svcore
1.9
|
#include <RangeMapper.h>
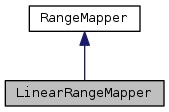
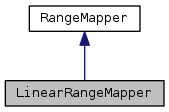
Public Member Functions | |
LinearRangeMapper (int minpos, int maxpos, double minval, double maxval, QString unit="", bool inverted=false, std::map< int, QString > labels={}) | |
Map values in range minval->maxval linearly into integer range minpos->maxpos. More... | |
int | getPositionForValue (double value) const override |
Return the position that maps to the given value, rounding to the nearest position and clamping to the minimum and maximum extents of the mapper's positional range. More... | |
int | getPositionForValueUnclamped (double value) const override |
Return the position that maps to the given value, rounding to the nearest position, without clamping. More... | |
double | getValueForPosition (int position) const override |
Return the value mapped from the given position, clamping to the minimum and maximum extents of the mapper's value range. More... | |
double | getValueForPositionUnclamped (int position) const override |
Return the value mapped from the given position, without clamping. More... | |
QString | getUnit () const override |
Get the unit of the mapper's value range. More... | |
QString | getLabel (int position) const override |
The mapper may optionally provide special labels for one or more individual positions (such as the minimum position, the default, or indeed all positions). More... | |
Protected Attributes | |
int | m_minpos |
int | m_maxpos |
double | m_minval |
double | m_maxval |
QString | m_unit |
bool | m_inverted |
std::map< int, QString > | m_labels |
Detailed Description
Definition at line 78 of file RangeMapper.h.
Constructor & Destructor Documentation
LinearRangeMapper::LinearRangeMapper | ( | int | minpos, |
int | maxpos, | ||
double | minval, | ||
double | maxval, | ||
QString | unit = "" , |
||
bool | inverted = false , |
||
std::map< int, QString > | labels = {} |
||
) |
Map values in range minval->maxval linearly into integer range minpos->maxpos.
minval and minpos must be less than maxval and maxpos respectively. If inverted is true, the range will be mapped "backwards" (minval to maxpos and maxval to minpos).
Definition at line 25 of file RangeMapper.cpp.
Member Function Documentation
|
overridevirtual |
Return the position that maps to the given value, rounding to the nearest position and clamping to the minimum and maximum extents of the mapper's positional range.
Implements RangeMapper.
Definition at line 46 of file RangeMapper.cpp.
References getPositionForValueUnclamped(), m_maxpos, and m_minpos.
Referenced by AutoRangeMapper::chooseMappingTypeFor().
|
overridevirtual |
Return the position that maps to the given value, rounding to the nearest position, without clamping.
That is, whatever mapping function is in use will be projected even outside the minimum and maximum extents of the mapper's positional range. (The mapping outside that range is not guaranteed to be exact, except if the mapper is a linear one.)
Implements RangeMapper.
Definition at line 55 of file RangeMapper.cpp.
References m_inverted, m_maxpos, m_maxval, m_minpos, and m_minval.
Referenced by getPositionForValue().
|
overridevirtual |
Return the value mapped from the given position, clamping to the minimum and maximum extents of the mapper's value range.
Implements RangeMapper.
Definition at line 65 of file RangeMapper.cpp.
References getValueForPositionUnclamped(), m_maxpos, and m_minpos.
|
overridevirtual |
Return the value mapped from the given position, without clamping.
That is, whatever mapping function is in use will be projected even outside the minimum and maximum extents of the mapper's value range. (The mapping outside that range is not guaranteed to be exact, except if the mapper is a linear one.)
Implements RangeMapper.
Definition at line 74 of file RangeMapper.cpp.
References m_inverted, m_maxpos, m_maxval, m_minpos, and m_minval.
Referenced by getValueForPosition().
|
inlineoverridevirtual |
Get the unit of the mapper's value range.
Reimplemented from RangeMapper.
Definition at line 98 of file RangeMapper.h.
References RangeMapper::getLabel().
|
overridevirtual |
The mapper may optionally provide special labels for one or more individual positions (such as the minimum position, the default, or indeed all positions).
These should be used in any display context in preference to just showing the numerical value for the position. If a position has such a label, return it here.
Reimplemented from RangeMapper.
Definition at line 85 of file RangeMapper.cpp.
References m_labels.
Member Data Documentation
|
protected |
Definition at line 102 of file RangeMapper.h.
Referenced by getPositionForValue(), getPositionForValueUnclamped(), getValueForPosition(), getValueForPositionUnclamped(), and LinearRangeMapper().
|
protected |
Definition at line 103 of file RangeMapper.h.
Referenced by getPositionForValue(), getPositionForValueUnclamped(), getValueForPosition(), getValueForPositionUnclamped(), and LinearRangeMapper().
|
protected |
Definition at line 104 of file RangeMapper.h.
Referenced by getPositionForValueUnclamped(), getValueForPositionUnclamped(), and LinearRangeMapper().
|
protected |
Definition at line 105 of file RangeMapper.h.
Referenced by getPositionForValueUnclamped(), getValueForPositionUnclamped(), and LinearRangeMapper().
|
protected |
Definition at line 106 of file RangeMapper.h.
|
protected |
Definition at line 107 of file RangeMapper.h.
Referenced by getPositionForValueUnclamped(), and getValueForPositionUnclamped().
|
protected |
Definition at line 108 of file RangeMapper.h.
Referenced by getLabel().
The documentation for this class was generated from the following files:
Generated by
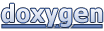