svcore
1.9
|
NoteData.h
Go to the documentation of this file.
static double getFrequencyForPitch(int midiPitch, double centsOffset=0, double concertA=0.0)
Return the frequency at the given MIDI pitch plus centsOffset cents (1/100ths of a semitone)...
Definition: Pitch.cpp:23
Note record used when constructing synthetic events for sonification.
Definition: NoteData.h:25
NoteData(sv_frame_t _start, sv_frame_t _dur, int _mp, int _vel)
Definition: NoteData.h:27
Generated by
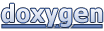