svcore
1.9
|
#include <DSSIPluginFactory.h>
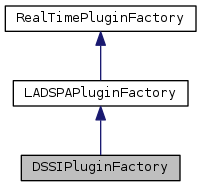
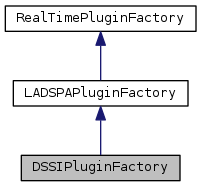
Public Member Functions | |
virtual | ~DSSIPluginFactory () |
void | enumeratePlugins (std::vector< QString > &list) override |
Append to the given list descriptions of all the available plugins and their ports. More... | |
std::shared_ptr< RealTimePluginInstance > | instantiatePlugin (QString identifier, int clientId, int position, sv_samplerate_t sampleRate, int blockSize, int channels) override |
Instantiate a plugin. More... | |
void | discoverPlugins () override |
Look up the plugin path and find the plugins in it. More... | |
const std::vector< QString > & | getPluginIdentifiers () const override |
Return a reference to a list of all plugin identifiers that can be created by this factory. More... | |
RealTimePluginDescriptor | getPluginDescriptor (QString identifier) const override |
Get some basic information about a plugin (rapidly). More... | |
QString | getPluginCategory (QString identifier) override |
Get category metadata about a plugin (without instantiating it). More... | |
QString | getPluginLibraryPath (QString identifier) override |
Get the full file path (including both directory and filename) of the library file that provides a given plugin identifier. More... | |
float | getPortMinimum (const LADSPA_Descriptor *, int port) |
float | getPortMaximum (const LADSPA_Descriptor *, int port) |
float | getPortDefault (const LADSPA_Descriptor *, int port) |
float | getPortQuantization (const LADSPA_Descriptor *, int port) |
int | getPortDisplayHint (const LADSPA_Descriptor *, int port) |
Static Public Member Functions | |
static std::vector< QString > | getPluginPath () |
static RealTimePluginFactory * | instance (QString pluginType) |
static RealTimePluginFactory * | instanceFor (QString identifier) |
static std::vector< QString > | getAllPluginIdentifiers () |
static void | enumerateAllPlugins (std::vector< QString > &) |
static void | setSampleRate (sv_samplerate_t sampleRate) |
Protected Types | |
typedef std::map< QString, void * > | LibraryHandleMap |
Protected Member Functions | |
DSSIPluginFactory () | |
PluginScan::PluginType | getPluginType () const override |
std::vector< QString > | getLRDFPath (QString &baseUri) override |
void | discoverPluginsFrom (QString soName) override |
const LADSPA_Descriptor * | getLADSPADescriptor (QString identifier) override |
virtual const DSSI_Descriptor * | getDSSIDescriptor (QString identifier) |
virtual void | generateTaxonomy (QString uri, QString base) |
virtual void | generateFallbackCategories () |
void | loadLibrary (QString soName) |
void | unloadLibrary (QString soName) |
void | unloadUnusedLibraries () |
Protected Attributes | |
DSSI_Host_Descriptor | m_hostDescriptor |
std::vector< QString > | m_identifiers |
std::map< QString, QString > | m_libraries |
std::map< QString, RealTimePluginDescriptor > | m_rtDescriptors |
std::map< QString, QString > | m_taxonomy |
std::map< unsigned long, QString > | m_lrdfTaxonomy |
std::map< unsigned long, std::map< int, float > > | m_portDefaults |
std::set< std::weak_ptr< RealTimePluginInstance >, std::owner_less< std::weak_ptr< RealTimePluginInstance > > > | m_instances |
LibraryHandleMap | m_libraryHandles |
Static Protected Attributes | |
static sv_samplerate_t | m_sampleRate = 48000 |
Friends | |
class | RealTimePluginFactory |
Detailed Description
Definition at line 33 of file DSSIPluginFactory.h.
Member Typedef Documentation
|
protectedinherited |
Definition at line 101 of file LADSPAPluginFactory.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 54 of file DSSIPluginFactory.cpp.
|
protected |
Definition at line 44 of file DSSIPluginFactory.cpp.
References m_hostDescriptor, DSSIPluginInstance::midiSend(), DSSIPluginInstance::requestMidiSend(), and DSSIPluginInstance::requestNonRTThread().
Member Function Documentation
|
overridevirtual |
Append to the given list descriptions of all the available plugins and their ports.
This is in a standard format, see the LADSPA implementation for details.
Implements RealTimePluginFactory.
Definition at line 60 of file DSSIPluginFactory.cpp.
