svcore
1.9
|
DSSIPluginFactory.cpp
Go to the documentation of this file.
73 // SVDEBUG << "DSSIPluginFactory::enumeratePlugins: Name " << (descriptor->Name ? descriptor->Name : "NONE" ) << endl;
164 SVCERR << "WARNING: DSSIPluginFactory::getDSSIDescriptor: loadLibrary failed for " << soname << endl;
176 SVCERR << "WARNING: DSSIPluginFactory::getDSSIDescriptor: No descriptor function in library " << soname << endl;
193 SVCERR << "WARNING: DSSIPluginFactory::getDSSIDescriptor: No such plugin as " << label << " in library " << soname << endl;
308 SVCERR << "WARNING: DSSIPluginFactory::discoverPlugins: No descriptor function in " << soname << endl;
319 SVCERR << "WARNING: DSSIPluginFactory::discoverPlugins: No LADSPA descriptor for plugin " << index << " in " << soname << endl;
388 // SVCERR << "Default for this port (" << defs->items[j].pid << ", " << defs->items[j].label << ") is " << defs->items[j].value << "; applying this to port number " << i << " with name " << ladspaDescriptor->PortNames[i] << endl;
431 SVCERR << "WARNING: DSSIPluginFactory::discoverPlugins - can't unload " << libraryHandle << endl;
virtual ~DSSIPluginFactory()
Definition: DSSIPluginFactory.cpp:54
std::vector< QString > getLRDFPath(QString &baseUri) override
Definition: DSSIPluginFactory.cpp:256
std::map< QString, QString > m_libraries
Definition: LADSPAPluginFactory.h:91
std::vector< std::string > controlOutputPortNames
Definition: RealTimePluginFactory.h:47
void enumeratePlugins(std::vector< QString > &list) override
Append to the given list descriptions of all the available plugins and their ports.
Definition: DSSIPluginFactory.cpp:60
static int requestMidiSend(LADSPA_Handle instance, unsigned char ports, unsigned char channels)
Definition: DSSIPluginInstance.cpp:1267
int getPortDisplayHint(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:317
const LADSPA_Descriptor * getLADSPADescriptor(QString identifier) override
Definition: DSSIPluginFactory.cpp:199
static void midiSend(LADSPA_Handle instance, snd_seq_event_t *events, unsigned long eventCount)
Definition: DSSIPluginInstance.cpp:1278
void discoverPluginsFrom(QString soName) override
Definition: DSSIPluginFactory.cpp:289
LibraryHandleMap m_libraryHandles
Definition: LADSPAPluginFactory.h:102
bool getEnvUtf8(std::string variable, std::string &value)
Return the value of the given environment variable by reference.
Definition: System.cpp:344
Definition: DSSIPluginInstance.h:39
static void parseIdentifier(QString identifier, QString &type, QString &soName, QString &label)
Definition: PluginIdentifier.cpp:43
static QString createIdentifier(QString type, QString soName, QString label)
Definition: PluginIdentifier.cpp:26
static std::vector< QString > getPluginPath()
Definition: DSSIPluginFactory.cpp:208
float getPortDefault(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:199
unsigned int audioInputPortCount
Definition: RealTimePluginFactory.h:44
unsigned int parameterCount
Definition: RealTimePluginFactory.h:43
unsigned int controlOutputPortCount
Definition: RealTimePluginFactory.h:46
static const DSSI_Descriptor * getDescriptor(unsigned long index)
Definition: SamplePlayer.cpp:128
std::set< std::weak_ptr< RealTimePluginInstance >, std::owner_less< std::weak_ptr< RealTimePluginInstance > > > m_instances
Definition: LADSPAPluginFactory.h:99
float getPortMaximum(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:176
static QString BUILTIN_PLUGIN_SONAME
Definition: PluginIdentifier.h:44
void unloadUnusedLibraries()
Definition: LADSPAPluginFactory.cpp:474
virtual const DSSI_Descriptor * getDSSIDescriptor(QString identifier)
Definition: DSSIPluginFactory.cpp:142
DSSI_Host_Descriptor m_hostDescriptor
Definition: DSSIPluginFactory.h:65
std::map< unsigned long, std::map< int, float > > m_portDefaults
Definition: LADSPAPluginFactory.h:96
std::shared_ptr< RealTimePluginInstance > instantiatePlugin(QString identifier, int clientId, int position, sv_samplerate_t sampleRate, int blockSize, int channels) override
Instantiate a plugin.
Definition: DSSIPluginFactory.cpp:114
std::map< QString, RealTimePluginDescriptor > m_rtDescriptors
Definition: LADSPAPluginFactory.h:92
std::map< QString, QString > m_taxonomy
Definition: LADSPAPluginFactory.h:94
static int requestNonRTThread(LADSPA_Handle instance, void(*runFunction)(LADSPA_Handle))
Definition: DSSIPluginInstance.cpp:1297
Definition: LADSPAPluginFactory.h:36
std::vector< QString > m_identifiers
Definition: LADSPAPluginFactory.h:90
std::map< unsigned long, QString > m_lrdfTaxonomy
Definition: LADSPAPluginFactory.h:95
static RealTimePluginFactory * instance(QString pluginType)
Definition: RealTimePluginFactory.cpp:43
void loadLibrary(QString soName)
Definition: LADSPAPluginFactory.cpp:402
float getPortMinimum(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:149
unsigned int audioOutputPortCount
Definition: RealTimePluginFactory.h:45
Generated by
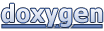