svcore
1.9
|
PluginRDFDescription.cpp
Go to the documentation of this file.
357 SVDEBUG << "ERROR: PluginRDFDescription::indexURL: No valid URI for output " << output << " of plugin <" << m_pluginUri << ">" << endl;
363 SVDEBUG << "ERROR: PluginRDFDescription::indexURL: No vamp:identifier for output <" << output << ">" << endl;
387 // SVDEBUG << "output " << output << " -> id " << outputId << ", type " << outputType << ", unit "
QString getURIForPluginId(QString pluginId)
Definition: PluginRDFIndexer.cpp:210
Definition: PluginRDFIndexer.h:29
PluginRDFDescription()
Definition: PluginRDFDescription.h:31
OutputStringMap m_outputSignalTypeURIMap
Definition: PluginRDFDescription.h:73
Provider getPluginProvider() const
Definition: PluginRDFDescription.cpp:85
bool haveDescription() const
Definition: PluginRDFDescription.cpp:61
OutputStringMap m_outputNames
Definition: PluginRDFDescription.h:69
QString getOutputName(QString outputId) const
Definition: PluginRDFDescription.cpp:102
QStringList getOutputIds() const
Definition: PluginRDFDescription.cpp:91
QString getOutputUnit(QString outputId) const
Definition: PluginRDFDescription.cpp:150
QString getOutputFeatureAttributeURI(QString outputId) const
Definition: PluginRDFDescription.cpp:130
Definition: Provider.h:29
Definition: Provider.h:32
QString getOutputSignalTypeURI(QString outputId) const
Definition: PluginRDFDescription.cpp:140
QString m_pluginDescription
Definition: PluginRDFDescription.h:66
const Dataquay::BasicStore * getIndex()
Definition: PluginRDFIndexer.cpp:73
QString getPluginDescription() const
Definition: PluginRDFDescription.cpp:73
Definition: Provider.h:31
OutputDispositionMap m_outputDispositions
Definition: PluginRDFDescription.h:70
OutputDisposition getOutputDisposition(QString outputId) const
Definition: PluginRDFDescription.cpp:111
QString getOutputEventTypeURI(QString outputId) const
Definition: PluginRDFDescription.cpp:120
QString getPluginMaker() const
Definition: PluginRDFDescription.cpp:79
QString getPluginName() const
Definition: PluginRDFDescription.cpp:67
OutputStringMap m_outputEventTypeURIMap
Definition: PluginRDFDescription.h:71
OutputStringMap m_outputUnitMap
Definition: PluginRDFDescription.h:74
~PluginRDFDescription()
Definition: PluginRDFDescription.cpp:56
Definition: Provider.h:30
Definition: Provider.h:28
static PluginRDFIndexer * getInstance()
Definition: PluginRDFIndexer.cpp:57
Definition: Provider.h:22
OutputStringMap m_outputFeatureAttributeURIMap
Definition: PluginRDFDescription.h:72
QString getOutputUri(QString outputId) const
Definition: PluginRDFDescription.cpp:159
OutputStringMap m_outputUriMap
Definition: PluginRDFDescription.h:75
Generated by
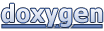