svcore
1.9
|
PlayParameters.cpp
Go to the documentation of this file.
void playPanChanged(float)
void playAudibleChanged(bool)
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: PlayParameters.cpp:56
virtual void setPlayAudible(bool nonMuted)
Definition: PlayParameters.cpp:94
void playParametersChanged()
void playMutedChanged(bool)
virtual void setPlayClipId(QString id)
Definition: PlayParameters.cpp:121
void playGainChanged(float)
void playClipIdChanged(QString)
Definition: PlayParameters.h:23
virtual void copyFrom(const PlayParameters *)
Definition: PlayParameters.cpp:23
Generated by
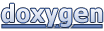