References PortType::Audio, PortType::Control, getDSSIDescriptor(), LADSPAPluginFactory::getPortDefault(), LADSPAPluginFactory::getPortDisplayHint(), LADSPAPluginFactory::getPortMaximum(), LADSPAPluginFactory::getPortMinimum(), PortType::Input, LADSPAPluginFactory::m_identifiers, LADSPAPluginFactory::m_taxonomy, PortType::Output, and LADSPAPluginFactory::unloadUnusedLibraries().
|
overridevirtual |
Instantiate a plugin.
Implements RealTimePluginFactory.
Definition at line 114 of file DSSIPluginFactory.cpp.
References getDSSIDescriptor(), RealTimePluginFactory::instance(), and LADSPAPluginFactory::m_instances.
|
static |
Definition at line 208 of file DSSIPluginFactory.cpp.
References DEFAULT_DSSI_PATH, getEnvUtf8(), and PATH_SEPARATOR.
Referenced by getLRDFPath().
|
inlineoverrideprotectedvirtual |
Reimplemented from LADSPAPluginFactory.
Definition at line 54 of file DSSIPluginFactory.h.
References discoverPluginsFrom(), PluginScan::DSSIPlugin, getDSSIDescriptor(), getLADSPADescriptor(), and getLRDFPath().
|
overrideprotectedvirtual |
Reimplemented from LADSPAPluginFactory.
Definition at line 256 of file DSSIPluginFactory.cpp.
References getPluginPath().
Referenced by getPluginType().
|
overrideprotectedvirtual |
Reimplemented from LADSPAPluginFactory.
Definition at line 289 of file DSSIPluginFactory.cpp.
References RealTimePluginDescriptor::audioInputPortCount, RealTimePluginDescriptor::audioOutputPortCount, RealTimePluginDescriptor::category, RealTimePluginDescriptor::controlOutputPortCount, RealTimePluginDescriptor::controlOutputPortNames, RealTimePluginDescriptor::copyright, PluginIdentifier::createIdentifier(), DLCLOSE, DLERROR, DLOPEN, DLSYM, RealTimePluginDescriptor::isSynth, RealTimePluginDescriptor::label, LADSPAPluginFactory::m_identifiers, LADSPAPluginFactory::m_libraries, LADSPAPluginFactory::m_lrdfTaxonomy, LADSPAPluginFactory::m_portDefaults, LADSPAPluginFactory::m_rtDescriptors, LADSPAPluginFactory::m_taxonomy, RealTimePluginDescriptor::maker, RealTimePluginDescriptor::name, RealTimePluginDescriptor::parameterCount, and SVCERR.
Referenced by getPluginType().
|
overrideprotectedvirtual |
Reimplemented from LADSPAPluginFactory.
Definition at line 199 of file DSSIPluginFactory.cpp.
References getDSSIDescriptor().
Referenced by getPluginType().
|
protectedvirtual |
Definition at line 142 of file DSSIPluginFactory.cpp.
References PluginIdentifier::BUILTIN_PLUGIN_SONAME, DLSYM, SamplePlayer::getDescriptor(), LADSPAPluginFactory::loadLibrary(), m_hostDescriptor, LADSPAPluginFactory::m_libraryHandles, PluginIdentifier::parseIdentifier(), and SVCERR.
Referenced by enumeratePlugins(), getLADSPADescriptor(), getPluginType(), and instantiatePlugin().
|
overridevirtualinherited |
Look up the plugin path and find the plugins in it.
Called automatically after construction of a factory.
Implements RealTimePluginFactory.
Definition at line 595 of file LADSPAPluginFactory.cpp.
References LADSPAPluginFactory::discoverPluginsFrom(), LADSPAPluginFactory::generateFallbackCategories(), LADSPAPluginFactory::generateTaxonomy(), PluginScan::getCandidateLibrariesFor(), PluginScan::getInstance(), LADSPAPluginFactory::getLRDFPath(), LADSPAPluginFactory::getPluginPath(), and LADSPAPluginFactory::getPluginType().
Referenced by RealTimePluginFactory::instance().
|
overridevirtualinherited |
Return a reference to a list of all plugin identifiers that can be created by this factory.
Implements RealTimePluginFactory.
Definition at line 62 of file LADSPAPluginFactory.cpp.
References LADSPAPluginFactory::m_identifiers.
|
overridevirtualinherited |
Get some basic information about a plugin (rapidly).
Implements RealTimePluginFactory.
Definition at line 136 of file LADSPAPluginFactory.cpp.
References LADSPAPluginFactory::m_rtDescriptors.
|
overridevirtualinherited |
Get category metadata about a plugin (without instantiating it).
Implements RealTimePluginFactory.
Definition at line 852 of file LADSPAPluginFactory.cpp.
