svcore
1.9
|
RealTimePluginInstance.h
Go to the documentation of this file.
111 virtual std::string getProgram(int /* bank */, int /* program */) const { return std::string(); }
121 virtual std::string configure(std::string /* key */, std::string /* value */) { return std::string(); }
135 virtual void setIdealChannelCount(int channels) = 0; // must also silence(); may also re-instantiate
virtual std::string configure(std::string, std::string)
Definition: RealTimePluginInstance.h:121
Definition: RealTimePluginFactory.h:50
ConfigurationPairMap m_configurationData
Definition: RealTimePluginInstance.h:151
Definition: RealTimePluginInstance.h:69
std::map< std::string, std::string > ConfigurationPairMap
Definition: RealTimePluginInstance.h:139
Definition: AudioPlaySource.h:23
virtual void discardEvents()
Definition: RealTimePluginInstance.h:134
RealTimePluginFactory * m_factory
Definition: RealTimePluginInstance.h:148
virtual std::string getProgram(int, int) const
Definition: RealTimePluginInstance.h:111
virtual ConfigurationPairMap getConfigurePairs()
Definition: RealTimePluginInstance.h:140
RealTimePluginInstance is an interface that an audio process can use to refer to an instance of a plu...
Definition: RealTimePluginInstance.h:62
std::string getType() const override
Definition: RealTimePluginInstance.h:137
virtual void clearEvents()
Definition: RealTimePluginInstance.h:125
virtual void sendEvent(const RealTime &, const void *)
Definition: RealTimePluginInstance.h:123
RealTimePluginInstance(RealTimePluginFactory *factory, QString identifier)
Definition: RealTimePluginInstance.h:145
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
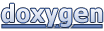