svcore
1.9
|
RangeMapper.cpp
Go to the documentation of this file.
80 // cerr << "getValueForPositionUnclamped(" << position << "): minval " << m_minval << ", maxval " << m_maxval << ", value " << value << endl;
double getValueForPositionUnclamped(int position) const override
Return the value mapped from the given position, without clamping.
Definition: RangeMapper.cpp:74
double getValueForPosition(int position) const override
Return the value mapped from the given position, clamping to the minimum and maximum extents of the m...
Definition: RangeMapper.cpp:346
Definition: RangeMapper.h:152
int getPositionForValue(double value) const override
Return the position that maps to the given value, rounding to the nearest position and clamping to th...
Definition: RangeMapper.cpp:186
int getPositionForValueUnclamped(double value) const override
Return the position that maps to the given value, rounding to the nearest position, without clamping.
Definition: RangeMapper.cpp:148
double getValueForPositionUnclamped(int position) const override
Return the value mapped from the given position, without clamping.
Definition: RangeMapper.cpp:358
virtual int getPositionForValue(double value) const =0
Return the position that maps to the given value, rounding to the nearest position and clamping to th...
MappingType chooseMappingTypeFor(const CoordMap &)
Definition: RangeMapper.cpp:282
QString getLabel(int position) const override
The mapper may optionally provide special labels for one or more individual positions (such as the mi...
Definition: RangeMapper.cpp:85
double interpolate(T *mapping, double v) const
Definition: RangeMapper.cpp:222
Definition: RangeMapper.h:111
Definition: RangeMapper.h:78
int getPositionForValue(double value) const override
Return the position that maps to the given value, rounding to the nearest position and clamping to th...
Definition: RangeMapper.cpp:139
static void convertRatioMinLog(double ratio, double minlog, int minpos, int maxpos, double &minval, double &maxval)
Definition: RangeMapper.cpp:130
int getPositionForValueUnclamped(double value) const override
Return the position that maps to the given value, rounding to the nearest position, without clamping.
Definition: RangeMapper.cpp:352
InterpolatingRangeMapper(CoordMap pointMappings, QString unit)
Given a series of (value, position) coordinate mappings, construct a range mapper that maps arbitrary...
Definition: RangeMapper.cpp:174
Definition: RangeMapper.h:199
virtual double getValueForPositionUnclamped(int position) const =0
Return the value mapped from the given position, without clamping.
double getValueForPosition(int position) const override
Return the value mapped from the given position, clamping to the minimum and maximum extents of the m...
Definition: RangeMapper.cpp:65
int getPositionForValueUnclamped(double value) const override
Return the position that maps to the given value, rounding to the nearest position, without clamping.
Definition: RangeMapper.cpp:197
Definition: RangeMapper.h:200
virtual double getValueForPosition(int position) const =0
Return the value mapped from the given position, clamping to the minimum and maximum extents of the m...
Definition: RangeMapper.h:201
virtual int getPositionForValueUnclamped(double value) const =0
Return the position that maps to the given value, rounding to the nearest position, without clamping.
int getPositionForValueUnclamped(double value) const override
Return the position that maps to the given value, rounding to the nearest position, without clamping.
Definition: RangeMapper.cpp:55
static void convertMinMax(int minpos, int maxpos, double minval, double maxval, double &minlog, double &ratio)
Definition: RangeMapper.cpp:119
LinearRangeMapper(int minpos, int maxpos, double minval, double maxval, QString unit="", bool inverted=false, std::map< int, QString > labels={})
Map values in range minval->maxval linearly into integer range minpos->maxpos.
Definition: RangeMapper.cpp:25
double getValueForPositionUnclamped(int position) const override
Return the value mapped from the given position, without clamping.
Definition: RangeMapper.cpp:167
double getValueForPosition(int position) const override
Return the value mapped from the given position, clamping to the minimum and maximum extents of the m...
Definition: RangeMapper.cpp:204
LogRangeMapper(int minpos, int maxpos, double minval, double maxval, QString m_unit="", bool inverted=false)
Map values in range minval->maxval into integer range minpos->maxpos such that logs of the values are...
Definition: RangeMapper.cpp:94
double getValueForPositionUnclamped(int position) const override
Return the value mapped from the given position, without clamping.
Definition: RangeMapper.cpp:215
AutoRangeMapper(CoordMap pointMappings, QString unit)
Given a series of (value, position) coordinate mappings, construct a range mapper that maps arbitrary...
Definition: RangeMapper.cpp:248
double getValueForPosition(int position) const override
Return the value mapped from the given position, clamping to the minimum and maximum extents of the m...
Definition: RangeMapper.cpp:158
int getPositionForValue(double value) const override
Return the position that maps to the given value, rounding to the nearest position and clamping to th...
Definition: RangeMapper.cpp:46
int getPositionForValue(double value) const override
Return the position that maps to the given value, rounding to the nearest position and clamping to th...
Definition: RangeMapper.cpp:340
Generated by
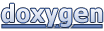