svcore
1.9
|
MIDIInput.h
Go to the documentation of this file.
Definition: MIDIInput.h:28
Definition: MIDIEvent.h:119
void eventsAvailable()
A trivial interface for things that permit retrieving "the current frame".
Definition: FrameTimer.h:27
int getReadSpace(int R=0) const
Return the amount of data available for reading by reader R, in samples.
Definition: RingBuffer.h:236
static void staticCallback(double, std::vector< unsigned char > *, void *)
Definition: MIDIInput.cpp:80
void callback(double, std::vector< unsigned char > *)
Definition: MIDIInput.cpp:87
Generated by
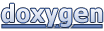