector< QString > getTransformMakers(TransformDescription::Type)
Definition: TransformFactory.cpp:290
Definition: Transform.h:34
QString getStartupFailureReport() const
Definition: TransformFactory.h:198
void setParametersFromPluginConfigurationXml(Transform &transform, QString xml)
Set the plugin parameters, program and configuration strings on the given Transform object from the g...
Definition: TransformFactory.cpp:1103
bool haveUninstalledTransforms(bool waitForCheckToComplete=false)
Definition: TransformFactory.cpp:194
std::map< TransformId, TextMatcher::Match > SearchResults
Definition: TransformFactory.h:78
QString getTransformUnits(TransformId identifier)
Definition: TransformFactory.cpp:871
void populateRealTimePlugins(TransformDescriptionMap &)
Definition: TransformFactory.cpp:511
Definition: Provider.h:22
void makeContextConsistentWithPlugin(Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin)
If the given Transform object has no processing step and block sizes set, set them to appropriate def...
Definition: TransformFactory.cpp:1041
void startPopulationThread()
TransformFactory has a background thread that can populate uninstalled transforms from network RDF re...
Definition: TransformFactory.cpp:86
SearchResults searchUnadjusted(QStringList keywords)
Definition: TransformFactory.cpp:1162
TransformDescription getUninstalledTransformDescription(TransformId id)
Definition: TransformFactory.cpp:181
TransformDescriptionMap m_uninstalledTransforms
Definition: TransformFactory.h:212
Generated by
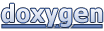