svcore
1.9
|
TextMatcher.cpp
Go to the documentation of this file.
void test(Match &match, QStringList keywords, QString text, QString textType, int score)
Definition: TextMatcher.cpp:27
Definition: TextMatcher.h:33
Generated by
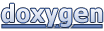