svcore
1.9
|
#include <PlayParameters.h>
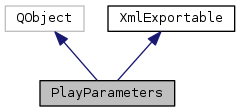
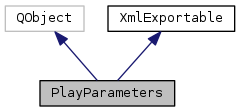
Public Types | |
enum | { NO_ID = -1 } |
typedef int | ExportId |
Public Slots | |
virtual void | setPlayMuted (bool muted) |
virtual void | setPlayAudible (bool nonMuted) |
virtual void | setPlayPan (float pan) |
virtual void | setPlayGain (float gain) |
virtual void | setPlayClipId (QString id) |
Signals | |
void | playParametersChanged () |
void | playMutedChanged (bool) |
void | playAudibleChanged (bool) |
void | playPanChanged (float) |
void | playGainChanged (float) |
void | playClipIdChanged (QString) |
Public Member Functions | |
PlayParameters () | |
virtual bool | isPlayMuted () const |
virtual bool | isPlayAudible () const |
virtual float | getPlayPan () const |
virtual float | getPlayGain () const |
virtual QString | getPlayClipId () const |
virtual void | copyFrom (const PlayParameters *) |
void | toXml (QTextStream &stream, QString indent="", QString extraAttributes="") const override |
Stream this exportable object out to XML on a text stream. More... | |
ExportId | getExportId () const |
Return the numerical export identifier for this object. More... | |
virtual QString | toXmlString (QString indent="", QString extraAttributes="") const |
Convert this exportable object to XML in a string. More... | |
Static Public Member Functions | |
static QString | encodeEntities (QString) |
static QString | encodeColour (int r, int g, int b) |
Protected Attributes | |
bool | m_playMuted |
float | m_playPan |
float | m_playGain |
QString | m_playClipId |
Private Member Functions | |
PlayParameters (const PlayParameters &) | |
PlayParameters & | operator= (const PlayParameters &) |
Detailed Description
Definition at line 23 of file PlayParameters.h.
Member Typedef Documentation
|
inherited |
Definition at line 33 of file XmlExportable.h.
Member Enumeration Documentation
|
inherited |
Enumerator | |
---|---|
NO_ID |
Definition at line 28 of file XmlExportable.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 28 of file PlayParameters.h.
|
private |
Member Function Documentation
|
inlinevirtual |
|
inlinevirtual |
Definition at line 31 of file PlayParameters.h.
References m_playMuted.
Referenced by PlayParameterRepository::EditCommand::getName().
|
inlinevirtual |
Definition at line 32 of file PlayParameters.h.
References m_playPan.
Referenced by copyFrom(), and PlayParameterRepository::EditCommand::getName().
|
inlinevirtual |
Definition at line 33 of file PlayParameters.h.
References m_playGain.
Referenced by copyFrom(), and PlayParameterRepository::EditCommand::getName().
|
inlinevirtual |
Definition at line 35 of file PlayParameters.h.
References copyFrom(), m_playClipId, playAudibleChanged(), playClipIdChanged(), playGainChanged(), playMutedChanged(), playPanChanged(), playParametersChanged(), setPlayAudible(), setPlayClipId(), setPlayGain(), setPlayMuted(), setPlayPan(), and toXml().
Referenced by copyFrom(), and PlayParameterRepository::EditCommand::getName().
|
virtual |
Definition at line 23 of file PlayParameters.cpp.
References getPlayClipId(), getPlayGain(), getPlayPan(), isPlayMuted(), m_playClipId, m_playGain, m_playMuted, m_playPan, playAudibleChanged(), playClipIdChanged(), playGainChanged(), playMutedChanged(), playPanChanged(), and playParametersChanged().
Referenced by PlayParameterRepository::EditCommand::EditCommand(), and getPlayClipId().
|
overridevirtual |
Stream this exportable object out to XML on a text stream.
Implements XmlExportable.
Definition at line 56 of file PlayParameters.cpp.
References m_playClipId, m_playGain, m_playMuted, and m_playPan.
Referenced by getPlayClipId().
|
virtualslot |
Definition at line 82 of file PlayParameters.cpp.
References m_playMuted, playAudibleChanged(), playMutedChanged(), and playParametersChanged().
