svcore
1.9
|
#include <TransformFactory.h>
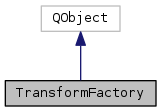
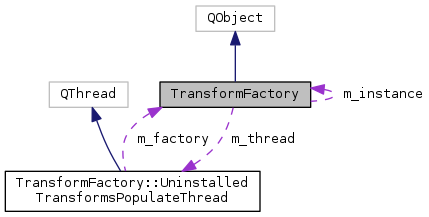
Classes | |
class | UninstalledTransformsPopulateThread |
Public Types | |
enum | TransformInstallStatus { TransformUnknown, TransformInstalled, TransformNotInstalled } |
typedef std::map< TransformId, TextMatcher::Match > | SearchResults |
Signals | |
void | transformsPopulated () |
void | uninstalledTransformsPopulated () |
Public Member Functions | |
TransformFactory () | |
virtual | ~TransformFactory () |
void | startPopulationThread () |
TransformFactory has a background thread that can populate uninstalled transforms from network RDF resources. More... | |
TransformList | getAllTransformDescriptions () |
TransformDescription | getTransformDescription (TransformId id) |
bool | haveInstalledTransforms () |
TransformList | getUninstalledTransformDescriptions () |
TransformDescription | getUninstalledTransformDescription (TransformId id) |
bool | haveUninstalledTransforms (bool waitForCheckToComplete=false) |
TransformInstallStatus | getTransformInstallStatus (TransformId id) |
std::vector< TransformDescription::Type > | getAllTransformTypes () |
std::vector< QString > | getTransformCategories (TransformDescription::Type) |
std::vector< QString > | getTransformMakers (TransformDescription::Type) |
QString | getTransformTypeName (TransformDescription::Type) const |
SearchResults | search (QString keyword) |
SearchResults | search (QStringList keywords) |
bool | havePopulated () |
Return true if the transforms have been populated, i.e. More... | |
bool | haveTransform (TransformId identifier) |
Return true if the given transform is known. More... | |
Transform | getDefaultTransformFor (TransformId identifier, sv_samplerate_t rate=0) |
A single transform ID can lead to many possible Transforms, with different parameters and execution context settings. More... | |
QString | getTransformName (TransformId identifier) |
Full name of a transform, suitable for putting on a menu. More... | |
QString | getTransformFriendlyName (TransformId identifier) |
Brief but friendly name of a transform, suitable for use as the name of the output layer. More... | |
QString | getTransformUnits (TransformId identifier) |
Provider | getTransformProvider (TransformId identifier) |
Vamp::Plugin::InputDomain | getTransformInputDomain (TransformId identifier) |
bool | isTransformConfigurable (TransformId identifier) |
Return true if the transform has any configurable parameters, i.e. More... | |
bool | getTransformChannelRange (TransformId identifier, int &minChannels, int &maxChannels) |
If the transform has a prescribed number or range of channel inputs, return true and set minChannels and maxChannels to the minimum and maximum number of channel inputs the transform can accept. More... | |
std::shared_ptr< Vamp::PluginBase > | instantiatePluginFor (const Transform &transform) |
Load an appropriate plugin for the given transform and set the parameters, program and configuration strings on that plugin from the Transform object. More... | |
void | setParametersFromPlugin (Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin) |
Set the plugin parameters, program and configuration strings on the given Transform object from the given plugin instance. More... | |
void | setPluginParameters (const Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin) |
Set the parameters, program and configuration strings on the given plugin from the given Transform object. More... | |
void | makeContextConsistentWithPlugin (Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin) |
If the given Transform object has no processing step and block sizes set, set them to appropriate defaults for the given plugin. More... | |
QString | getPluginConfigurationXml (const Transform &transform) |
Retrieve a <plugin ... More... | |
void | setParametersFromPluginConfigurationXml (Transform &transform, QString xml) |
Set the plugin parameters, program and configuration strings on the given Transform object from the given <plugin ... More... | |
