svcore
1.9
|
#include <SamplePlayer.h>
Static Public Member Functions | |
static const DSSI_Descriptor * | getDescriptor (unsigned long index) |
Private Types | |
enum | { OutputPort = 0, RetunePort = 1, BasePitchPort = 2, ConcertAPort = 3, SustainPort = 4, ReleasePort = 5, PortCount = 6 } |
enum | { Polyphony = 128 } |
Private Member Functions | |
SamplePlayer (int sampleRate) | |
~SamplePlayer () | |
void | searchSamples () |
void | loadSampleData (QString path) |
void | runImpl (unsigned long, snd_seq_event_t *, unsigned long) |
void | addSample (int, unsigned long, unsigned long) |
Static Private Member Functions | |
static LADSPA_Handle | instantiate (const LADSPA_Descriptor *, unsigned long) |
static void | connectPort (LADSPA_Handle, unsigned long, LADSPA_Data *) |
static void | activate (LADSPA_Handle) |
static void | run (LADSPA_Handle, unsigned long) |
static void | deactivate (LADSPA_Handle) |
static void | cleanup (LADSPA_Handle) |
static char * | configure (LADSPA_Handle, const char *, const char *) |
static const DSSI_Program_Descriptor * | getProgram (LADSPA_Handle, unsigned long) |
static void | selectProgram (LADSPA_Handle, unsigned long, unsigned long) |
static int | getMidiController (LADSPA_Handle, unsigned long) |
static void | runSynth (LADSPA_Handle, unsigned long, snd_seq_event_t *, unsigned long) |
static void | receiveHostDescriptor (const DSSI_Host_Descriptor *descriptor) |
static void | workThreadCallback (LADSPA_Handle) |
Private Attributes | |
float * | m_output |
float * | m_retune |
float * | m_basePitch |
float * | m_concertA |
float * | m_sustain |
float * | m_release |
float * | m_sampleData |
size_t | m_sampleCount |
int | m_sampleRate |
long | m_ons [Polyphony] |
long | m_offs [Polyphony] |
int | m_velocities [Polyphony] |
long | m_sampleNo |
QString | m_sampleDir |
QString | m_program |
std::vector< std::pair< QString, QString > > | m_samples |
bool | m_sampleSearchComplete |
int | m_pendingProgramChange |
QMutex | m_mutex |
Static Private Attributes | |
static const char *const | portNames [PortCount] |
static const LADSPA_PortDescriptor | ports [PortCount] |
static const LADSPA_PortRangeHint | hints [PortCount] |
static const LADSPA_Properties | properties = LADSPA_PROPERTY_HARD_RT_CAPABLE |
static const LADSPA_Descriptor | ladspaDescriptor |
static const DSSI_Descriptor | dssiDescriptor |
static const DSSI_Host_Descriptor * | hostDescriptor = nullptr |
Detailed Description
Definition at line 30 of file SamplePlayer.h.
Member Enumeration Documentation
|
private |
Enumerator | |
---|---|
OutputPort | |
RetunePort | |
BasePitchPort | |
ConcertAPort | |
SustainPort | |
ReleasePort | |
PortCount |
Definition at line 39 of file SamplePlayer.h.
|
private |
Enumerator | |
---|---|
Polyphony |
Definition at line 49 of file SamplePlayer.h.
Constructor & Destructor Documentation
|
private |
Definition at line 134 of file SamplePlayer.cpp.
Referenced by instantiate().
|
private |
Definition at line 151 of file SamplePlayer.cpp.
References m_sampleData.
Member Function Documentation
|
static |
Definition at line 128 of file SamplePlayer.cpp.
References dssiDescriptor.
Referenced by DSSIPluginFactory::getDSSIDescriptor().
|
staticprivate |
Definition at line 157 of file SamplePlayer.cpp.
References hostDescriptor, SamplePlayer(), SVDEBUG, and workThreadCallback().
|
staticprivate |
Definition at line 177 of file SamplePlayer.cpp.
References m_basePitch, m_concertA, m_output, m_release, m_retune, m_sustain, PortCount, and ports.
|
staticprivate |
Definition at line 195 of file SamplePlayer.cpp.
References m_mutex, m_offs, m_ons, m_sampleNo, m_velocities, and Polyphony.
Referenced by deactivate().
|
staticprivate |
Definition at line 210 of file SamplePlayer.cpp.
References runSynth().
|
staticprivate |
Definition at line 216 of file SamplePlayer.cpp.
References activate().
|
staticprivate |
Definition at line 222 of file SamplePlayer.cpp.
|
staticprivate |
Definition at line 228 of file SamplePlayer.cpp.
References m_mutex, m_sampleDir, m_sampleSearchComplete, and searchSamples().
|
staticprivate |
Definition at line 259 of file SamplePlayer.cpp.
