svcore
1.9
|
#include <RangeMapper.h>
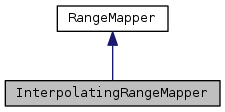
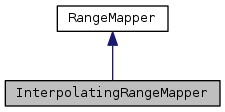
Public Types | |
typedef std::map< double, int > | CoordMap |
Public Member Functions | |
InterpolatingRangeMapper (CoordMap pointMappings, QString unit) | |
Given a series of (value, position) coordinate mappings, construct a range mapper that maps arbitrary values, in the range between minimum and maximum of the provided values, onto coordinates using linear interpolation between the supplied points. More... | |
int | getPositionForValue (double value) const override |
Return the position that maps to the given value, rounding to the nearest position and clamping to the minimum and maximum extents of the mapper's positional range. More... | |
int | getPositionForValueUnclamped (double value) const override |
Return the position that maps to the given value, rounding to the nearest position, without clamping. More... | |
double | getValueForPosition (int position) const override |
Return the value mapped from the given position, clamping to the minimum and maximum extents of the mapper's value range. More... | |
double | getValueForPositionUnclamped (int position) const override |
Return the value mapped from the given position, without clamping. More... | |
QString | getUnit () const override |
Get the unit of the mapper's value range. More... | |
virtual QString | getLabel (int) const |
The mapper may optionally provide special labels for one or more individual positions (such as the minimum position, the default, or indeed all positions). More... | |
Protected Member Functions | |
template<typename T > | |
double | interpolate (T *mapping, double v) const |
Protected Attributes | |
CoordMap | m_mappings |
std::map< int, double > | m_reverse |
QString | m_unit |
Detailed Description
Definition at line 152 of file RangeMapper.h.
Member Typedef Documentation
typedef std::map<double, int> InterpolatingRangeMapper::CoordMap |
Definition at line 155 of file RangeMapper.h.
Constructor & Destructor Documentation
InterpolatingRangeMapper::InterpolatingRangeMapper | ( | CoordMap | pointMappings, |
QString | unit | ||
) |
Given a series of (value, position) coordinate mappings, construct a range mapper that maps arbitrary values, in the range between minimum and maximum of the provided values, onto coordinates using linear interpolation between the supplied points.
!!! todo: Cubic – more generally useful than linear interpolation !!! todo: inverted flag
The set of provided mappings must contain at least two coordinates.
It is expected that the values and positions in the coordinate mappings will both be monotonically increasing (i.e. no inflections in the mapping curve). Behaviour is undefined if this is not the case.
Definition at line 174 of file RangeMapper.cpp.
References m_mappings, and m_reverse.
Member Function Documentation
|
overridevirtual |
Return the position that maps to the given value, rounding to the nearest position and clamping to the minimum and maximum extents of the mapper's positional range.
Implements RangeMapper.
Definition at line 186 of file RangeMapper.cpp.
References getPositionForValueUnclamped(), and m_mappings.
|
overridevirtual |
Return the position that maps to the given value, rounding to the nearest position, without clamping.
That is, whatever mapping function is in use will be projected even outside the minimum and maximum extents of the mapper's positional range. (The mapping outside that range is not guaranteed to be exact, except if the mapper is a linear one.)
Implements RangeMapper.
Definition at line 197 of file RangeMapper.cpp.
References interpolate(), and m_mappings.
Referenced by getPositionForValue().
|
overridevirtual |
Return the value mapped from the given position, clamping to the minimum and maximum extents of the mapper's value range.
Implements RangeMapper.
Definition at line 204 of file RangeMapper.cpp.
References getValueForPositionUnclamped(), and m_mappings.
|
overridevirtual |
Return the value mapped from the given position, without clamping.
That is, whatever mapping function is in use will be projected even outside the minimum and maximum extents of the mapper's value range. (The mapping outside that range is not guaranteed to be exact, except if the mapper is a linear one.)
Implements RangeMapper.
Definition at line 215 of file RangeMapper.cpp.
References interpolate(), and m_reverse.
Referenced by getValueForPosition().
|
inlineoverridevirtual |
Get the unit of the mapper's value range.
Reimplemented from RangeMapper.
Definition at line 184 of file RangeMapper.h.
|
protected |
Definition at line 222 of file RangeMapper.cpp.
Referenced by getPositionForValueUnclamped(), and getValueForPositionUnclamped().
|
inlinevirtualinherited |
The mapper may optionally provide special labels for one or more individual positions (such as the minimum position, the default, or indeed all positions).
These should be used in any display context in preference to just showing the numerical value for the position. If a position has such a label, return it here.
Reimplemented in LinearRangeMapper.
Definition at line 74 of file RangeMapper.h.
Referenced by LinearRangeMapper::getUnit().
Member Data Documentation
|
protected |
Definition at line 187 of file RangeMapper.h.
Referenced by getPositionForValue(), getPositionForValueUnclamped(), getValueForPosition(), and InterpolatingRangeMapper().
|
protected |
Definition at line 188 of file RangeMapper.h.
Referenced by getValueForPositionUnclamped(), and InterpolatingRangeMapper().
|
protected |
Definition at line 189 of file RangeMapper.h.
The documentation for this class was generated from the following files:
Generated by
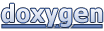