svcore
1.9
|
DSSIPluginInstance.h
Go to the documentation of this file.
217 void (*m_runFunction)(LADSPA_Handle);
std::vector< LADSPA_Data > m_backupControlPortsIn
Definition: DSSIPluginInstance.h:151
std::vector< std::pair< int, LADSPA_Data * > > m_controlPortsOut
Definition: DSSIPluginInstance.h:149
static int requestMidiSend(LADSPA_Handle instance, unsigned char ports, unsigned char channels)
Definition: DSSIPluginInstance.cpp:1267
std::string configure(std::string key, std::string value) override
Definition: DSSIPluginInstance.cpp:866
static std::map< LADSPA_Handle, std::set< NonRTPluginThread * > > m_threads
Definition: DSSIPluginInstance.h:220
Definition: RealTimePluginFactory.h:50
float getControlOutputValue(int n) const override
Definition: DSSIPluginInstance.cpp:821
int getAudioOutputCount() const override
Definition: DSSIPluginInstance.h:76
static void midiSend(LADSPA_Handle instance, snd_seq_event_t *events, unsigned long eventCount)
Definition: DSSIPluginInstance.cpp:1278
std::map< int, int > m_controllerMap
Definition: DSSIPluginInstance.h:153
int getPluginVersion() const override
Definition: DSSIPluginInstance.cpp:134
Definition: DSSIPluginInstance.h:39
bool handleController(snd_seq_event_t *ev)
Definition: DSSIPluginInstance.cpp:962
A very simple class that facilitates running things like plugins without locking, by collecting unwan...
Definition: Scavenger.h:44
NonRTPluginThread(LADSPA_Handle handle, void(*runFunction)(LADSPA_Handle))
Definition: DSSIPluginInstance.h:206
void setParameterValue(int parameter, float value) override
Definition: DSSIPluginInstance.cpp:758
static snd_seq_event_t ** m_groupLocalEventBuffers
Definition: DSSIPluginInstance.h:198
std::string getName() const override
Definition: DSSIPluginInstance.cpp:116
sample_t ** getAudioOutputBuffers() override
Definition: DSSIPluginInstance.h:78
RingBuffer< snd_seq_event_t > m_eventBuffer
Definition: DSSIPluginInstance.h:173
int getParameterDisplayHint(int parameter) const override
Definition: DSSIPluginInstance.cpp:852
std::string getMaker() const override
Definition: DSSIPluginInstance.cpp:128
void selectProgram(std::string program) override
Definition: DSSIPluginInstance.cpp:612
int getControlOutputCount() const override
Definition: DSSIPluginInstance.h:80
void selectProgramAux(std::string program, bool backupPortValues)
Definition: DSSIPluginInstance.cpp:618
LADSPA_Handle m_handle
Definition: DSSIPluginInstance.h:216
void run(const RealTime &, int count=0) override
Run for one block, starting at the given time.
Definition: DSSIPluginInstance.cpp:992
std::vector< ProgramDescriptor > m_cachedPrograms
Definition: DSSIPluginInstance.h:170
int getParameterCount() const override
Definition: DSSIPluginInstance.cpp:752
std::vector< int > m_audioPortsOut
Definition: DSSIPluginInstance.h:156
QString getPluginIdentifier() const override
Definition: DSSIPluginInstance.h:47
void setIdealChannelCount(int channels) override
Definition: DSSIPluginInstance.cpp:316
bool m_haveLastEventSendTime
Definition: DSSIPluginInstance.h:191
std::string getProgram(int bank, int program) const override
Definition: DSSIPluginInstance.cpp:562
const DSSI_Descriptor * m_descriptor
Definition: DSSIPluginInstance.h:146
float getParameter(std::string) const override
Definition: DSSIPluginInstance.cpp:179
sv_frame_t getLatency() override
Definition: DSSIPluginInstance.cpp:273
static Scavenger< ScavengerArrayWrapper< snd_seq_event_t * > > m_bufferScavenger
Definition: DSSIPluginInstance.h:201
virtual ~DSSIPluginInstance()
Definition: DSSIPluginInstance.cpp:404
ParameterList getParameterDescriptors() const override
Definition: DSSIPluginInstance.cpp:146
void setExiting()
Definition: DSSIPluginInstance.h:213
sample_t ** getAudioInputBuffers() override
Definition: DSSIPluginInstance.h:77
void setParameter(std::string, float) override
Definition: DSSIPluginInstance.cpp:201
int getBufferSize() const override
Definition: DSSIPluginInstance.h:74
static int requestNonRTThread(LADSPA_Handle instance, void(*runFunction)(LADSPA_Handle))
Definition: DSSIPluginInstance.cpp:1297
std::string getDescription() const override
Definition: DSSIPluginInstance.cpp:122
ProgramList getPrograms() const override
Definition: DSSIPluginInstance.cpp:541
std::string getCurrentProgram() const override
Definition: DSSIPluginInstance.cpp:606
void checkProgramCache() const
Definition: DSSIPluginInstance.cpp:508
void instantiate(sv_samplerate_t sampleRate)
Definition: DSSIPluginInstance.cpp:459
Definition: DSSIPluginFactory.h:33
Definition: Thread.h:24
static RealTimePluginFactory * instance(QString pluginType)
Definition: RealTimePluginFactory.cpp:43
void setBypassed(bool bypassed) override
Definition: DSSIPluginInstance.h:90
std::vector< std::pair< int, LADSPA_Data * > > m_controlPortsIn
Definition: DSSIPluginInstance.h:148
float getParameterDefault(int parameter) const override
Definition: DSSIPluginInstance.cpp:838
void setPortValueFromController(int portNumber, int controlValue)
Definition: DSSIPluginInstance.cpp:782
void runGrouped(const RealTime &)
Definition: DSSIPluginInstance.cpp:1154
float getParameterValue(int parameter) const override
Definition: DSSIPluginInstance.cpp:828
std::string getCopyright() const override
Definition: DSSIPluginInstance.cpp:140
std::string getIdentifier() const override
Definition: DSSIPluginInstance.cpp:110
static size_t m_groupLocalEventBufferCount
Definition: DSSIPluginInstance.h:199
void discardEvents() override
Definition: DSSIPluginInstance.cpp:310
void sendEvent(const RealTime &eventTime, const void *event) override
Definition: DSSIPluginInstance.cpp:916
virtual void detachFromGroup()
Definition: DSSIPluginInstance.cpp:359
std::vector< int > m_audioPortsIn
Definition: DSSIPluginInstance.h:155
RealTime m_lastEventSendTime
Definition: DSSIPluginInstance.h:190
std::map< QString, PluginSet > GroupMap
Definition: DSSIPluginInstance.h:196
int getAudioInputCount() const override
Definition: DSSIPluginInstance.h:75
void initialiseGroupMembership()
Definition: DSSIPluginInstance.cpp:367
std::set< DSSIPluginInstance * > PluginSet
Definition: DSSIPluginInstance.h:195
LADSPA_Handle m_instanceHandle
Definition: DSSIPluginInstance.h:145
DSSIPluginInstance(RealTimePluginFactory *factory, int client, QString identifier, int position, sv_samplerate_t sampleRate, int blockSize, int idealChannelCount, const DSSI_Descriptor *descriptor)
Definition: DSSIPluginInstance.cpp:56
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
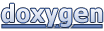