svcore
1.9
|
RangeMapper.h
Go to the documentation of this file.
QString getUnit() const override
Get the unit of the mapper's value range.
Definition: RangeMapper.h:140
Definition: RangeMapper.h:152
QString getUnit() const override
Get the unit of the mapper's value range.
Definition: RangeMapper.h:184
Definition: RangeMapper.h:195
virtual int getPositionForValue(double value) const =0
Return the position that maps to the given value, rounding to the nearest position and clamping to th...
QString getUnit() const override
Get the unit of the mapper's value range.
Definition: RangeMapper.h:257
Definition: RangeMapper.h:111
Definition: RangeMapper.h:78
Definition: RangeMapper.h:199
virtual double getValueForPositionUnclamped(int position) const =0
Return the value mapped from the given position, without clamping.
Definition: RangeMapper.h:200
Definition: RangeMapper.h:24
virtual double getValueForPosition(int position) const =0
Return the value mapped from the given position, clamping to the minimum and maximum extents of the m...
Definition: RangeMapper.h:201
virtual int getPositionForValueUnclamped(double value) const =0
Return the position that maps to the given value, rounding to the nearest position, without clamping.
virtual QString getLabel(int) const
The mapper may optionally provide special labels for one or more individual positions (such as the mi...
Definition: RangeMapper.h:74
virtual QString getUnit() const
Get the unit of the mapper's value range.
Definition: RangeMapper.h:64
QString getUnit() const override
Get the unit of the mapper's value range.
Definition: RangeMapper.h:98
Generated by
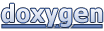