svcore
1.9
|
FFTModel.h
Go to the documentation of this file.
QVector< QVector< QString > > toStringExportRows(DataExportOptions, sv_frame_t, sv_frame_t) const override
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: FFTModel.h:108
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: FFTModel.h:78
bool isOK() const override
Return true if the model was constructed successfully.
Definition: FFTModel.cpp:93
std::pair< sv_frame_t, sv_frame_t > getSourceSampleRange(int column) const
Definition: FFTModel.h:187
void getValuesAt(int x, int y, float &real, float &imaginary) const
Definition: FFTModel.cpp:211
float getMagnitudeAt(int x, int y) const
!! review which of these are ever actually called
Definition: FFTModel.cpp:182
floatvec_t getSourceData(std::pair< sv_frame_t, sv_frame_t >) const
Definition: FFTModel.cpp:287
float getMaximumLevel() const override
Return the maximum permissible value in each bin.
Definition: FFTModel.h:86
int getResolution() const override
Return the number of sample frames covered by each column of bins.
Definition: FFTModel.h:81
float getMinimumLevel() const override
Return the minimum permissible value in each bin.
Definition: FFTModel.h:85
virtual bool estimateStableFrequency(int x, int y, double &frequency)
Calculate an estimated frequency for a stable signal in this bin, using phase unwrapping.
Definition: FFTModel.cpp:424
An implementation of DenseThreeDimensionalModel that makes FFT data derived from a DenseTimeValueMode...
Definition: FFTModel.h:36
bool hasBinValues() const override
Return true if the bins have values as well as names.
Definition: FFTModel.h:90
virtual PeakLocationSet getPeaks(PeakPickType type, int x, int ymin=0, int ymax=0) const
Return locations of peak bins in the range [ymin,ymax].
Definition: FFTModel.cpp:461
QString getBinValueUnit() const override
Obtain the name of the unit of the values returned from getBinValue(), if any.
Definition: FFTModel.h:93
const complexvec_t & getFFTColumn(int column) const
Definition: FFTModel.cpp:382
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
bool getMagnitudesAt(int x, float *values, int minbin=0, int count=0) const
Definition: FFTModel.cpp:224
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
Definition: FFTModel.cpp:108
virtual PeakSet getPeakFrequencies(PeakPickType type, int x, int ymin=0, int ymax=0) const
Return locations and estimated stable frequencies of peak bins.
Definition: FFTModel.cpp:625
QString getBinName(int n) const override
Get the name of a given bin (i.e.
Definition: FFTModel.cpp:148
bool shouldUseLogValueScale() const override
Estimate whether a logarithmic scale might be appropriate for the value scale.
Definition: FFTModel.h:96
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: FFTModel.h:72
float getMaximumMagnitudeAt(int x) const
Definition: FFTModel.cpp:192
Definition: FFTModel.h:145
FFTModel & operator=(const FFTModel &)=delete
int getHeight() const override
Return the number of bins in each column.
Definition: FFTModel.cpp:135
Definition: FFTModel.h:201
Peaks picked using sliding median window.
Definition: FFTModel.h:147
QVector< QString > getStringExportHeaders(DataExportOptions) const override
Return a label for each column that would be written by toStringExportRows.
Definition: FFTModel.h:103
FFTModel(ModelId model, int channel, WindowType windowType, int windowSize, int windowIncrement, int fftSize)
!! threading requirements? !! doubles? since we're not caching much
Definition: FFTModel.cpp:35
floatvec_t getSourceDataUncached(std::pair< sv_frame_t, sv_frame_t >) const
Definition: FFTModel.cpp:334
bool getPhasesAt(int x, float *values, int minbin=0, int count=0) const
Definition: FFTModel.cpp:237
Column getColumn(int x) const override
Get data from the given column of bin values.
Definition: FFTModel.cpp:160
float getValueAt(int x, int y) const override
Get the single data point from the n'th bin of the given column.
Definition: FFTModel.h:69
ColumnOp::Column Column
Definition: DenseThreeDimensionalModel.h:59
std::vector< std::complex< float >, breakfastquay::StlAllocator< std::complex< float > > > complexvec_t
Definition: BaseTypes.h:56
int getPeakPickWindowSize(PeakPickType type, sv_samplerate_t sampleRate, int bin, double &dist) const
Definition: FFTModel.cpp:581
int getWidth() const override
Return the number of columns of bins in the model.
Definition: FFTModel.cpp:126
float getBinValue(int n) const override
Return the value of bin n, if any.
Definition: FFTModel.cpp:154
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: FFTModel.h:75
floatvec_t getSourceSamples(int column) const
Definition: FFTModel.cpp:262
Definition: FFTModel.h:207
Generated by
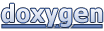