QString | getStartupFailureReport () const |
Static Public Member Functions | |
static TransformFactory * | getInstance () |
static void | deleteInstance () |
Protected Types | |
typedef std::map< TransformId, TransformDescription > | TransformDescriptionMap |
Protected Member Functions | |
void | populateTransforms () |
void | populateUninstalledTransforms () |
void | populateFeatureExtractionPlugins (TransformDescriptionMap &) |
void | populateRealTimePlugins (TransformDescriptionMap &) |
std::shared_ptr< Vamp::PluginBase > | instantiateDefaultPluginFor (TransformId id, sv_samplerate_t rate) |
SearchResults | searchUnadjusted (QStringList keywords) |
Protected Attributes | |
TransformDescriptionMap | m_transforms |
bool | m_transformsPopulated |
TransformDescriptionMap | m_uninstalledTransforms |
bool | m_uninstalledTransformsPopulated |
QString | m_errorString |
QMutex | m_transformsMutex |
QMutex | m_uninstalledTransformsMutex |
UninstalledTransformsPopulateThread * | m_thread |
bool | m_exiting |
bool | m_populatingSlowly |
Static Protected Attributes | |
static TransformFactory * | m_instance = new TransformFactory |
Detailed Description
Definition at line 34 of file TransformFactory.h.
Member Typedef Documentation
typedef std::map<TransformId, TextMatcher::Match> TransformFactory::SearchResults |
Definition at line 78 of file TransformFactory.h.
|
protected |
Definition at line 207 of file TransformFactory.h.
Member Enumeration Documentation
Enumerator | |
---|---|
TransformUnknown | |
TransformInstalled | |
TransformNotInstalled |
Definition at line 65 of file TransformFactory.h.
Constructor & Destructor Documentation
TransformFactory::TransformFactory | ( | ) |
Definition at line 61 of file TransformFactory.cpp.
|
virtual |
Definition at line 70 of file TransformFactory.cpp.
Member Function Documentation
|
static |
Definition at line 48 of file TransformFactory.cpp.
References m_instance.
Referenced by ModelTransformerFactory::getConfigurationForTransform(), FeatureExtractionModelTransformer::initialise(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), and ModelTransformerFactory::transformMultiple().
|
static |
Definition at line 54 of file TransformFactory.cpp.
References m_instance, and SVDEBUG.
void TransformFactory::startPopulationThread | ( | ) |
TransformFactory has a background thread that can populate uninstalled transforms from network RDF resources.
It is not started by default, but it's a good idea to start it when the program starts up, if the uninstalled transforms may be of use later; otherwise there will be a bottleneck the first time they're requested.
If this thread is not already running, start it now.
Definition at line 86 of file TransformFactory.cpp.
References m_thread, and m_uninstalledTransformsMutex.
TransformList TransformFactory::getAllTransformDescriptions | ( | ) |
Definition at line 112 of file TransformFactory.cpp.
References m_transforms, and populateTransforms().
TransformDescription TransformFactory::getTransformDescription | ( | TransformId | id | ) |
Definition at line 136 of file TransformFactory.cpp.
References m_transforms, and populateTransforms().
bool TransformFactory::haveInstalledTransforms | ( | ) |
Definition at line 148 of file TransformFactory.cpp.
References m_transforms, and populateTransforms().
TransformList TransformFactory::getUninstalledTransformDescriptions | ( | ) |
Definition at line 155 of file TransformFactory.cpp.
References m_populatingSlowly, m_uninstalledTransforms, and populateUninstalledTransforms().
TransformDescription TransformFactory::getUninstalledTransformDescription | ( | TransformId | id | ) |
Definition at line 181 of file TransformFactory.cpp.
References m_populatingSlowly, m_uninstalledTransforms, and populateUninstalledTransforms().
bool TransformFactory::haveUninstalledTransforms | ( | bool | waitForCheckToComplete = false | ) |
Definition at line 194 of file TransformFactory.cpp.
References m_uninstalledTransforms, m_uninstalledTransformsMutex, m_uninstalledTransformsPopulated, and populateUninstalledTransforms().
