svcore
1.9
|
Class containing static functions for simple operations on data columns, for use by display layers. More...
#include <ColumnOp.h>
Public Types | |
typedef floatvec_t | Column |
Column type. More... | |
Static Public Member Functions | |
static Column | applyGain (const Column &in, double gain) |
Scale the given column using the given gain multiplier. More... | |
static Column | applyShift (const Column &in, float offset) |
Shift the values in the given column by the given offset. More... | |
static Column | fftScale (const Column &in, int fftSize) |
Scale an FFT output downward by half the FFT size. More... | |
static bool | isPeak (const Column &in, int ix) |
Determine whether an index points to a local peak. More... | |
static Column | peakPick (const Column &in) |
Return a column containing only the local peak values (all others zero). More... | |
static Column | normalize (const Column &in, ColumnNormalization n) |
Return a column normalized from the input column according to the given normalization scheme. More... | |
static Column | distribute (const Column &in, int h, const std::vector< double > &binfory, int minbin, bool interpolate) |
Distribute the given column into a target vector of a different size, optionally using linear interpolation. More... | |
Detailed Description
Class containing static functions for simple operations on data columns, for use by display layers.
Definition at line 50 of file ColumnOp.h.
Member Typedef Documentation
typedef floatvec_t ColumnOp::Column |
Column type.
Definition at line 56 of file ColumnOp.h.
Member Function Documentation
Scale the given column using the given gain multiplier.
Definition at line 61 of file ColumnOp.h.
Shift the values in the given column by the given offset.
Definition at line 72 of file ColumnOp.h.
|
static |
Scale an FFT output downward by half the FFT size.
Definition at line 27 of file ColumnOp.cpp.
|
inlinestatic |
Determine whether an index points to a local peak.
Definition at line 88 of file ColumnOp.h.
References in_range_for().
|
static |
Return a column containing only the local peak values (all others zero).
Definition at line 33 of file ColumnOp.cpp.
References in_range_for().
|
static |
Return a column normalized from the input column according to the given normalization scheme.
Note that the sum or max (as appropriate) used for normalisation will be calculated from the absolute values of the column elements, should any of them be negative.
Definition at line 47 of file ColumnOp.cpp.
|
static |
Distribute the given column into a target vector of a different size, optionally using linear interpolation.
The binfory vector contains a mapping from y coordinate (i.e. index into the target vector) to bin (i.e. index into the source column). The source column ("in") may be a partial column; it's assumed to contain enough bins to span the destination range, starting with the bin of index minbin.
Definition at line 116 of file ColumnOp.cpp.
References SVCERR.
The documentation for this class was generated from the following files:
Generated by
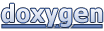