svcore
1.9
|
RDFExporter.cpp
Go to the documentation of this file.
virtual void setFixedEventTypeURI(QString uri)
Definition: RDFFeatureWriter.cpp:125
static RealTime frame2RealTime(sv_frame_t frame, sv_samplerate_t sampleRate)
Convert a sample frame at the given sample rate into a RealTime.
Definition: RealTimeSV.cpp:498
Definition: NoteModel.h:33
Definition: RDFFeatureWriter.h:41
EventVector getAllEvents() const
Definition: SparseTimeValueModel.h:158
virtual sv_samplerate_t getSampleRate() const =0
Return the frame rate in frames per second.
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
static QString getSupportedExtensions()
Return the file extensions that we can write, in a format suitable for use with QFileDialog.
Definition: RDFExporter.cpp:169
Definition: Transform.h:34
A model representing a wiggly-line plot with points at arbitrary intervals of the model resolution...
Definition: SparseTimeValueModel.h:34
void setParameters(map< string, string > ¶ms) override
Definition: RDFFeatureWriter.cpp:92
RegionModel – a model for intervals associated with a value, which we call regions for no very compe...
Definition: RegionModel.h:33
EventVector getAllEvents() const
Definition: SparseOneDimensionalModel.h:121
A model representing a series of time instants with optional labels but without values.
Definition: SparseOneDimensionalModel.h:37
void write(QString trackid, const Transform &transform, const Vamp::Plugin::OutputDescriptor &output, const Vamp::Plugin::FeatureList &features, std::string summaryType="") override
Definition: RDFFeatureWriter.cpp:131
Generated by
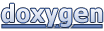