svcore
1.9
|
#include <PropertyContainer.h>
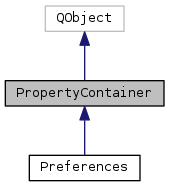
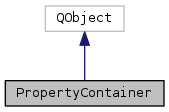
Classes | |
class | SetPropertyCommand |
Public Types | |
enum | PropertyType { ToggleProperty, RangeProperty, ValueProperty, ColourProperty, ColourMapProperty, UnitsProperty, InvalidProperty } |
typedef QString | PropertyName |
typedef std::vector< PropertyName > | PropertyList |
Public Slots | |
virtual void | setProperty (const PropertyName &, int value) |
Set a property. More... | |
virtual Command * | getSetPropertyCommand (const PropertyName &, int value) |
Obtain a command that sets the given property, which can be added to the command history for undo/redo. More... | |
virtual void | setPropertyFuzzy (QString nameString, QString valueString) |
Set a property using a fuzzy match. More... | |
virtual Command * | getSetPropertyCommand (QString nameString, QString valueString) |
As above, but returning a command. More... | |
Signals | |
void | propertyChanged (PropertyContainer::PropertyName) |
Public Member Functions | |
virtual | ~PropertyContainer () |
virtual PropertyList | getProperties () const |
Get a list of the names of all the supported properties on this container. More... | |
virtual QString | getPropertyLabel (const PropertyName &) const =0 |
Return the human-readable (and i18n'ised) name of a property. More... | |
virtual PropertyType | getPropertyType (const PropertyName &) const |
Return the type of the given property, or InvalidProperty if the property is not supported on this container. More... | |
virtual QString | getPropertyIconName (const PropertyName &) const |
Return an icon for the property, if any. More... | |
virtual QString | getPropertyGroupName (const PropertyName &) const |
If this property has something in common with other properties on this container, return a name that can be used to group them (in order to save screen space, for example). More... | |
virtual int | getPropertyRangeAndValue (const PropertyName &, int *min, int *max, int *deflt) const |
Return the minimum and maximum values for the given property and its current value in this container. More... | |
virtual QString | getPropertyValueLabel (const PropertyName &, int value) const |
If the given property is a ValueProperty, return the display label to be used for the given value for that property. More... | |
virtual QString | getPropertyValueIconName (const PropertyName &, int value) const |
If the given property is a ValueProperty, return the icon to be used for the given value for that property, if any. More... | |
virtual RangeMapper * | getNewPropertyRangeMapper (const PropertyName &) const |
If the given property is a RangeProperty, return a new RangeMapper object mapping its integer range onto an underlying floating point value range for human-intelligible display, if appropriate. More... | |
virtual QString | getPropertyContainerName () const =0 |
virtual QString | getPropertyContainerIconName () const =0 |
virtual std::shared_ptr< PlayParameters > | getPlayParameters () |
Return the play parameters for this layer, if any. More... | |
Protected Member Functions | |
virtual bool | convertPropertyStrings (QString nameString, QString valueString, PropertyName &name, int &value) |
Detailed Description
Definition at line 29 of file PropertyContainer.h.
Member Typedef Documentation
typedef QString PropertyContainer::PropertyName |
Definition at line 36 of file PropertyContainer.h.
typedef std::vector<PropertyName> PropertyContainer::PropertyList |
Definition at line 37 of file PropertyContainer.h.
Member Enumeration Documentation
Enumerator | |
---|---|
ToggleProperty | |
RangeProperty | |
ValueProperty | |
ColourProperty | |
ColourMapProperty | |
UnitsProperty | |
InvalidProperty |
Definition at line 39 of file PropertyContainer.h.
Constructor & Destructor Documentation
|
inlinevirtual |
Definition at line 34 of file PropertyContainer.h.
Member Function Documentation
|
virtual |
Get a list of the names of all the supported properties on this container.
These should be fixed (i.e. not internationalized).
Reimplemented in Preferences.
Definition at line 23 of file PropertyContainer.cpp.
Referenced by convertPropertyStrings().
|
pure virtual |
Return the human-readable (and i18n'ised) name of a property.
Implemented in Preferences.
Referenced by convertPropertyStrings().
|
virtual |
Return the type of the given property, or InvalidProperty if the property is not supported on this container.
Reimplemented in Preferences.
Definition at line 29 of file PropertyContainer.cpp.
References InvalidProperty.
Referenced by convertPropertyStrings().
|
virtual |
Return an icon for the property, if any.
Definition at line 35 of file PropertyContainer.cpp.
|
virtual |
If this property has something in common with other properties on this container, return a name that can be used to group them (in order to save screen space, for example).
e.g. "Window Type" and "Window Size" might both have a group name of "Window". If this property is not groupable, return the empty string.