TransformFactory::TransformInstallStatus TransformFactory::getTransformInstallStatus | ( | TransformId | id | ) |
Definition at line 213 of file TransformFactory.cpp.
References m_populatingSlowly, m_transforms, m_uninstalledTransforms, m_uninstalledTransformsMutex, m_uninstalledTransformsPopulated, populateTransforms(), populateUninstalledTransforms(), TransformInstalled, TransformNotInstalled, and TransformUnknown.
std::vector< TransformDescription::Type > TransformFactory::getAllTransformTypes | ( | ) |
Definition at line 245 of file TransformFactory.cpp.
References m_transforms, and populateTransforms().
std::vector< QString > TransformFactory::getTransformCategories | ( | TransformDescription::Type | transformType | ) |
Definition at line 263 of file TransformFactory.cpp.
References TransformDescription::compareUserStrings(), m_transforms, and populateTransforms().
std::vector< QString > TransformFactory::getTransformMakers | ( | TransformDescription::Type | transformType | ) |
Definition at line 290 of file TransformFactory.cpp.
References TransformDescription::compareUserStrings(), m_transforms, and populateTransforms().
QString TransformFactory::getTransformTypeName | ( | TransformDescription::Type | type | ) | const |
Definition at line 317 of file TransformFactory.cpp.
References TransformDescription::Analysis, TransformDescription::Effects, TransformDescription::EffectsData, TransformDescription::Generator, and TransformDescription::UnknownType.
Referenced by searchUnadjusted().
TransformFactory::SearchResults TransformFactory::search | ( | QString | keyword | ) |
Definition at line 1122 of file TransformFactory.cpp.
TransformFactory::SearchResults TransformFactory::search | ( | QStringList | keywords | ) |
Definition at line 1130 of file TransformFactory.cpp.
References populateTransforms(), and searchUnadjusted().
bool TransformFactory::havePopulated | ( | ) |
Return true if the transforms have been populated, i.e.
a call to something like haveTransform() will return without having to do the work of scanning plugins
Definition at line 330 of file TransformFactory.cpp.
References m_transformsMutex, and m_transformsPopulated.
bool TransformFactory::haveTransform | ( | TransformId | identifier | ) |
Return true if the given transform is known.
Definition at line 848 of file TransformFactory.cpp.
References m_transforms, and populateTransforms().
Transform TransformFactory::getDefaultTransformFor | ( | TransformId | identifier, |
sv_samplerate_t | rate = 0 |
||
) |
A single transform ID can lead to many possible Transforms, with different parameters and execution context settings.
Return the default one for the given transform.
Definition at line 766 of file TransformFactory.cpp.
References instantiateDefaultPluginFor(), makeContextConsistentWithPlugin(), Transform::setIdentifier(), setParametersFromPlugin(), Transform::setPluginVersion(), Transform::setSampleRate(), and SVDEBUG.
QString TransformFactory::getTransformName | ( | TransformId | identifier | ) |
Full name of a transform, suitable for putting on a menu.
Definition at line 855 of file TransformFactory.cpp.
References m_transforms.
QString TransformFactory::getTransformFriendlyName | ( | TransformId | identifier | ) |
Brief but friendly name of a transform, suitable for use as the name of the output layer.
Definition at line 863 of file TransformFactory.cpp.
References m_transforms.
Referenced by ModelTransformerFactory::transformMultiple().
QString TransformFactory::getTransformUnits | ( | TransformId | identifier | ) |
Definition at line 871 of file TransformFactory.cpp.
References m_transforms.
Referenced by RealTimeEffectModelTransformer::RealTimeEffectModelTransformer().
Provider TransformFactory::getTransformProvider | ( | TransformId | identifier | ) |
Definition at line 879 of file TransformFactory.cpp.
References m_transforms.
Vamp::Plugin::InputDomain TransformFactory::getTransformInputDomain | ( | TransformId | identifier | ) |
Definition at line 887 of file TransformFactory.cpp.
