svcore
1.9
|
RingBuffer implements a lock-free ring buffer for one writer and N readers, that is to be used to store a sample type T. More...
#include <RingBuffer.h>
Public Member Functions | |
RingBuffer (int n) | |
Create a ring buffer with room to write n samples. More... | |
virtual | ~RingBuffer () |
int | getSize () const |
Return the total capacity of the ring buffer in samples. More... | |
RingBuffer< T, N > | resized (int newSize) const |
Return a new ring buffer of the given size, containing the same data as this one as perceived by reader 0 of this buffer. More... | |
void | reset () |
Reset read and write pointers, thus emptying the buffer. More... | |
int | getReadSpace (int R=0) const |
Return the amount of data available for reading by reader R, in samples. More... | |
int | getWriteSpace () const |
Return the amount of space available for writing, in samples. More... | |
int | read (T *destination, int n, int R=0) |
Read n samples from the buffer, for reader R. More... | |
int | readAdding (T *destination, int n, int R=0) |
Read n samples from the buffer, for reader R, adding them to the destination. More... | |
T | readOne (int R=0) |
Read one sample from the buffer, for reader R. More... | |
int | peek (T *destination, int n, int R=0) const |
Read n samples from the buffer, if available, for reader R, without advancing the read pointer – i.e. More... | |
T | peekOne (int R=0) const |
Read one sample from the buffer, if available, without advancing the read pointer – i.e. More... | |
int | skip (int n, int R=0) |
Pretend to read n samples from the buffer, for reader R, without actually returning them (i.e. More... | |
int | write (const T *source, int n) |
Write n samples to the buffer. More... | |
int | zero (int n) |
Write n zero-value samples to the buffer. More... | |
RingBuffer (const RingBuffer &)=default | |
RingBuffer & | operator= (const RingBuffer &)=default |
Protected Attributes | |
std::vector< T, breakfastquay::StlAllocator< T > > | m_buffer |
int | m_writer |
std::vector< int > | m_readers |
int | m_size |
Detailed Description
template<typename T, int N = 1>
class RingBuffer< T, N >
RingBuffer implements a lock-free ring buffer for one writer and N readers, that is to be used to store a sample type T.
Definition at line 45 of file RingBuffer.h.
Constructor & Destructor Documentation
RingBuffer< T, N >::RingBuffer | ( | int | n | ) |
Create a ring buffer with room to write n samples.
Note that the internal storage size will actually be n+1 samples, as one element is unavailable for administrative reasons. Since the ring buffer performs best if its size is a power of two, this means n should ideally be some power of two minus one.
Definition at line 168 of file RingBuffer.h.
|
virtual |
Definition at line 180 of file RingBuffer.h.
|
default |
Member Function Documentation
int RingBuffer< T, N >::getSize | ( | ) | const |
Return the total capacity of the ring buffer in samples.
(This is the argument n passed to the constructor.)
Definition at line 189 of file RingBuffer.h.
References RingBuffer< T, N >::m_size.
Referenced by MIDIInput::postEvent(), and OSCQueue::postMessage().
RingBuffer< T, N > RingBuffer< T, N >::resized | ( | int | newSize | ) | const |
Return a new ring buffer of the given size, containing the same data as this one as perceived by reader 0 of this buffer.
If another thread reads from or writes to this buffer during the call, the contents of the new buffer may be incomplete or inconsistent. If this buffer's data will not fit in the new size, the contents are undetermined.
Definition at line 200 of file RingBuffer.h.
References RingBuffer< T, N >::m_buffer, RingBuffer< T, N >::m_readers, RingBuffer< T, N >::m_size, RingBuffer< T, N >::m_writer, and RingBuffer< T, N >::write().
void RingBuffer< T, N >::reset | ( | ) |
Reset read and write pointers, thus emptying the buffer.
Should be called from the write thread.
Definition at line 222 of file RingBuffer.h.
