svcore
1.9
|
ColumnOp.h
Go to the documentation of this file.
Class containing static functions for simple operations on data columns, for use by display layers...
Definition: ColumnOp.h:50
static Column applyGain(const Column &in, double gain)
Scale the given column using the given gain multiplier.
Definition: ColumnOp.h:61
static Column applyShift(const Column &in, float offset)
Shift the values in the given column by the given offset.
Definition: ColumnOp.h:72
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
static bool isPeak(const Column &in, int ix)
Determine whether an index points to a local peak.
Definition: ColumnOp.h:88
Generated by
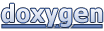