References Transform::FeatureExtraction, Transform::getType(), instantiateDefaultPluginFor(), Transform::setIdentifier(), and SVDEBUG.
bool TransformFactory::isTransformConfigurable | ( | TransformId | identifier | ) |
Return true if the transform has any configurable parameters, i.e.
if getConfigurationForTransform can ever return a non-trivial (not equivalent to empty) configuration string.
Definition at line 912 of file TransformFactory.cpp.
References m_transforms.
bool TransformFactory::getTransformChannelRange | ( | TransformId | identifier, |
int & | minChannels, | ||
int & | maxChannels | ||
) |
If the transform has a prescribed number or range of channel inputs, return true and set minChannels and maxChannels to the minimum and maximum number of channel inputs the transform can accept.
Return false if it doesn't care.
Definition at line 920 of file TransformFactory.cpp.
References RealTimePluginDescriptor::audioInputPortCount, FeatureExtractionPluginFactory::instance(), RealTimePluginFactory::instanceFor(), and RealTimePluginDescriptor::name.
std::shared_ptr< Vamp::PluginBase > TransformFactory::instantiatePluginFor | ( | const Transform & | transform | ) |
Load an appropriate plugin for the given transform and set the parameters, program and configuration strings on that plugin from the Transform object.
Note that this requires that the transform has a meaningful sample rate set, as that is used as the rate for the plugin. A Transform can legitimately have rate set at zero (= "use the rate of the input source"), so the caller will need to test for this case.
Returns the plugin thus loaded. This will be a Vamp::PluginBase, but not necessarily a Vamp::Plugin (only if the transform was a feature-extraction type – call downcastVampPlugin if you only want Vamp::Plugins). Returns nullptr if no suitable plugin was available.
Definition at line 787 of file TransformFactory.cpp.
References Transform::getIdentifier(), Transform::getSampleRate(), instantiateDefaultPluginFor(), setPluginParameters(), and SVDEBUG.
void TransformFactory::setParametersFromPlugin | ( | Transform & | transform, |
std::shared_ptr< Vamp::PluginBase > | plugin | ||
) |
Set the plugin parameters, program and configuration strings on the given Transform object from the given plugin instance.
Note that no check is made whether the plugin is actually the "correct" one for the transform.
!! record plugin & API version
!! check that this is the right plugin!
Definition at line 955 of file TransformFactory.cpp.
References RealTimePluginInstance::getConfigurePairs(), Transform::setConfiguration(), Transform::setParameters(), and Transform::setProgram().
Referenced by getDefaultTransformFor(), and setParametersFromPluginConfigurationXml().
void TransformFactory::setPluginParameters | ( | const Transform & | transform, |
std::shared_ptr< Vamp::PluginBase > | plugin | ||
) |
Set the parameters, program and configuration strings on the given plugin from the given Transform object.
!! check plugin & API version (see e.g. PluginXml::setParameters)
!! check that this is the right plugin!
Definition at line 1003 of file TransformFactory.cpp.
References Transform::getConfiguration(), Transform::getParameters(), and Transform::getProgram().
Referenced by getPluginConfigurationXml(), FeatureExtractionModelTransformer::initialise(), instantiatePluginFor(), and RealTimeEffectModelTransformer::RealTimeEffectModelTransformer().
void TransformFactory::makeContextConsistentWithPlugin | ( | Transform & | transform, |
std::shared_ptr< Vamp::PluginBase > | plugin | ||
) |
If the given Transform object has no processing step and block sizes set, set them to appropriate defaults for the given plugin.
Definition at line 1041 of file TransformFactory.cpp.
References Transform::getBlockSize(), Transform::getStepSize(), Transform::setBlockSize(), and Transform::setStepSize().
Referenced by getDefaultTransformFor(), and FeatureExtractionModelTransformer::initialise().
QString TransformFactory::getPluginConfigurationXml | ( | const Transform & | transform | ) |
Retrieve a <plugin ...
/> XML fragment that describes the plugin parameters, program and configuration data for the given transform.