References RingBuffer< T, N >::m_readers, and RingBuffer< T, N >::m_writer.
Referenced by DSSIPluginInstance::clearEvents(), and DSSIPluginInstance::discardEvents().
int RingBuffer< T, N >::getReadSpace | ( | int | R = 0 | ) | const |
Return the amount of data available for reading by reader R, in samples.
Definition at line 236 of file RingBuffer.h.
References RingBuffer< T, N >::m_readers, RingBuffer< T, N >::m_size, and RingBuffer< T, N >::m_writer.
Referenced by MIDIInput::getEventsAvailable(), OSCQueue::getMessagesAvailable(), RingBuffer< T, N >::getWriteSpace(), RingBuffer< T, N >::peek(), RingBuffer< T, N >::read(), RingBuffer< T, N >::readAdding(), DSSIPluginInstance::run(), DSSIPluginInstance::runGrouped(), RingBuffer< T, N >::skip(), and OSCQueue::~OSCQueue().
int RingBuffer< T, N >::getWriteSpace | ( | ) | const |
Return the amount of space available for writing, in samples.
Definition at line 254 of file RingBuffer.h.
References RingBuffer< T, N >::getReadSpace(), RingBuffer< T, N >::m_readers, RingBuffer< T, N >::m_size, and RingBuffer< T, N >::m_writer.
Referenced by MIDIInput::postEvent(), OSCQueue::postMessage(), RingBuffer< T, N >::write(), and RingBuffer< T, N >::zero().
int RingBuffer< T, N >::read | ( | T * | destination, |
int | n, | ||
int | R = 0 |
||
) |
Read n samples from the buffer, for reader R.
If fewer than n are available, the remainder will be zeroed out. Returns the number of samples actually read.
Definition at line 279 of file RingBuffer.h.
References RingBuffer< T, N >::getReadSpace(), RingBuffer< T, N >::m_buffer, RingBuffer< T, N >::m_readers, and RingBuffer< T, N >::m_size.
int RingBuffer< T, N >::readAdding | ( | T * | destination, |
int | n, | ||
int | R = 0 |
||
) |
Read n samples from the buffer, for reader R, adding them to the destination.
If fewer than n are available, the remainder will be left alone. Returns the number of samples actually read.
Definition at line 316 of file RingBuffer.h.
References RingBuffer< T, N >::getReadSpace(), RingBuffer< T, N >::m_buffer, RingBuffer< T, N >::m_readers, and RingBuffer< T, N >::m_size.
T RingBuffer< T, N >::readOne | ( | int | R = 0 | ) |
Read one sample from the buffer, for reader R.
If no sample is available, this will silently return zero. Calling this repeatedly is obviously slower than calling read once, but it may be good enough if you don't want to allocate a buffer to read into.
Definition at line 354 of file RingBuffer.h.
References RingBuffer< T, N >::m_buffer, RingBuffer< T, N >::m_readers, RingBuffer< T, N >::m_size, and RingBuffer< T, N >::m_writer.
Referenced by MIDIInput::readEvent(), OSCQueue::readMessage(), and OSCQueue::~OSCQueue().
int RingBuffer< T, N >::peek | ( | T * | destination, |
int | n, | ||
int | R = 0 |
||
) | const |
Read n samples from the buffer, if available, for reader R, without advancing the read pointer – i.e.
a subsequent read() or skip() will be necessary to empty the buffer. If fewer than n are available, the remainder will be zeroed out. Returns the number of samples actually read.
Definition at line 375 of file RingBuffer.h.
References RingBuffer< T, N >::getReadSpace(), RingBuffer< T, N >::m_buffer, RingBuffer< T, N >::m_readers, and RingBuffer< T, N >::m_size.
T RingBuffer< T, N >::peekOne | ( | int | R = 0 | ) | const |
Read one sample from the buffer, if available, without advancing the read pointer – i.e.
a subsequent read() or skip() will be necessary to empty the buffer. Returns zero if no sample was available.
