svcore
1.9
|
WavFileWriter.cpp
Go to the documentation of this file.
virtual int getChannelCount() const =0
Return the number of distinct channels for this model.
const SelectionList & getSelections() const
Definition: Selection.cpp:111
void setSelection(const Selection &selection)
Definition: Selection.cpp:117
virtual floatvec_t getData(int channel, sv_frame_t start, sv_frame_t count) const =0
Get the specified set of samples from the given channel of the model in single-precision floating-poi...
A selection object simply represents a range in time, via start and end frame.
Definition: Selection.h:40
Definition: Selection.h:63
sv_frame_t getEndFrame() const
Return the audio frame at the end of the model, i.e.
Definition: Model.h:87
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
WavFileWriter(QString path, sv_samplerate_t sampleRate, int channels, FileWriteMode mode)
Definition: WavFileWriter.cpp:35
bool writeModel(DenseTimeValueModel *source, MultiSelection *selection=0)
Definition: WavFileWriter.cpp:114
Definition: Exceptions.h:69
A class that manages the creation of a temporary file with a given prefix and the renaming of that fi...
Definition: TempWriteFile.h:26
void moveToTarget()
Rename the temporary file to the target filename.
Definition: TempWriteFile.cpp:53
virtual sv_frame_t getStartFrame() const =0
Return the first audio frame spanned by the model.
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
Base class for models containing dense two-dimensional data (value against time). ...
Definition: DenseTimeValueModel.h:30
QString getTemporaryFilename()
Return the name of the temporary file.
Definition: TempWriteFile.cpp:47
bool putInterleavedFrames(const floatvec_t &frames)
As writeSamples, but compatible with WavFileReader api. More expensive.
Definition: WavFileWriter.cpp:204
bool writeSamples(const float *const *samples, sv_frame_t count)
Write samples from raw arrays; count is per-channel.
Definition: WavFileWriter.cpp:176
Generated by
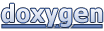