svcore
1.9
|
MIDIEvent.h
Go to the documentation of this file.
static const MIDIByte MIDI_CHANNEL_PREFIX_OR_PORT
Definition: MIDIEvent.h:89
static const MIDIByte MIDI_CONTROLLER_FILTER
Definition: MIDIEvent.h:105
static const MIDIByte MIDI_CONTROLLER_RPN_2
Definition: MIDIEvent.h:111
static const MIDIByte MIDI_COPYRIGHT_NOTICE
Definition: MIDIEvent.h:82
static const MIDIByte MIDI_CONTROLLER_RESET
Definition: MIDIEvent.h:113
static const MIDIByte MIDI_CHANNEL_PREFIX
Definition: MIDIEvent.h:88
static const MIDIByte MIDI_MMC_RECORD_STROBE
Definition: MIDIEvent.h:73
static const MIDIByte MIDI_CHANNEL_NUM_MASK
Definition: MIDIEvent.h:41
static const MIDIByte MIDI_CONTROLLER_MODULATION
Definition: MIDIEvent.h:99
static const MIDIByte MIDI_TC_QUARTER_FRAME
Definition: MIDIEvent.h:52
static const char *const MIDI_TRACK_HEADER
Definition: MIDIEvent.h:37
static const MIDIByte MIDI_CONTROLLER_NRPN_2
Definition: MIDIEvent.h:109
static const MIDIByte MIDI_MMC_DEFERRED_PLAY
Definition: MIDIEvent.h:70
static const MIDIByte MIDI_CONTROLLER_ATTACK
Definition: MIDIEvent.h:104
Definition: MIDIEvent.h:119
static const MIDIByte MIDI_SELECT_CHNL_MODE
Definition: MIDIEvent.h:50
static const MIDIByte MIDI_SEQUENCE_NUMBER
Definition: MIDIEvent.h:80
Definition: MIDIEvent.h:34
static const MIDIByte MIDI_CONTROLLER_BANK_LSB
Definition: MIDIEvent.h:98
static const MIDIByte MIDI_MMC_RECORD_PAUSE
Definition: MIDIEvent.h:75
MIDIEvent(unsigned long deltaTime, MIDIByte eventCode, MIDIByte metaEventCode, const std::string &metaMessage)
Definition: MIDIEvent.h:140
static const MIDIByte MIDI_PERCUSSION_CHANNEL
Definition: MIDIEvent.h:116
static const MIDIByte MIDI_CONTROLLER_NRPN_1
Definition: MIDIEvent.h:108
static const MIDIByte MIDI_SYSEX_RT_COMMAND
Definition: MIDIEvent.h:66
static const MIDIByte MIDI_FILE_META_EVENT
Definition: MIDIEvent.h:79
MIDIEvent(unsigned long deltaTime, MIDIByte eventCode, const std::string &sysEx)
Definition: MIDIEvent.h:153
static const MIDIByte MIDI_CONTROLLER_ALL_NOTES_OFF
Definition: MIDIEvent.h:115
static const MIDIByte MIDI_CONTROLLER_RPN_1
Definition: MIDIEvent.h:110
static const MIDIByte MIDI_INSTRUMENT_NAME
Definition: MIDIEvent.h:84
static const MIDIByte MIDI_CONTROLLER_RESONANCE
Definition: MIDIEvent.h:102
static const MIDIByte MIDI_SEQUENCER_SPECIFIC
Definition: MIDIEvent.h:95
static const MIDIByte MIDI_STATUS_BYTE_MASK
Definition: MIDIEvent.h:39
static const MIDIByte MIDI_CONTROLLER_SOUNDS_OFF
Definition: MIDIEvent.h:112
static const MIDIByte MIDI_ACTIVE_SENSING
Definition: MIDIEvent.h:61
Definition: MIDIEvent.h:212
static const MIDIByte MIDI_MMC_RECORD_EXIT
Definition: MIDIEvent.h:74
static const MIDIByte MIDI_CONTROLLER_RELEASE
Definition: MIDIEvent.h:103
static const MIDIByte MIDI_MMC_FAST_FORWARD
Definition: MIDIEvent.h:71
static const MIDIByte MIDI_CONTROLLER_REVERB
Definition: MIDIEvent.h:106
static const MIDIByte MIDI_POLY_AFTERTOUCH
Definition: MIDIEvent.h:45
static const MIDIByte MIDI_SYSTEM_EXCLUSIVE
Definition: MIDIEvent.h:51
static const MIDIByte MIDI_CONTROLLER_LOCAL
Definition: MIDIEvent.h:114
static const MIDIByte MIDI_SYSEX_RT_RESPONSE
Definition: MIDIEvent.h:67
bool operator()(const MIDIEvent *mE1, const MIDIEvent *mE2) const
Definition: MIDIEvent.h:217
Definition: MIDIEvent.h:221
static const MIDIByte MIDI_TIME_SIGNATURE
Definition: MIDIEvent.h:93
static const MIDIByte MIDI_SONG_POSITION_PTR
Definition: MIDIEvent.h:53
static const MIDIByte MIDI_SYSEX_NONCOMMERCIAL
Definition: MIDIEvent.h:63
static const MIDIByte MIDI_MESSAGE_TYPE_MASK
Definition: MIDIEvent.h:40
static const MIDIByte MIDI_CONTROLLER_VOLUME
Definition: MIDIEvent.h:97
MIDIEvent(unsigned long deltaTime, int eventCode, int data1=0, int data2=0)
Definition: MIDIEvent.h:122
static const MIDIByte MIDI_CONTROLLER_SUSTAIN
Definition: MIDIEvent.h:101
bool operator()(const MIDIEvent &mE1, const MIDIEvent &mE2) const
Definition: MIDIEvent.h:214
static const MIDIByte MIDI_END_OF_EXCLUSIVE
Definition: MIDIEvent.h:56
static const MIDIByte MIDI_CHNL_AFTERTOUCH
Definition: MIDIEvent.h:48
static const MIDIByte MIDI_CONTROLLER_PAN
Definition: MIDIEvent.h:100
static const MIDIByte MIDI_CONTROLLER_BANK_MSB
Definition: MIDIEvent.h:96
static const MIDIByte MIDI_CONTROLLER_CHORUS
Definition: MIDIEvent.h:107
Generated by
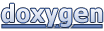