Definition at line 409 of file RingBuffer.h.
References RingBuffer< T, N >::m_buffer, RingBuffer< T, N >::m_readers, and RingBuffer< T, N >::m_writer.
Referenced by DSSIPluginInstance::run(), and DSSIPluginInstance::runGrouped().
int RingBuffer< T, N >::skip | ( | int | n, |
int | R = 0 |
||
) |
Pretend to read n samples from the buffer, for reader R, without actually returning them (i.e.
discard the next n samples). Returns the number of samples actually available for discarding.
Definition at line 428 of file RingBuffer.h.
References RingBuffer< T, N >::getReadSpace(), RingBuffer< T, N >::m_readers, and RingBuffer< T, N >::m_size.
Referenced by DSSIPluginInstance::run(), and DSSIPluginInstance::runGrouped().
int RingBuffer< T, N >::write | ( | const T * | source, |
int | n | ||
) |
Write n samples to the buffer.
If insufficient space is available, not all samples may actually be written. Returns the number of samples actually written.
Definition at line 449 of file RingBuffer.h.
References RingBuffer< T, N >::getWriteSpace(), RingBuffer< T, N >::m_buffer, RingBuffer< T, N >::m_size, and RingBuffer< T, N >::m_writer.
Referenced by MIDIInput::postEvent(), OSCQueue::postMessage(), RingBuffer< T, N >::resized(), and DSSIPluginInstance::sendEvent().
int RingBuffer< T, N >::zero | ( | int | n | ) |
Write n zero-value samples to the buffer.
If insufficient space is available, not all zeros may actually be written. Returns the number of zeroes actually written.
Definition at line 485 of file RingBuffer.h.
References RingBuffer< T, N >::getWriteSpace(), RingBuffer< T, N >::m_buffer, RingBuffer< T, N >::m_size, and RingBuffer< T, N >::m_writer.
|
default |
Member Data Documentation
|
protected |
Definition at line 161 of file RingBuffer.h.
Referenced by RingBuffer< T, N >::peek(), RingBuffer< T, N >::peekOne(), RingBuffer< T, N >::read(), RingBuffer< T, N >::readAdding(), RingBuffer< T, N >::readOne(), RingBuffer< T, N >::resized(), RingBuffer< T, N >::write(), and RingBuffer< T, N >::zero().
|
protected |
Definition at line 162 of file RingBuffer.h.
Referenced by RingBuffer< T, N >::getReadSpace(), RingBuffer< T, N >::getWriteSpace(), RingBuffer< T, N >::peekOne(), RingBuffer< T, N >::readOne(), RingBuffer< T, N >::reset(), RingBuffer< T, N >::resized(), RingBuffer< T, N >::write(), and RingBuffer< T, N >::zero().
|
protected |
Definition at line 163 of file RingBuffer.h.
Referenced by RingBuffer< T, N >::getReadSpace(), RingBuffer< T, N >::getWriteSpace(), RingBuffer< T, N >::peek(), RingBuffer< T, N >::peekOne(), RingBuffer< T, N >::read(), RingBuffer< T, N >::readAdding(), RingBuffer< T, N >::readOne(), RingBuffer< T, N >::reset(), RingBuffer< T, N >::resized(), and RingBuffer< T, N >::skip().
|
protected |
Definition at line 164 of file RingBuffer.h.
Referenced by RingBuffer< T, N >::getReadSpace(), RingBuffer< T, N >::getSize(), RingBuffer< T, N >::getWriteSpace(), RingBuffer< T, N >::peek(), RingBuffer< T, N >::read(), RingBuffer< T, N >::readAdding(), RingBuffer< T, N >::readOne(), RingBuffer< T, N >::resized(), RingBuffer< T, N >::skip(), RingBuffer< T, N >::write(), and RingBuffer< T, N >::zero().
The documentation for this class was generated from the following file:
Generated by
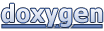