svcore
1.9
|
DSSIPluginInstance.cpp
Go to the documentation of this file.
52 Scavenger<ScavengerArrayWrapper<snd_seq_event_t *> > DSSIPluginInstance::m_bufferScavenger(2, 10);
53 std::map<LADSPA_Handle, std::set<DSSIPluginInstance::NonRTPluginThread *> > DSSIPluginInstance::m_threads;
278 SVDEBUG << "DSSIPluginInstance::getLatency(): m_latencyPort " << m_latencyPort << ", m_run " << m_run << endl;
534 SVDEBUG << "DSSIPluginInstance::checkProgramCache: have " << m_cachedPrograms.size() << " programs" << endl;
643 SVDEBUG << "DSSIPluginInstance::selectProgram(" << program << "): found at bank " << bankNo << ", program " << programNo << endl;
659 SVDEBUG << "DSSIPluginInstance::selectProgram(" << program << "): made select_program(" << bankNo << "," << programNo << ") call" << endl;
688 SVDEBUG << "DSSIPluginInstance::activate: setting port " << m_controlPortsIn[i].first << " to " << m_backupControlPortsIn[i] << endl;
738 SVDEBUG << "DSSIPluginInstance::connectPorts: set control port " << i << " to default value " << defaultValue << endl;
909 cerr << "DSSIPluginInstance::configure: warning: configure returned message: \"" << qm << "\"" << endl;
920 SVDEBUG << "DSSIPluginInstance::sendEvent: last was " << m_lastEventSendTime << " (valid " << m_haveLastEventSendTime << "), this is " << eventTime << endl;
1056 SVDEBUG << "DSSIPluginInstance::run: evTime " << evTime << ", blockTime " << blockTime << ", frameOffset " << frameOffset
1058 cerr << "Type: " << int(ev->type) << ", pitch: " << int(ev->data.note.note) << ", velocity: " << int(ev->data.note.velocity) << endl;
1091 SVDEBUG << "DSSIPluginInstance::run: making select_program(" << bank << "," << program << ") call" << endl;
1098 SVDEBUG << "DSSIPluginInstance::run: made select_program(" << bank << "," << program << ") call" << endl;
1167 SVDEBUG << "DSSIPluginInstance::runGrouped(" << blockTime << "): this is " << this << "; " << s.size() << " elements in m_groupMap[" << m_identifier << "]" << endl;
1175 SVDEBUG << "DSSIPluginInstance::runGrouped(" << blockTime << "): plugin " << instance << " has already been run" << endl;
1184 SVDEBUG << "DSSIPluginInstance::runGrouped(" << blockTime << "): already run, returning" << endl;
1190 SVDEBUG << "DSSIPluginInstance::runGrouped(" << blockTime << "): I'm the first, running" << endl;
1208 SVDEBUG << "DSSIPluginInstance::runGrouped(" << blockTime << "): running " << instance << endl;
1234 SVDEBUG << "DSSIPluginInstance::runGrouped: evTime " << evTime << ", frameOffset " << frameOffset
T peekOne(int R=0) const
Read one sample from the buffer, if available, without advancing the read pointer – i...
Definition: RingBuffer.h:409
std::vector< LADSPA_Data > m_backupControlPortsIn
Definition: DSSIPluginInstance.h:151
std::vector< std::pair< int, LADSPA_Data * > > m_controlPortsOut
Definition: DSSIPluginInstance.h:149
static int requestMidiSend(LADSPA_Handle instance, unsigned char ports, unsigned char channels)
Definition: DSSIPluginInstance.cpp:1267
std::string configure(std::string key, std::string value) override
Definition: DSSIPluginInstance.cpp:866
static std::map< LADSPA_Handle, std::set< NonRTPluginThread * > > m_threads
Definition: DSSIPluginInstance.h:220
int getPortDisplayHint(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:317
static QString RESERVED_PROJECT_DIRECTORY_KEY
Definition: PluginIdentifier.h:47
Definition: RealTimePluginFactory.h:50
float getControlOutputValue(int n) const override
Definition: DSSIPluginInstance.cpp:821
static void midiSend(LADSPA_Handle instance, snd_seq_event_t *events, unsigned long eventCount)
Definition: DSSIPluginInstance.cpp:1278
std::map< int, int > m_controllerMap
Definition: DSSIPluginInstance.h:153
int getPluginVersion() const override
Definition: DSSIPluginInstance.cpp:134
Definition: DSSIPluginInstance.h:39
bool handleController(snd_seq_event_t *ev)
Definition: DSSIPluginInstance.cpp:962
A very simple class that facilitates running things like plugins without locking, by collecting unwan...
Definition: Scavenger.h:44
void setParameterValue(int parameter, float value) override
Definition: DSSIPluginInstance.cpp:758
static snd_seq_event_t ** m_groupLocalEventBuffers
Definition: DSSIPluginInstance.h:198
ConfigurationPairMap m_configurationData
Definition: RealTimePluginInstance.h:151
std::string getName() const override
Definition: DSSIPluginInstance.cpp:116
RingBuffer< snd_seq_event_t > m_eventBuffer
Definition: DSSIPluginInstance.h:173
int getParameterDisplayHint(int parameter) const override
Definition: DSSIPluginInstance.cpp:852
std::string getMaker() const override
Definition: DSSIPluginInstance.cpp:128
void selectProgram(std::string program) override
Definition: DSSIPluginInstance.cpp:612
void selectProgramAux(std::string program, bool backupPortValues)
Definition: DSSIPluginInstance.cpp:618
float getPortDefault(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:199
void run(const RealTime &, int count=0) override
Run for one block, starting at the given time.