This function is provided for backward compatibility only. Use Transform::toXml where compatibility with PluginXml descriptions of transforms is not required.
Definition at line 1079 of file TransformFactory.cpp.
References Transform::getIdentifier(), instantiateDefaultPluginFor(), setPluginParameters(), SVDEBUG, and PluginXml::toXml().
void TransformFactory::setParametersFromPluginConfigurationXml | ( | Transform & | transform, |
QString | xml | ||
) |
Set the plugin parameters, program and configuration strings on the given Transform object from the given <plugin ...
/> XML fragment.
This function is provided for backward compatibility only. Use Transform(QString) where compatibility with PluginXml descriptions of transforms is not required.
Definition at line 1103 of file TransformFactory.cpp.
References Transform::getIdentifier(), instantiateDefaultPluginFor(), setParametersFromPlugin(), PluginXml::setParametersFromXml(), and SVDEBUG.
|
inline |
Definition at line 198 of file TransformFactory.h.
References m_errorString, transformsPopulated(), and uninstalledTransformsPopulated().
|
signal |
Referenced by getStartupFailureReport(), and populateTransforms().
|
signal |
Referenced by getStartupFailureReport(), and populateUninstalledTransforms().
|
protected |
Definition at line 337 of file TransformFactory.cpp.
References TransformDescription::identifier, m_exiting, m_transforms, m_transformsMutex, m_transformsPopulated, TransformDescription::maker, TransformDescription::name, populateFeatureExtractionPlugins(), populateRealTimePlugins(), SVCERR, and transformsPopulated().
Referenced by getAllTransformDescriptions(), getAllTransformTypes(), getTransformCategories(), getTransformDescription(), getTransformInstallStatus(), getTransformMakers(), haveInstalledTransforms(), haveTransform(), instantiateDefaultPluginFor(), populateUninstalledTransforms(), and search().
|
protected |
!! This will be amazingly slow
!! basically duplicated from above
!!???
Definition at line 646 of file TransformFactory.cpp.
References TransformDescription::Analysis, TransformDescription::category, TransformDescription::configurable, TransformDescription::description, TransformDescription::friendlyName, Transform::getIdentifierForPluginOutput(), PluginRDFIndexer::getIndexedPluginIds(), PluginRDFIndexer::getInstance(), PluginRDFDescription::getOutputIds(), PluginRDFDescription::getOutputName(), PluginRDFDescription::getPluginDescription(), PluginRDFDescription::getPluginMaker(), PluginRDFDescription::getPluginName(), PluginRDFDescription::getPluginProvider(), TransformDescription::identifier, PluginRDFIndexer::indexConfiguredURLs(), TransformDescription::longDescription, m_exiting, m_transforms, m_uninstalledTransforms, m_uninstalledTransformsMutex, m_uninstalledTransformsPopulated, TransformDescription::maker, TransformDescription::name, PluginRDFIndexer::performConsistencyChecks(), populateTransforms(), TransformDescription::provider, SVCERR, TransformDescription::type, uninstalledTransformsPopulated(), and TransformDescription::units.
Referenced by getTransformInstallStatus(), getUninstalledTransformDescription(), getUninstalledTransformDescriptions(), and haveUninstalledTransforms().
|
protected |
!! return to this QString units = outputs[j].unit.c_str();
!! units,
Definition at line 416 of file TransformFactory.cpp.
References TransformDescription::Analysis, FeatureExtractionPluginFactory::getPluginCategory(), FeatureExtractionPluginFactory::getPluginIdentifiers(), FeatureExtractionPluginFactory::getPluginStaticData(), in_range_for(), FeatureExtractionPluginFactory::instance(), m_errorString, and m_exiting.
Referenced by populateTransforms().
|
protected |
!! if (descriptor.controlOutputPortCount == 0 ||
Definition at line 511 of file TransformFactory.cpp.
