svcore
1.9
|
#include <MIDIEvent.h>
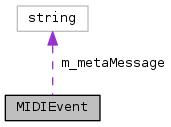
Public Member Functions | |
MIDIEvent (unsigned long deltaTime, int eventCode, int data1=0, int data2=0) | |
MIDIEvent (unsigned long deltaTime, MIDIByte eventCode, MIDIByte metaEventCode, const std::string &metaMessage) | |
MIDIEvent (unsigned long deltaTime, MIDIByte eventCode, const std::string &sysEx) | |
MIDIEvent (const MIDIEvent &)=default | |
MIDIEvent & | operator= (const MIDIEvent &)=default |
~MIDIEvent () | |
void | setTime (const unsigned long &time) |
void | setDuration (const unsigned long &duration) |
unsigned long | addTime (const unsigned long &time) |
MIDIByte | getMessageType () const |
MIDIByte | getChannelNumber () const |
unsigned long | getTime () const |
unsigned long | getDuration () const |
MIDIByte | getPitch () const |
MIDIByte | getVelocity () const |
MIDIByte | getData1 () const |
MIDIByte | getData2 () const |
MIDIByte | getEventCode () const |
bool | isMeta () const |
MIDIByte | getMetaEventCode () const |
std::string | getMetaMessage () const |
void | setMetaMessage (const std::string &meta) |
Private Attributes | |
unsigned long | m_deltaTime |
unsigned long | m_duration |
MIDIByte | m_eventCode |
MIDIByte | m_data1 |
MIDIByte | m_data2 |
MIDIByte | m_metaEventCode |
std::string | m_metaMessage |
Friends | |
bool | operator< (const MIDIEvent &a, const MIDIEvent &b) |
Detailed Description
Definition at line 119 of file MIDIEvent.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 122 of file MIDIEvent.h.
|
inline |
Definition at line 140 of file MIDIEvent.h.
|
inline |
Definition at line 153 of file MIDIEvent.h.
|
default |
|
inline |
Definition at line 168 of file MIDIEvent.h.
Member Function Documentation
|
inline |
Definition at line 170 of file MIDIEvent.h.
|
inline |
Definition at line 171 of file MIDIEvent.h.
|
inline |
Definition at line 172 of file MIDIEvent.h.
|
inline |
Definition at line 177 of file MIDIEvent.h.
References MIDIConstants::MIDI_MESSAGE_TYPE_MASK.
|
inline |
Definition at line 180 of file MIDIEvent.h.
References MIDIConstants::MIDI_CHANNEL_NUM_MASK.
Referenced by MIDIFileReader::parseTrack().
|
inline |
Definition at line 183 of file MIDIEvent.h.
Referenced by MIDIEventCmp::operator()().
|
inline |
Definition at line 184 of file MIDIEvent.h.
|
inline |
Definition at line 186 of file MIDIEvent.h.
|
inline |
Definition at line 187 of file MIDIEvent.h.
|
inline |
Definition at line 188 of file MIDIEvent.h.
|
inline |
Definition at line 189 of file MIDIEvent.h.
|
inline |
Definition at line 190 of file MIDIEvent.h.
|
inline |
Definition at line 192 of file MIDIEvent.h.
References MIDIConstants::MIDI_FILE_META_EVENT.
|
inline |
Definition at line 194 of file MIDIEvent.h.
|
inline |
Definition at line 195 of file MIDIEvent.h.
|
inline |
Definition at line 196 of file MIDIEvent.h.
Friends And Related Function Documentation
Member Data Documentation
|
private |
Definition at line 201 of file MIDIEvent.h.
|
private |
Definition at line 202 of file MIDIEvent.h.
|
private |
Definition at line 203 of file MIDIEvent.h.
|
private |
Definition at line 204 of file MIDIEvent.h.
|
private |
Definition at line 205 of file MIDIEvent.h.
|
private |
Definition at line 206 of file MIDIEvent.h.
|
private |
Definition at line 207 of file MIDIEvent.h.
The documentation for this class was generated from the following file:
Generated by
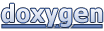