Definition: DSSIPluginInstance.cpp:992
std::vector< ProgramDescriptor > m_cachedPrograms
Definition: DSSIPluginInstance.h:170
int getParameterCount() const override
Definition: DSSIPluginInstance.cpp:752
std::vector< int > m_audioPortsOut
Definition: DSSIPluginInstance.h:156
void setIdealChannelCount(int channels) override
Definition: DSSIPluginInstance.cpp:316
bool m_haveLastEventSendTime
Definition: DSSIPluginInstance.h:191
static sv_samplerate_t m_sampleRate
Definition: RealTimePluginFactory.h:112
std::string getProgram(int bank, int program) const override
Definition: DSSIPluginInstance.cpp:562
float getPortMaximum(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:176
const DSSI_Descriptor * m_descriptor
Definition: DSSIPluginInstance.h:146
float getParameter(std::string) const override
Definition: DSSIPluginInstance.cpp:179
sv_frame_t getLatency() override
Definition: DSSIPluginInstance.cpp:273
float getPortQuantization(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:303
static Scavenger< ScavengerArrayWrapper< snd_seq_event_t * > > m_bufferScavenger
Definition: DSSIPluginInstance.h:201
virtual ~DSSIPluginInstance()
Definition: DSSIPluginInstance.cpp:404
ParameterList getParameterDescriptors() const override
Definition: DSSIPluginInstance.cpp:146
void run() override
Definition: DSSIPluginInstance.cpp:1288
void setParameter(std::string, float) override
Definition: DSSIPluginInstance.cpp:201
static int requestNonRTThread(LADSPA_Handle instance, void(*runFunction)(LADSPA_Handle))
Definition: DSSIPluginInstance.cpp:1297
std::string getDescription() const override
Definition: DSSIPluginInstance.cpp:122
int getReadSpace(int R=0) const
Return the amount of data available for reading by reader R, in samples.
Definition: RingBuffer.h:236
ProgramList getPrograms() const override
Definition: DSSIPluginInstance.cpp:541
static sv_frame_t realTime2Frame(const RealTime &r, sv_samplerate_t sampleRate)
Convert a RealTime into a sample frame at the given sample rate.
Definition: RealTimeSV.cpp:490
Definition: LADSPAPluginFactory.h:36
std::string getCurrentProgram() const override
Definition: DSSIPluginInstance.cpp:606
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
void checkProgramCache() const
Definition: DSSIPluginInstance.cpp:508
void instantiate(sv_samplerate_t sampleRate)
Definition: DSSIPluginInstance.cpp:459
RealTimePluginFactory * m_factory
Definition: RealTimePluginInstance.h:148
static RealTimePluginFactory * instance(QString pluginType)
Definition: RealTimePluginFactory.cpp:43
std::ostream & operator<<(std::ostream &ostr, const TypedId< T > &id)
Definition: ById.h:140
std::vector< std::pair< int, LADSPA_Data * > > m_controlPortsIn
Definition: DSSIPluginInstance.h:148
float getParameterDefault(int parameter) const override
Definition: DSSIPluginInstance.cpp:838
void setPortValueFromController(int portNumber, int controlValue)
Definition: DSSIPluginInstance.cpp:782
void runGrouped(const RealTime &)
Definition: DSSIPluginInstance.cpp:1154
float getParameterValue(int parameter) const override
Definition: DSSIPluginInstance.cpp:828
int skip(int n, int R=0)
Pretend to read n samples from the buffer, for reader R, without actually returning them (i...
Definition: RingBuffer.h:428
std::string getCopyright() const override
Definition: DSSIPluginInstance.cpp:140
std::string getIdentifier() const override
Definition: DSSIPluginInstance.cpp:110
void claim(T *t)
Call from an RT thread etc., to pass ownership of t to us.
Definition: Scavenger.h:125
static size_t m_groupLocalEventBufferCount
Definition: DSSIPluginInstance.h:199
void discardEvents() override
Definition: DSSIPluginInstance.cpp:310
void sendEvent(const RealTime &eventTime, const void *event) override
Definition: DSSIPluginInstance.cpp:916
virtual void detachFromGroup()
Definition: DSSIPluginInstance.cpp:359
std::vector< int > m_audioPortsIn
Definition: DSSIPluginInstance.h:155
RealTime m_lastEventSendTime
Definition: DSSIPluginInstance.h:190
float getPortMinimum(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:149
std::map< QString, PluginSet > GroupMap
Definition: DSSIPluginInstance.h:196
int getAudioInputCount() const override
Definition: DSSIPluginInstance.h:75
void initialiseGroupMembership()
Definition: DSSIPluginInstance.cpp:367
std::set< DSSIPluginInstance * > PluginSet
Definition: DSSIPluginInstance.h:195
LADSPA_Handle m_instanceHandle
Definition: DSSIPluginInstance.h:145
DSSIPluginInstance(RealTimePluginFactory *factory, int client, QString identifier, int position, sv_samplerate_t sampleRate, int blockSize, int idealChannelCount, const DSSI_Descriptor *descriptor)
Definition: DSSIPluginInstance.cpp:56
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
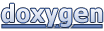