svcore
1.9
|
ColumnOp.cpp
Go to the documentation of this file.
192 SVCERR << "ERROR: bin index out of range in ColumnOp::distribute: by0 = " << by0 << ", by1 = " << by1 << ", sy0 = " << sy0 << ", sy1 = " << sy1 << ", y = " << y << ", binfory[y] = " << binfory[y] << ", minbin = " << minbin << ", bins = " << bins << endl;
static Column normalize(const Column &in, ColumnNormalization n)
Return a column normalized from the input column according to the given normalization scheme...
Definition: ColumnOp.cpp:47
static Column fftScale(const Column &in, int fftSize)
Scale an FFT output downward by half the FFT size.
Definition: ColumnOp.cpp:27
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
static Column distribute(const Column &in, int h, const std::vector< double > &binfory, int minbin, bool interpolate)
Distribute the given column into a target vector of a different size, optionally using linear interpo...
Definition: ColumnOp.cpp:116
static Column peakPick(const Column &in)
Return a column containing only the local peak values (all others zero).
Definition: ColumnOp.cpp:33
Generated by
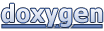