Definition at line 41 of file PropertyContainer.cpp.
|
virtual |
Return the minimum and maximum values for the given property and its current value in this container.
Min and/or max may be passed as NULL if their values are not required.
Reimplemented in Preferences.
Definition at line 47 of file PropertyContainer.cpp.
Referenced by convertPropertyStrings(), PropertyContainer::SetPropertyCommand::execute(), and getSetPropertyCommand().
|
virtual |
If the given property is a ValueProperty, return the display label to be used for the given value for that property.
Reimplemented in Preferences.
Definition at line 57 of file PropertyContainer.cpp.
Referenced by convertPropertyStrings().
|
virtual |
If the given property is a ValueProperty, return the icon to be used for the given value for that property, if any.
Definition at line 63 of file PropertyContainer.cpp.
|
virtual |
If the given property is a RangeProperty, return a new RangeMapper object mapping its integer range onto an underlying floating point value range for human-intelligible display, if appropriate.
The RangeMapper should be allocated with new, and the caller takes responsibility for deleting it. Return NULL (as in the default implementation) if there is no such mapping.
Definition at line 69 of file PropertyContainer.cpp.
Referenced by convertPropertyStrings().
|
pure virtual |
Implemented in Preferences.
Referenced by setProperty().
|
pure virtual |
Implemented in Preferences.
|
inlinevirtual |
Return the play parameters for this layer, if any.
The return value is a shared_ptr that, if not null, can be passed to e.g. PlayParameterRepository::EditCommand to change the parameters.
Definition at line 121 of file PropertyContainer.h.
References getSetPropertyCommand(), propertyChanged(), setProperty(), and setPropertyFuzzy().
|
signal |
Referenced by getPlayParameters(), Preferences::setBackgroundMode(), Preferences::setFixedSampleRate(), Preferences::setNormaliseAudio(), Preferences::setOctaveOfMiddleC(), Preferences::setOmitTempsFromRecentFiles(), Preferences::setPropertyBoxLayout(), Preferences::setRecordMono(), Preferences::setResampleOnLoad(), Preferences::setRunPluginsInProcess(), Preferences::setShowHMS(), Preferences::setShowSplash(), Preferences::setSpectrogramSmoothing(), Preferences::setSpectrogramXSmoothing(), Preferences::setTemporaryDirectoryRoot(), Preferences::setTimeToTextMode(), Preferences::setTuningFrequency(), Preferences::setUseGaplessMode(), Preferences::setViewFontSize(), and Preferences::setWindowType().
|
virtualslot |
Set a property.
This is used for all property types. For boolean properties, zero is false and non-zero true; for colours, the integer value is an index into the colours in the global ColourDatabase.
Definition at line 75 of file PropertyContainer.cpp.
References getPropertyContainerName().
Referenced by PropertyContainer::SetPropertyCommand::execute(), getPlayParameters(), setPropertyFuzzy(), and PropertyContainer::SetPropertyCommand::unexecute().
|
virtualslot |
Obtain a command that sets the given property, which can be added to the command history for undo/redo.
Returns NULL if the property is already set to the given value.
Definition at line 81 of file PropertyContainer.cpp.
References getPropertyRangeAndValue().
Referenced by getPlayParameters(), and getSetPropertyCommand().
|
virtualslot |
Set a property using a fuzzy match.
Compare nameString with the property labels and underlying names, and if it matches one (with preference given to labels), try to convert valueString appropriately and set it. The valueString should contain a value label for value properties, a mapped value for range properties, "on" or "off" for toggle properties, a colour or unit name, or the underlying integer value for the property.
Note that as property and value labels may be translatable, the results of this function may vary by locale. It is intended for handling user-originated strings, not persistent storage.
The default implementation should work for most subclasses.
Definition at line 89 of file PropertyContainer.cpp.
References convertPropertyStrings(), and setProperty().
Referenced by getPlayParameters().
|
virtualslot |
As above, but returning a command.
Definition at line 104 of file PropertyContainer.cpp.
References convertPropertyStrings(), and getSetPropertyCommand().
|
protectedvirtual |
Definition at line 119 of file PropertyContainer.cpp.
References ColourMapProperty, ColourProperty, UnitDatabase::getInstance(), getNewPropertyRangeMapper(), RangeMapper::getPositionForValue(), getProperties(), getPropertyLabel(), getPropertyRangeAndValue(), getPropertyType(), getPropertyValueLabel(), UnitDatabase::getUnitId(), InvalidProperty, RangeProperty, SVDEBUG, ToggleProperty, UnitsProperty, and ValueProperty.
Referenced by getSetPropertyCommand(), and setPropertyFuzzy().
The documentation for this class was generated from the following files:
Generated by
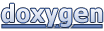