svcore
1.9
|
MIDIInput.cpp
Go to the documentation of this file.
119 SVCERR << "ERROR: MIDIInput::postEvent: MIDI event queue is full and not clearing -- abandoning incoming event" << endl;
122 SVCERR << "WARNING: MIDIInput::postEvent: MIDI event queue (capacity " << m_buffer.getSize() << ") is full!" << endl;
Definition: MIDIInput.h:28
virtual sv_frame_t getFrame() const =0
int getSize() const
Return the total capacity of the ring buffer in samples.
Definition: RingBuffer.h:189
Definition: MIDIEvent.h:119
void eventsAvailable()
A trivial interface for things that permit retrieving "the current frame".
Definition: FrameTimer.h:27
static void staticCallback(double, std::vector< unsigned char > *, void *)
Definition: MIDIInput.cpp:80
int getWriteSpace() const
Return the amount of space available for writing, in samples.
Definition: RingBuffer.h:254
void callback(double, std::vector< unsigned char > *)
Definition: MIDIInput.cpp:87
Generated by
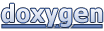