svcore
1.9
|
RealTimePluginFactory.cpp
Go to the documentation of this file.
47 // SVDEBUG << "RealTimePluginFactory::instance(" << pluginType// << "): creating new LADSPAPluginFactory" << endl;
54 // SVDEBUG << "RealTimePluginFactory::instance(" << pluginType// << "): creating new DSSIPluginFactory" << endl;
Definition: RealTimePluginFactory.h:50
virtual ~RealTimePluginFactory()
Definition: RealTimePluginFactory.cpp:38
static void enumerateAllPlugins(std::vector< QString > &)
Definition: RealTimePluginFactory.cpp:110
static RealTimePluginFactory * instanceFor(QString identifier)
Definition: RealTimePluginFactory.cpp:65
void discoverPlugins() override
Look up the plugin path and find the plugins in it.
Definition: LADSPAPluginFactory.cpp:595
static void parseIdentifier(QString identifier, QString &type, QString &soName, QString &label)
Definition: PluginIdentifier.cpp:43
static std::vector< QString > getAllPluginIdentifiers()
Definition: RealTimePluginFactory.cpp:73
static sv_samplerate_t m_sampleRate
Definition: RealTimePluginFactory.h:112
Definition: LADSPAPluginFactory.h:36
Definition: DSSIPluginFactory.h:33
static RealTimePluginFactory * instance(QString pluginType)
Definition: RealTimePluginFactory.cpp:43
virtual const std::vector< QString > & getPluginIdentifiers() const =0
Return a reference to a list of all plugin identifiers that can be created by this factory...
virtual void enumeratePlugins(std::vector< QString > &list)=0
Append to the given list descriptions of all the available plugins and their ports.
Generated by
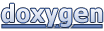