References m_mutex, m_samples, m_sampleSearchComplete, and searchSamples().
|
staticprivate |
Definition at line 285 of file SamplePlayer.cpp.
References m_pendingProgramChange.
|
staticprivate |
Definition at line 294 of file SamplePlayer.cpp.
References PortCount.
|
staticprivate |
|
staticprivate |
Definition at line 317 of file SamplePlayer.cpp.
References hostDescriptor.
|
staticprivate |
Definition at line 323 of file SamplePlayer.cpp.
References loadSampleData(), m_mutex, m_pendingProgramChange, m_program, m_samples, m_sampleSearchComplete, searchSamples(), and SVDEBUG.
Referenced by instantiate().
|
private |
Definition at line 366 of file SamplePlayer.cpp.
References m_sampleDir, m_samples, m_sampleSearchComplete, and SVDEBUG.
Referenced by configure(), getProgram(), and workThreadCallback().
|
private |
Definition at line 394 of file SamplePlayer.cpp.
References m_mutex, m_offs, m_ons, m_sampleCount, m_sampleData, m_sampleRate, m_velocities, and Polyphony.
Referenced by workThreadCallback().
|
private |
Definition at line 491 of file SamplePlayer.cpp.
References addSample(), m_mutex, m_offs, m_ons, m_output, m_sampleCount, m_sampleData, m_sampleNo, m_sustain, m_velocities, and Polyphony.
Referenced by runSynth().
|
private |
Definition at line 573 of file SamplePlayer.cpp.
References m_basePitch, m_concertA, m_offs, m_ons, m_output, m_release, m_retune, m_sampleCount, m_sampleData, m_sampleNo, m_sampleRate, and m_velocities.
Referenced by runImpl().
Member Data Documentation
|
staticprivate |
Definition at line 53 of file SamplePlayer.h.
|
staticprivate |
Definition at line 54 of file SamplePlayer.h.
Referenced by connectPort().
|
staticprivate |
Definition at line 55 of file SamplePlayer.h.
|
staticprivate |
Definition at line 56 of file SamplePlayer.h.
|
staticprivate |
Definition at line 57 of file SamplePlayer.h.
|
staticprivate |
Definition at line 58 of file SamplePlayer.h.
Referenced by getDescriptor().
|
staticprivate |
Definition at line 59 of file SamplePlayer.h.
Referenced by instantiate(), and receiveHostDescriptor().
|
private |
Definition at line 81 of file SamplePlayer.h.
Referenced by addSample(), connectPort(), and runImpl().
|
private |
Definition at line 82 of file SamplePlayer.h.
Referenced by addSample(), and connectPort().
|
private |
Definition at line 83 of file SamplePlayer.h.
Referenced by addSample(), and connectPort().
|
private |
Definition at line 84 of file SamplePlayer.h.
Referenced by addSample(), and connectPort().
|
private |
Definition at line 85 of file SamplePlayer.h.
Referenced by connectPort(), and runImpl().
|
private |
Definition at line 86 of file SamplePlayer.h.
Referenced by addSample(), and connectPort().
|
private |
Definition at line 88 of file SamplePlayer.h.
Referenced by addSample(), loadSampleData(), runImpl(), and ~SamplePlayer().
|
private |
Definition at line 89 of file SamplePlayer.h.
Referenced by addSample(), loadSampleData(), and runImpl().
|
private |
Definition at line 90 of file SamplePlayer.h.
Referenced by addSample(), and loadSampleData().
|
private |
Definition at line 92 of file SamplePlayer.h.
Referenced by activate(), addSample(), loadSampleData(), and runImpl().
|
private |
Definition at line 93 of file SamplePlayer.h.
Referenced by activate(), addSample(), loadSampleData(), and runImpl().
|
private |
Definition at line 94 of file SamplePlayer.h.
Referenced by activate(), addSample(), loadSampleData(), and runImpl().
|
private |
Definition at line 95 of file SamplePlayer.h.
Referenced by activate(), addSample(), and runImpl().
|
private |
Definition at line 97 of file SamplePlayer.h.
Referenced by configure(), and searchSamples().
|
private |
Definition at line 98 of file SamplePlayer.h.
Referenced by workThreadCallback().
|
private |
Definition at line 99 of file SamplePlayer.h.
Referenced by getProgram(), searchSamples(), and workThreadCallback().
|
private |
Definition at line 100 of file SamplePlayer.h.
Referenced by configure(), getProgram(), searchSamples(), and workThreadCallback().
|
private |
Definition at line 101 of file SamplePlayer.h.
Referenced by selectProgram(), and workThreadCallback().
|
private |
Definition at line 103 of file SamplePlayer.h.
Referenced by activate(), configure(), getProgram(), loadSampleData(), runImpl(), and workThreadCallback().
The documentation for this class was generated from the following files:
Generated by
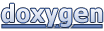