References LADSPAPluginFactory::m_taxonomy.
|
overridevirtualinherited |
Get the full file path (including both directory and filename) of the library file that provides a given plugin identifier.
Note getPluginIdentifiers() must have been called before this has access to the necessary information.
Implements RealTimePluginFactory.
Definition at line 68 of file LADSPAPluginFactory.cpp.
References LADSPAPluginFactory::m_libraries.
|
inherited |
Definition at line 149 of file LADSPAPluginFactory.cpp.
References RealTimePluginFactory::m_sampleRate.
Referenced by enumeratePlugins(), LADSPAPluginFactory::enumeratePlugins(), LADSPAPluginInstance::getParameterDescriptors(), DSSIPluginInstance::getParameterDescriptors(), LADSPAPluginFactory::getPortDefault(), LADSPAPluginFactory::getPortQuantization(), LADSPAPluginInstance::setParameterValue(), and DSSIPluginInstance::setParameterValue().
|
inherited |
Definition at line 176 of file LADSPAPluginFactory.cpp.
References RealTimePluginFactory::m_sampleRate.
Referenced by enumeratePlugins(), LADSPAPluginFactory::enumeratePlugins(), LADSPAPluginInstance::getParameterDescriptors(), DSSIPluginInstance::getParameterDescriptors(), LADSPAPluginFactory::getPortDefault(), LADSPAPluginFactory::getPortQuantization(), LADSPAPluginInstance::setParameterValue(), and DSSIPluginInstance::setParameterValue().
|
inherited |
!! No – the min and max have already been multiplied by the rate,
Definition at line 199 of file LADSPAPluginFactory.cpp.
References Preferences::getInstance(), LADSPAPluginFactory::getPortMaximum(), LADSPAPluginFactory::getPortMinimum(), Preferences::getTuningFrequency(), and LADSPAPluginFactory::m_portDefaults.
Referenced by LADSPAPluginInstance::connectPorts(), DSSIPluginInstance::connectPorts(), enumeratePlugins(), LADSPAPluginFactory::enumeratePlugins(), LADSPAPluginInstance::getParameterDefault(), DSSIPluginInstance::getParameterDefault(), LADSPAPluginInstance::getParameterDescriptors(), and DSSIPluginInstance::getParameterDescriptors().
|
inherited |
Definition at line 303 of file LADSPAPluginFactory.cpp.
References LADSPAPluginFactory::getPortDisplayHint(), LADSPAPluginFactory::getPortMaximum(), LADSPAPluginFactory::getPortMinimum(), PortHint::Integer, and PortHint::Toggled.
Referenced by LADSPAPluginInstance::getParameterDescriptors(), and DSSIPluginInstance::getParameterDescriptors().
|
inherited |
Definition at line 317 of file LADSPAPluginFactory.cpp.
References PortHint::Integer, PortHint::Logarithmic, PortHint::NoHint, and PortHint::Toggled.
Referenced by enumeratePlugins(), LADSPAPluginFactory::enumeratePlugins(), LADSPAPluginInstance::getParameterDisplayHint(), DSSIPluginInstance::getParameterDisplayHint(), and LADSPAPluginFactory::getPortQuantization().
|
protectedvirtualinherited |
Definition at line 822 of file LADSPAPluginFactory.cpp.
References LADSPAPluginFactory::m_lrdfTaxonomy.
Referenced by LADSPAPluginFactory::discoverPlugins(), and LADSPAPluginFactory::getPluginType().
|
protectedvirtualinherited |
Definition at line 774 of file LADSPAPluginFactory.cpp.
References PluginIdentifier::canonicalise(), LADSPAPluginFactory::getPluginPath(), and LADSPAPluginFactory::m_taxonomy.
Referenced by LADSPAPluginFactory::discoverPlugins(), and LADSPAPluginFactory::getPluginType().
|
protectedinherited |
Definition at line 402 of file LADSPAPluginFactory.cpp.
References DLERROR, DLOPEN, LADSPAPluginFactory::getPluginPath(), LADSPAPluginFactory::m_libraryHandles, PLUGIN_GLOB, SVCERR, and SVDEBUG.
Referenced by getDSSIDescriptor(), LADSPAPluginFactory::getLADSPADescriptor(), and LADSPAPluginFactory::getPluginType().
|
protectedinherited |
Definition at line 463 of file LADSPAPluginFactory.cpp.
References DLCLOSE, and LADSPAPluginFactory::m_libraryHandles.
Referenced by LADSPAPluginFactory::getPluginType(), and LADSPAPluginFactory::unloadUnusedLibraries().
|
protectedinherited |
Definition at line 474 of file LADSPAPluginFactory.cpp.
