svcore
1.9
|
Simple interface for audio playback. More...
#include <AudioPlaySource.h>
Public Member Functions | |
virtual | ~AudioPlaySource () |
virtual void | play (sv_frame_t startFrame)=0 |
Start playing from the given frame. More... | |
virtual void | stop ()=0 |
Stop playback. More... | |
virtual bool | isPlaying () const =0 |
Return whether playback is currently supposed to be happening. More... | |
virtual sv_frame_t | getCurrentPlayingFrame ()=0 |
Return the frame number that is currently expected to be coming out of the speakers. More... | |
virtual bool | getOutputLevels (float &left, float &right)=0 |
Return the current (or thereabouts) output levels in the range 0.0 -> 1.0, for metering purposes. More... | |
virtual sv_samplerate_t | getSourceSampleRate () const =0 |
Return the sample rate of the source material – any material that wants to play at a different rate will sound wrong. More... | |
virtual sv_samplerate_t | getDeviceSampleRate () const =0 |
Return the sample rate set by the target audio device (or 0 if the target hasn't told us yet). More... | |
virtual int | getTargetBlockSize () const =0 |
Get the block size of the target audio device. More... | |
virtual int | getTargetChannelCount () const =0 |
Get the number of channels of audio that will be provided to the play target. More... | |
virtual void | setAuditioningEffect (std::shared_ptr< Auditionable >)=0 |
Set a plugin or other subclass of Auditionable as an auditioning effect. More... | |
Detailed Description
Simple interface for audio playback.
This should be all that the ViewManager needs to know about to synchronise with playback by sample frame, but it doesn't provide enough to determine what is actually being played or how. See the audioio directory for a concrete subclass.
Definition at line 35 of file AudioPlaySource.h.
Constructor & Destructor Documentation
|
inlinevirtual |
Definition at line 38 of file AudioPlaySource.h.
Member Function Documentation
|
pure virtual |
Start playing from the given frame.
If playback is already under way, reseek to the given frame and continue.
|
pure virtual |
Stop playback.
|
pure virtual |
Return whether playback is currently supposed to be happening.
|
pure virtual |
Return the frame number that is currently expected to be coming out of the speakers.
(i.e. compensating for playback latency.)
|
pure virtual |
Return the current (or thereabouts) output levels in the range 0.0 -> 1.0, for metering purposes.
The values returned are peak values since the last call to this function was made (i.e. calling this function also resets them).
|
pure virtual |
Return the sample rate of the source material – any material that wants to play at a different rate will sound wrong.
Referenced by ModelTransformerFactory::getConfigurationForTransform().
|
pure virtual |
Return the sample rate set by the target audio device (or 0 if the target hasn't told us yet).
If the source and target sample rates differ, resampling will occur.
Note that we don't actually do any processing at the device sample rate. All processing happens at the source sample rate, and then a resampler is applied if necessary at the interface between application and driver layer.
|
pure virtual |
Get the block size of the target audio device.
This may be an estimate or upper bound, if the target has a variable block size; the source should behave itself even if this value turns out to be inaccurate.
Referenced by ModelTransformerFactory::getConfigurationForTransform().
|
pure virtual |
Get the number of channels of audio that will be provided to the play target.
This may be more than the source channel count: for example, a mono source will provide 2 channels after pan.
Referenced by ModelTransformerFactory::getConfigurationForTransform().
|
pure virtual |
Set a plugin or other subclass of Auditionable as an auditioning effect.
The Auditionable is shared with the caller: the expectation is that the caller may continue to modify its parameters etc during auditioning.
The documentation for this class was generated from the following file:
Generated by
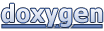