svcore
1.9
|
DSSIPluginFactory.h
Go to the documentation of this file.
virtual ~DSSIPluginFactory()
Definition: DSSIPluginFactory.cpp:54
std::vector< QString > getLRDFPath(QString &baseUri) override
Definition: DSSIPluginFactory.cpp:256
void enumeratePlugins(std::vector< QString > &list) override
Append to the given list descriptions of all the available plugins and their ports.
Definition: DSSIPluginFactory.cpp:60
PluginScan::PluginType getPluginType() const override
Definition: DSSIPluginFactory.h:54
Definition: RealTimePluginFactory.h:50
const LADSPA_Descriptor * getLADSPADescriptor(QString identifier) override
Definition: DSSIPluginFactory.cpp:199
void discoverPluginsFrom(QString soName) override
Definition: DSSIPluginFactory.cpp:289
Definition: PluginScan.h:54
Definition: DSSIPluginInstance.h:39
static std::vector< QString > getPluginPath()
Definition: DSSIPluginFactory.cpp:208
virtual const DSSI_Descriptor * getDSSIDescriptor(QString identifier)
Definition: DSSIPluginFactory.cpp:142
DSSI_Host_Descriptor m_hostDescriptor
Definition: DSSIPluginFactory.h:65
std::shared_ptr< RealTimePluginInstance > instantiatePlugin(QString identifier, int clientId, int position, sv_samplerate_t sampleRate, int blockSize, int channels) override
Instantiate a plugin.
Definition: DSSIPluginFactory.cpp:114
Definition: LADSPAPluginFactory.h:36
Definition: DSSIPluginFactory.h:33
Generated by
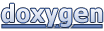