References PluginIdentifier::BUILTIN_PLUGIN_SONAME, LADSPAPluginFactory::m_instances, LADSPAPluginFactory::m_libraryHandles, PluginIdentifier::parseIdentifier(), and LADSPAPluginFactory::unloadLibrary().
Referenced by enumeratePlugins(), LADSPAPluginFactory::enumeratePlugins(), LADSPAPluginFactory::getPluginType(), and LADSPAPluginFactory::~LADSPAPluginFactory().
|
staticinherited |
Definition at line 43 of file RealTimePluginFactory.cpp.
References _dssiInstance, _ladspaInstance, and LADSPAPluginFactory::discoverPlugins().
Referenced by RealTimePluginFactory::enumerateAllPlugins(), RealTimePluginFactory::getAllPluginIdentifiers(), RealTimePluginFactory::instanceFor(), instantiatePlugin(), LADSPAPluginFactory::instantiatePlugin(), DSSIPluginInstance::requestNonRTThread(), and DSSIPluginInstance::runGrouped().
|
staticinherited |
Definition at line 65 of file RealTimePluginFactory.cpp.
References RealTimePluginFactory::instance(), and PluginIdentifier::parseIdentifier().
Referenced by ModelTransformerFactory::createTransformer(), ModelTransformerFactory::getConfigurationForTransform(), TransformFactory::getTransformChannelRange(), Transform::getType(), TransformFactory::instantiateDefaultPluginFor(), TransformFactory::populateRealTimePlugins(), and RealTimeEffectModelTransformer::RealTimeEffectModelTransformer().
|
staticinherited |
Definition at line 73 of file RealTimePluginFactory.cpp.
References RealTimePluginFactory::getPluginIdentifiers(), RealTimePluginFactory::instance(), and RestoreStartupLocale().
Referenced by TransformFactory::populateRealTimePlugins().
|
staticinherited |
Definition at line 110 of file RealTimePluginFactory.cpp.
References RealTimePluginFactory::enumeratePlugins(), RealTimePluginFactory::instance(), and RestoreStartupLocale().
|
inlinestaticinherited |
Definition at line 60 of file RealTimePluginFactory.h.
Friends And Related Function Documentation
|
friend |
Definition at line 52 of file DSSIPluginFactory.h.
Member Data Documentation
|
protected |
Definition at line 65 of file DSSIPluginFactory.h.
Referenced by DSSIPluginFactory(), and getDSSIDescriptor().
|
protectedinherited |
Definition at line 90 of file LADSPAPluginFactory.h.
Referenced by discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), enumeratePlugins(), LADSPAPluginFactory::enumeratePlugins(), and LADSPAPluginFactory::getPluginIdentifiers().
|
protectedinherited |
Definition at line 91 of file LADSPAPluginFactory.h.
Referenced by discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), and LADSPAPluginFactory::getPluginLibraryPath().
|
protectedinherited |
Definition at line 92 of file LADSPAPluginFactory.h.
Referenced by discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), and LADSPAPluginFactory::getPluginDescriptor().
|
protectedinherited |
Definition at line 94 of file LADSPAPluginFactory.h.
Referenced by discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), enumeratePlugins(), LADSPAPluginFactory::enumeratePlugins(), LADSPAPluginFactory::generateFallbackCategories(), and LADSPAPluginFactory::getPluginCategory().
|
protectedinherited |
Definition at line 95 of file LADSPAPluginFactory.h.
Referenced by discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), and LADSPAPluginFactory::generateTaxonomy().
|
protectedinherited |
Definition at line 96 of file LADSPAPluginFactory.h.
Referenced by discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), and LADSPAPluginFactory::getPortDefault().
|
protectedinherited |
Definition at line 99 of file LADSPAPluginFactory.h.
Referenced by instantiatePlugin(), LADSPAPluginFactory::instantiatePlugin(), and LADSPAPluginFactory::unloadUnusedLibraries().
|
protectedinherited |
Definition at line 102 of file LADSPAPluginFactory.h.
Referenced by getDSSIDescriptor(), LADSPAPluginFactory::getLADSPADescriptor(), LADSPAPluginFactory::loadLibrary(), LADSPAPluginFactory::unloadLibrary(), and LADSPAPluginFactory::unloadUnusedLibraries().
|
staticprotectedinherited |
Definition at line 112 of file RealTimePluginFactory.h.
Referenced by LADSPAPluginFactory::getPortMaximum(), and LADSPAPluginFactory::getPortMinimum().
The documentation for this class was generated from the following files:
Generated by
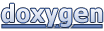