svcore
1.9
|
TransformDescription.h
Go to the documentation of this file.
TransformDescription(Type _type, QString _category, TransformId _identifier, QString _name, QString _friendlyName, QString _description, QString _longDescription, QString _maker, QString _units, bool _configurable)
Definition: TransformDescription.h:60
TransformDescription()
Definition: TransformDescription.h:58
static bool compareUserStrings(QString s1, QString s2)
Definition: TransformDescription.h:85
Definition: Provider.h:22
bool operator<(const TransformDescription &od) const
Definition: TransformDescription.h:89
Generated by
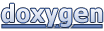