References RealTimePluginDescriptor::audioInputPortCount, RealTimePluginDescriptor::audioOutputPortCount, RealTimePluginDescriptor::controlOutputPortCount, RealTimePluginDescriptor::controlOutputPortNames, TransformDescription::Effects, TransformDescription::EffectsData, TransformDescription::Generator, RealTimePluginFactory::getAllPluginIdentifiers(), RealTimePluginFactory::getPluginCategory(), RealTimePluginFactory::getPluginDescriptor(), in_range_for(), RealTimePluginFactory::instanceFor(), RealTimePluginDescriptor::isSynth, m_exiting, RealTimePluginDescriptor::maker, RealTimePluginDescriptor::name, and RealTimePluginDescriptor::parameterCount.
Referenced by populateTransforms().
|
protected |
Definition at line 803 of file TransformFactory.cpp.
References Transform::FeatureExtraction, Transform::getPluginIdentifier(), Transform::getType(), FeatureExtractionPluginFactory::instance(), RealTimePluginFactory::instanceFor(), FeatureExtractionPluginFactory::instantiatePlugin(), RealTimePluginFactory::instantiatePlugin(), populateTransforms(), Transform::RealTimeEffect, Transform::setIdentifier(), and SVDEBUG.
Referenced by getDefaultTransformFor(), getPluginConfigurationXml(), getTransformInputDomain(), instantiatePluginFor(), and setParametersFromPluginConfigurationXml().
|
protected |
Definition at line 1162 of file TransformFactory.cpp.
References getTransformTypeName(), TextMatcher::Match::key, m_populatingSlowly, m_transforms, m_uninstalledTransforms, m_uninstalledTransformsMutex, m_uninstalledTransformsPopulated, TextMatcher::Match::score, SVCERR, SVDEBUG, and TextMatcher::test().
Referenced by search().
Member Data Documentation
|
protected |
Definition at line 209 of file TransformFactory.h.
Referenced by getAllTransformDescriptions(), getAllTransformTypes(), getTransformCategories(), getTransformDescription(), getTransformFriendlyName(), getTransformInstallStatus(), getTransformMakers(), getTransformName(), getTransformProvider(), getTransformUnits(), haveInstalledTransforms(), haveTransform(), isTransformConfigurable(), populateTransforms(), populateUninstalledTransforms(), and searchUnadjusted().
|
protected |
Definition at line 210 of file TransformFactory.h.
Referenced by havePopulated(), and populateTransforms().
|
protected |
Definition at line 212 of file TransformFactory.h.
Referenced by getTransformInstallStatus(), getUninstalledTransformDescription(), getUninstalledTransformDescriptions(), haveUninstalledTransforms(), populateUninstalledTransforms(), and searchUnadjusted().
|
protected |
Definition at line 213 of file TransformFactory.h.
Referenced by getTransformInstallStatus(), haveUninstalledTransforms(), populateUninstalledTransforms(), and searchUnadjusted().
|
protected |
Definition at line 215 of file TransformFactory.h.
Referenced by getStartupFailureReport(), and populateFeatureExtractionPlugins().
|
protected |
Definition at line 223 of file TransformFactory.h.
Referenced by havePopulated(), and populateTransforms().
|
protected |
Definition at line 224 of file TransformFactory.h.
Referenced by getTransformInstallStatus(), haveUninstalledTransforms(), populateUninstalledTransforms(), searchUnadjusted(), and startPopulationThread().
|
protected |
Definition at line 236 of file TransformFactory.h.
Referenced by startPopulationThread(), and ~TransformFactory().
|
protected |
Definition at line 237 of file TransformFactory.h.
Referenced by populateFeatureExtractionPlugins(), populateRealTimePlugins(), populateTransforms(), populateUninstalledTransforms(), and ~TransformFactory().
|
protected |
Definition at line 238 of file TransformFactory.h.
Referenced by getTransformInstallStatus(), getUninstalledTransformDescription(), getUninstalledTransformDescriptions(), and searchUnadjusted().
|
staticprotected |
Definition at line 242 of file TransformFactory.h.
Referenced by deleteInstance(), and getInstance().
The documentation for this class was generated from the following files:
Generated by
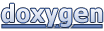