svcore
1.9
|
TempWriteFile.cpp
Go to the documentation of this file.
30 SVCERR << "TempWriteFile: Failed to create temporary file in directory of " << m_target << ": " << temp.errorString() << endl;
62 SVCERR << "TempWriteFile: WARNING: Failed to remove existing target file " << m_target << " prior to moving temporary file " << m_temp << " to it" << endl;
67 SVCERR << "TempWriteFile: Failed to rename temporary file " << m_temp << " to target " << m_target << endl;
Definition: Exceptions.h:69
void moveToTarget()
Rename the temporary file to the target filename.
Definition: TempWriteFile.cpp:53
QString getTemporaryFilename()
Return the name of the temporary file.
Definition: TempWriteFile.cpp:47
Generated by
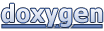