svcore
1.9
|
WavFileWriter.h
Go to the documentation of this file.
Definition: WavFileWriter.h:51
Definition: Selection.h:63
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
WavFileWriter(QString path, sv_samplerate_t sampleRate, int channels, FileWriteMode mode)
Definition: WavFileWriter.cpp:35
bool writeModel(DenseTimeValueModel *source, MultiSelection *selection=0)
Definition: WavFileWriter.cpp:114
A class that manages the creation of a temporary file with a given prefix and the renaming of that fi...
Definition: TempWriteFile.h:26
Base class for models containing dense two-dimensional data (value against time). ...
Definition: DenseTimeValueModel.h:30
Definition: WavFileWriter.h:34
bool putInterleavedFrames(const floatvec_t &frames)
As writeSamples, but compatible with WavFileReader api. More expensive.
Definition: WavFileWriter.cpp:204
bool writeSamples(const float *const *samples, sv_frame_t count)
Write samples from raw arrays; count is per-channel.
Definition: WavFileWriter.cpp:176
Generated by
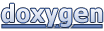