svcore
1.9
|
EventSeries.cpp
Go to the documentation of this file.
Definition: EventSeries.h:182
QString toXmlString(QString indent="", QString extraAttributes="") const
Definition: Event.h:329
bool seamsEqual(const std::vector< Event > &s1, const std::vector< Event > &s2) const
Return true if the two seam map entries contain the same set of events.
Definition: EventSeries.h:327
bool getEventFollowing(const Event &e, Event &following) const
If e is in the series and is not the final event in it, set following to the event immediate followin...
Definition: EventSeries.cpp:489
Event getEventByIndex(int index) const
Return the event at the given numerical index in the series, where 0 = the first event and count()-1 ...
Definition: EventSeries.cpp:547
EventVector getAllEvents() const
Retrieve all events, in their natural order.
Definition: EventSeries.cpp:464
static EventSeries fromEvents(const EventVector &ee)
Definition: EventSeries.cpp:62
sv_frame_t getStartFrame() const
Return the frame of the first event in the series.
Definition: EventSeries.cpp:265
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration, sv_samplerate_t sampleRate, sv_frame_t resolution, Event fillEvent) const
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: EventSeries.cpp:615
Export sparse event-based models as if they were dense models, writing an event at every interval of ...
Definition: DataExportOptions.h:28
Definition: EventSeries.h:181
QVector< QString > getStringExportHeaders(DataExportOptions options, Event::ExportNameOptions) const
Return a label for each column that would be written by toStringExportRows.
Definition: EventSeries.cpp:604
int getIndexForEvent(const Event &e) const
Return the index of the first event in the series that does not compare inferior to the given event...
Definition: EventSeries.cpp:557
void toXml(QTextStream &out, QString indent, QString extraAttributes) const override
Emit to XML as a dataset element.
Definition: EventSeries.cpp:567
QVector< QString > toStringExportRow(DataExportOptions opts, sv_samplerate_t sampleRate) const
Definition: Event.h:421
Definition: Event.h:282
void createSeam(sv_frame_t frame)
Create a seam at the given frame, copying from the prior seam if there is one.
Definition: EventSeries.h:302
EventVector getEventsCovering(sv_frame_t frame) const
Retrieve all events that cover the given frame.
Definition: EventSeries.cpp:420
bool getEventPreceding(const Event &e, Event &preceding) const
If e is in the series and is not the first event in it, set preceding to the event immediate precedin...
Definition: EventSeries.cpp:472
EventVector getEventsSpanning(sv_frame_t frame, sv_frame_t duration) const
Retrieve all events any part of which falls within the range in frames defined by the given frame f a...
Definition: EventSeries.cpp:294
sv_frame_t m_finalDurationlessEventFrame
The frame of the last durationless event we have in the series.
Definition: EventSeries.h:293
sv_frame_t getEndFrame() const
Return the frame plus duration of the event in the series that ends last.
Definition: EventSeries.cpp:273
bool operator==(const EventSeries &other) const
Definition: EventSeries.cpp:55
ExportId getExportId() const
Return the numerical export identifier for this object.
Definition: XmlExportable.cpp:71
bool getNearestEventMatching(sv_frame_t startSearchAt, std::function< bool(const Event &)> predicate, Direction direction, Event &found) const
Return the first event for which the given predicate returns true, searching events with start frames...
Definition: EventSeries.cpp:508
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
EventVector getEventsWithin(sv_frame_t frame, sv_frame_t duration, int overspill=0) const
Retrieve all events falling wholly within the range in frames defined by the given frame f and durati...
Definition: EventSeries.cpp:342
An immutable(-ish) type used for point and event representation in sparse models, as well as for inte...
Definition: Event.h:55
Container storing a series of events, with or without durations, and supporting the ability to query ...
Definition: EventSeries.h:49
EventVector getEventsStartingWithin(sv_frame_t frame, sv_frame_t duration) const
Retrieve all events starting within the range in frames defined by the given frame f and duration d...
Definition: EventSeries.cpp:395
Generated by
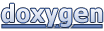