svcore
1.9
|
EventCommands.h
Go to the documentation of this file.
void addCommand(Command *command) override
Stack an arbitrary other command in the same sequence.
Definition: EventCommands.h:131
virtual void add(Event e)=0
Command to add an event to an editable containing events, with undo.
Definition: EventCommands.h:55
ChangeEventsCommand(int editableId, QString name)
Definition: EventCommands.h:118
ChangeEventsCommand * finish()
If any points have been added or deleted, return this command (so the caller can add it to the comman...
Definition: EventCommands.h:138
AddEventCommand(int editableId, const Event &e, QString name)
Definition: EventCommands.h:59
Definition: EventCommands.h:33
An immutable(-ish) type used for point and event representation in sparse models, as well as for inte...
Definition: Event.h:55
Definition: Command.h:26
Definition: Command.h:86
std::shared_ptr< EventEditable > getEditable()
Definition: EventCommands.h:38
RemoveEventCommand(int editableId, const Event &e, QString name)
Definition: EventCommands.h:88
virtual void execute()=0
virtual void addCommand(Command *command, bool executeFirst)
Definition: EventCommands.h:148
Interface for classes that can be modified through these commands.
Definition: EventCommands.h:26
Command to remove an event from an editable containing events, with undo.
Definition: EventCommands.h:84
Command to add or remove a series of events to or from an editable, with undo.
Definition: EventCommands.h:115
Generated by
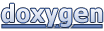