svcore
1.9
|
LADSPAPluginInstance.cpp
Go to the documentation of this file.
float getControlOutputValue(int n) const override
Definition: LADSPAPluginInstance.cpp:501
float getParameterValue(int parameter) const override
Definition: LADSPAPluginInstance.cpp:508
int getPluginVersion() const override
Definition: LADSPAPluginInstance.cpp:110
sample_t ** m_inputBuffers
Definition: LADSPAPluginInstance.h:123
std::vector< std::pair< int, LADSPA_Data * > > m_controlPortsOut
Definition: LADSPAPluginInstance.h:117
std::string getDescription() const override
Definition: LADSPAPluginInstance.cpp:98
int getParameterCount() const override
Definition: LADSPAPluginInstance.cpp:475
virtual ~LADSPAPluginInstance()
Definition: LADSPAPluginInstance.cpp:342
int getPortDisplayHint(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:317
Definition: RealTimePluginFactory.h:50
std::vector< LADSPA_Handle > m_instanceHandles
Definition: LADSPAPluginInstance.h:112
sv_samplerate_t m_sampleRate
Definition: LADSPAPluginInstance.h:126
LADSPAPluginInstance(RealTimePluginFactory *factory, int client, QString identifier, int position, sv_samplerate_t sampleRate, int blockSize, int idealChannelCount, const LADSPA_Descriptor *descriptor)
Definition: LADSPAPluginInstance.cpp:36
std::string getIdentifier() const override
Definition: LADSPAPluginInstance.cpp:86
void setParameterValue(int parameter, float value) override
Definition: LADSPAPluginInstance.cpp:481
float getPortDefault(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:199
int getAudioInputCount() const override
Definition: LADSPAPluginInstance.h:67
int getParameterDisplayHint(int parameter) const override
Definition: LADSPAPluginInstance.cpp:528
static sv_samplerate_t m_sampleRate
Definition: RealTimePluginFactory.h:112
float getPortMaximum(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:176
sv_frame_t getLatency() override
Definition: LADSPAPluginInstance.cpp:293
float getPortQuantization(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:303
void instantiate(sv_samplerate_t sampleRate)
Definition: LADSPAPluginInstance.cpp:381
float getParameterDefault(int parameter) const override
Definition: LADSPAPluginInstance.cpp:515
ParameterList getParameterDescriptors() const override
Definition: LADSPAPluginInstance.cpp:122
Definition: LADSPAPluginFactory.h:36
std::string getCopyright() const override
Definition: LADSPAPluginInstance.cpp:116
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
void setIdealChannelCount(int channels) override
Definition: LADSPAPluginInstance.cpp:319
void init(int idealChannelCount=0)
Definition: LADSPAPluginInstance.cpp:221
std::vector< int > m_audioPortsOut
Definition: LADSPAPluginInstance.h:120
RealTimePluginFactory * m_factory
Definition: RealTimePluginInstance.h:148
std::vector< int > m_audioPortsIn
Definition: LADSPAPluginInstance.h:119
std::string getName() const override
Definition: LADSPAPluginInstance.cpp:92
void run(const RealTime &rt, int count=0) override
Run for one block, starting at the given time.
Definition: LADSPAPluginInstance.cpp:541
sample_t ** m_outputBuffers
Definition: LADSPAPluginInstance.h:124
std::string getMaker() const override
Definition: LADSPAPluginInstance.cpp:104
float getParameter(std::string) const override
Definition: LADSPAPluginInstance.cpp:194
const LADSPA_Descriptor * m_descriptor
Definition: LADSPAPluginInstance.h:114
std::vector< std::pair< int, LADSPA_Data * > > m_controlPortsIn
Definition: LADSPAPluginInstance.h:116
float getPortMinimum(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:149
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
void setParameter(std::string, float) override
Definition: LADSPAPluginInstance.cpp:206
Generated by
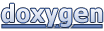