svcore
1.9
|
Provider.h
Go to the documentation of this file.
Definition: Provider.h:29
Definition: Provider.h:32
Definition: Provider.h:31
Definition: Provider.h:33
Definition: Provider.h:30
Definition: Provider.h:28
Definition: Provider.h:22
bool hasDownloadForThisPlatform() const
Definition: Provider.h:43
Generated by
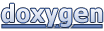