svcore
1.9
|
RecentFiles.cpp
Go to the documentation of this file.
void recentChanged()
std::vector< std::pair< QString, QString > > getRecentEntries() const
Return the list of recent identifiers, with labels.
Definition: RecentFiles.cpp:116
void add(QString identifier, QString label="")
Add a literal identifier, optionally with a label.
Definition: RecentFiles.cpp:131
void addFile(QString filepath, QString label="")
Add a name that is known to be either a file path or a URL, optionally with a label.
Definition: RecentFiles.cpp:163
bool getOmitTempsFromRecentFiles() const
Definition: Preferences.h:64
std::deque< std::pair< QString, QString > > m_entries
Definition: RecentFiles.h:118
std::vector< QString > getRecentIdentifiers() const
Return the list of recent identifiers, without labels.
Definition: RecentFiles.cpp:101
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
Definition: Preferences.h:23
RecentFiles(QString settingsGroup="RecentFiles", int maxCount=10)
Construct a RecentFiles object that saves and restores in the given QSettings group and truncates whe...
Definition: RecentFiles.cpp:25
Generated by
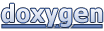