Referenced by getPlayClipId(), setPlayAudible(), and PlayParameterRepository::EditCommand::setPlayMuted().
|
virtualslot |
Definition at line 94 of file PlayParameters.cpp.
References setPlayMuted().
Referenced by getPlayClipId(), and PlayParameterRepository::EditCommand::setPlayAudible().
|
virtualslot |
Definition at line 101 of file PlayParameters.cpp.
References m_playPan, playPanChanged(), and playParametersChanged().
Referenced by getPlayClipId(), and PlayParameterRepository::EditCommand::setPlayPan().
|
virtualslot |
Definition at line 111 of file PlayParameters.cpp.
References m_playGain, playGainChanged(), and playParametersChanged().
Referenced by getPlayClipId(), and PlayParameterRepository::EditCommand::setPlayGain().
|
virtualslot |
Definition at line 121 of file PlayParameters.cpp.
References m_playClipId, playClipIdChanged(), and playParametersChanged().
Referenced by getPlayClipId(), and PlayParameterRepository::EditCommand::setPlayClipId().
|
signal |
Referenced by copyFrom(), getPlayClipId(), setPlayClipId(), setPlayGain(), setPlayMuted(), and setPlayPan().
|
signal |
Referenced by copyFrom(), getPlayClipId(), and setPlayMuted().
|
signal |
Referenced by copyFrom(), getPlayClipId(), and setPlayMuted().
|
signal |
Referenced by copyFrom(), getPlayClipId(), and setPlayPan().
|
signal |
Referenced by copyFrom(), getPlayClipId(), and setPlayGain().
|
signal |
Referenced by copyFrom(), getPlayClipId(), and setPlayClipId().
|
private |
|
inherited |
Return the numerical export identifier for this object.
It's allocated the first time this is called, so objects on which this is never called do not get allocated one.
Definition at line 71 of file XmlExportable.cpp.
References XmlExportable::m_exportId, and mutex.
Referenced by EditableDenseThreeDimensionalModel::toXml(), BasicCompressedDenseThreeDimensionalModel::toXml(), EventSeries::toXml(), ImageModel::toXml(), TextModel::toXml(), Model::toXml(), SparseOneDimensionalModel::toXml(), RegionModel::toXml(), BoxModel::toXml(), SparseTimeValueModel::toXml(), NoteModel::toXml(), and XmlExportable::~XmlExportable().
|
virtualinherited |
Convert this exportable object to XML in a string.
The default implementation calls toXml and returns the result as a string. Do not override this unless you really know what you're doing.
Definition at line 25 of file XmlExportable.cpp.
References XmlExportable::toXml().
Referenced by ModelTransformerFactory::getConfigurationForTransform(), RDFTransformFactoryImpl::getTransforms(), and XmlExportable::~XmlExportable().
|
staticinherited |
Definition at line 41 of file XmlExportable.cpp.
Referenced by TextMatcher::test(), PluginXml::toXml(), ReadOnlyWaveFileModel::toXml(), Transform::toXml(), WritableWaveFileModel::toXml(), Model::toXml(), Event::toXml(), RegionModel::toXml(), BoxModel::toXml(), SparseTimeValueModel::toXml(), NoteModel::toXml(), and XmlExportable::~XmlExportable().
|
staticinherited |
Definition at line 54 of file XmlExportable.cpp.
Referenced by XmlExportable::~XmlExportable().
Member Data Documentation
|
protected |
Definition at line 59 of file PlayParameters.h.
Referenced by copyFrom(), isPlayAudible(), isPlayMuted(), setPlayMuted(), and toXml().
|
protected |
Definition at line 60 of file PlayParameters.h.
Referenced by copyFrom(), getPlayPan(), setPlayPan(), and toXml().
|
protected |
Definition at line 61 of file PlayParameters.h.
Referenced by copyFrom(), getPlayGain(), setPlayGain(), and toXml().
|
protected |
Definition at line 62 of file PlayParameters.h.
Referenced by copyFrom(), getPlayClipId(), setPlayClipId(), and toXml().
The documentation for this class was generated from the following files:
Generated by
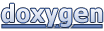