svcore
1.9
|
PropertyContainer.cpp
Go to the documentation of this file.
77 cerr << "WARNING: PropertyContainer[" << getPropertyContainerName() << "]::setProperty(" << name << "): no implementation in subclass!" << endl;
144 cerr << "PropertyContainer::convertPropertyStrings: Unable to match name string \"" << nameString << "\"" << endl;
202 SVDEBUG << "PropertyContainer::convertPropertyStrings: Invalid property name \"" << name << "\"" << endl;
214 cerr << "PropertyContainer::convertPropertyStrings: Unable to parse value string \"" << valueString << "\"" << endl;
217 SVDEBUG << "PropertyContainer::convertPropertyStrings: Property value \"" << i << "\" outside valid range " << min << " to " << max << endl;
void execute() override
Definition: PropertyContainer.cpp:236
int getUnitId(QString unit, bool registerNew=true)
Return the reference id for a given unit name.
Definition: UnitDatabase.cpp:52
virtual PropertyType getPropertyType(const PropertyName &) const
Return the type of the given property, or InvalidProperty if the property is not supported on this co...
Definition: PropertyContainer.cpp:29
virtual int getPositionForValue(double value) const =0
Return the position that maps to the given value, rounding to the nearest position and clamping to th...
virtual QString getPropertyGroupName(const PropertyName &) const
If this property has something in common with other properties on this container, return a name that ...
Definition: PropertyContainer.cpp:41
PropertyContainer * m_pc
Definition: PropertyContainer.h:177
void unexecute() override
Definition: PropertyContainer.cpp:243
virtual int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const
Return the minimum and maximum values for the given property and its current value in this container...
Definition: PropertyContainer.cpp:47
virtual bool convertPropertyStrings(QString nameString, QString valueString, PropertyName &name, int &value)
Definition: PropertyContainer.cpp:119
virtual QString getPropertyValueIconName(const PropertyName &, int value) const
If the given property is a ValueProperty, return the icon to be used for the given value for that pro...
Definition: PropertyContainer.cpp:63
virtual RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const
If the given property is a RangeProperty, return a new RangeMapper object mapping its integer range o...
Definition: PropertyContainer.cpp:69
virtual PropertyList getProperties() const
Get a list of the names of all the supported properties on this container.
Definition: PropertyContainer.cpp:23
virtual QString getPropertyContainerName() const =0
virtual void setPropertyFuzzy(QString nameString, QString valueString)
Set a property using a fuzzy match.
Definition: PropertyContainer.cpp:89
Definition: RangeMapper.h:24
QString getName() const override
Definition: PropertyContainer.cpp:249
virtual QString getPropertyLabel(const PropertyName &) const =0
Return the human-readable (and i18n'ised) name of a property.
virtual Command * getSetPropertyCommand(const PropertyName &, int value)
Obtain a command that sets the given property, which can be added to the command history for undo/red...
Definition: PropertyContainer.cpp:81
Definition: PropertyContainer.h:29
virtual QString getPropertyValueLabel(const PropertyName &, int value) const
If the given property is a ValueProperty, return the display label to be used for the given value for...
Definition: PropertyContainer.cpp:57
SetPropertyCommand(PropertyContainer *pc, const PropertyName &pn, int)
Definition: PropertyContainer.cpp:225
Definition: Command.h:26
virtual void setProperty(const PropertyName &, int value)
Set a property.
Definition: PropertyContainer.cpp:75
std::vector< PropertyName > PropertyList
Definition: PropertyContainer.h:37
virtual QString getPropertyIconName(const PropertyName &) const
Return an icon for the property, if any.
Definition: PropertyContainer.cpp:35
Generated by
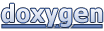