svcore
1.9
|
MIDIFileReader.h
Go to the documentation of this file.
80 MIDI_SINGLE_TRACK_FILE = 0x00,
81 MIDI_SIMULTANEOUS_TRACK_FILE = 0x01,
82 MIDI_SEQUENTIAL_TRACK_FILE = 0x02,
83 MIDI_FILE_BAD_FORMAT = 0xFF
std::set< unsigned int > m_percussionTracks
Definition: MIDIFileReader.h:124
Definition: MIDIFileReader.h:58
std::map< unsigned long, TempoChange > TempoMap
Definition: MIDIFileReader.h:77
Definition: ProgressReporter.h:22
virtual ~MIDIFileImportPreferenceAcquirer()
Definition: MIDIFileReader.h:48
Definition: MIDIEvent.h:119
Definition: DataFileReader.h:24
MIDIFileImportPreferenceAcquirer * m_acquirer
Definition: MIDIFileReader.h:134
virtual TrackPreference getTrackImportPreference(QStringList trackNames, bool haveSomePercussion, QString &singleTrack) const =0
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
sv_samplerate_t m_mainModelSampleRate
Definition: MIDIFileReader.h:132
virtual void showError(QString error)=0
std::set< unsigned int > m_loadableTracks
Definition: MIDIFileReader.h:123
std::map< unsigned int, MIDITrack > MIDIComposition
Definition: MIDIFileReader.h:75
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
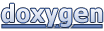