svcore
1.9
|
FileFeatureWriter.h
Go to the documentation of this file.
QString createOutputFilename(QString, TransformId)
Definition: FileFeatureWriter.cpp:170
map< TrackTransformPair, QString > FileNameMap
Definition: FileFeatureWriter.h:62
QFile * getOutputFile(QString, TransformId)
Definition: FileFeatureWriter.cpp:277
QTextStream * getOutputStream(QString, TransformId, QTextCodec *)
Definition: FileFeatureWriter.cpp:317
void testOutputFile(QString trackId, TransformId transformId) override
Throw FailedToOpenOutputStream if we can already tell that we will be unable to write to the output f...
Definition: FileFeatureWriter.cpp:231
map< QFile *, QTextStream * > FileStreamMap
Definition: FileFeatureWriter.h:64
void setParameters(map< string, string > ¶ms) override
Definition: FileFeatureWriter.cpp:123
TrackTransformPair getFilenameKey(QString, TransformId)
Definition: FileFeatureWriter.cpp:249
map< TrackTransformPair, QFile * > FileMap
Definition: FileFeatureWriter.h:63
virtual ~FileFeatureWriter()
Definition: FileFeatureWriter.cpp:54
Definition: FeatureWriter.h:37
pair< QString, TransformId > TrackTransformPair
Definition: FileFeatureWriter.h:61
Definition: FileFeatureWriter.h:38
QString getOutputFilename(QString, TransformId)
Definition: FileFeatureWriter.cpp:266
FileFeatureWriter(int support, QString extension)
Definition: FileFeatureWriter.cpp:33
ParameterList getSupportedParameters() const override
Definition: FileFeatureWriter.cpp:72
virtual void reviewFileForAppending(QString)
Definition: FileFeatureWriter.h:85
Generated by
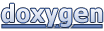