svcore
1.9
|
MP3FileReader.h
Go to the documentation of this file.
Definition: MP3FileReader.h:31
QString getLocation() const override
Return the location of the audio data in the reader (as passed in to the FileSource constructor...
Definition: MP3FileReader.h:80
QString loadTag(void *vtag, const char *name)
Definition: MP3FileReader.cpp:243
enum mad_flow filter(struct mad_stream const *, struct mad_frame *)
Definition: MP3FileReader.cpp:398
Definition: MP3FileReader.h:125
static void getSupportedExtensions(std::set< QString > &extensions)
Definition: MP3FileReader.cpp:614
QString getLocation() const
Return the location filename or URL as passed to the constructor.
Definition: FileSource.cpp:616
Definition: ProgressReporter.h:22
GaplessMode
How the MP3FileReader should handle leading and trailing gaps.
Definition: MP3FileReader.h:41
QString getMaker() const override
Return the "maker" of the work in the audio file, if known.
Definition: MP3FileReader.h:82
Definition: CodedAudioFileReader.h:40
Do not trim any samples.
int getDecodeCompletion() const override
Return a percentage value indicating how far through decoding the audio file we are.
Definition: MP3FileReader.h:90
bool isUpdating() const override
Return true if decoding is still in progress and the frame count may change.
Definition: MP3FileReader.h:92
enum mad_flow accept(struct mad_header const *, struct mad_pcm *)
Definition: MP3FileReader.cpp:487
static enum mad_flow filter_callback(void *, struct mad_stream const *, struct mad_frame *)
Definition: MP3FileReader.cpp:380
std::map< QString, QString > TagMap
Return any tag pairs picked up from the audio file.
Definition: AudioFileReader.h:117
MP3FileReader(FileSource source, DecodeMode decodeMode, CacheMode cacheMode, GaplessMode gaplessMode, sv_samplerate_t targetRate=0, bool normalised=false, ProgressReporter *reporter=0)
Definition: MP3FileReader.cpp:52
Definition: MP3FileReader.h:144
Trim unwanted samples from the start and end of the decoded audio.
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
static enum mad_flow output_callback(void *, struct mad_header const *, struct mad_pcm *)
Definition: MP3FileReader.cpp:478
Definition: Thread.h:24
QString getTitle() const override
Return the title of the work in the audio file, if known.
Definition: MP3FileReader.h:81
static enum mad_flow error_callback(void *, struct mad_stream *, struct mad_frame *)
Definition: MP3FileReader.cpp:585
DecodeThread(MP3FileReader *reader)
Definition: MP3FileReader.h:147
static bool supportsExtension(QString ext)
Definition: MP3FileReader.cpp:620
QString getError() const override
If isOK() is false, return an error string.
Definition: MP3FileReader.h:78
static bool supportsContentType(QString type)
Definition: MP3FileReader.cpp:628
static enum mad_flow input_callback(void *, struct mad_stream *)
Definition: MP3FileReader.cpp:349
Generated by
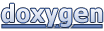